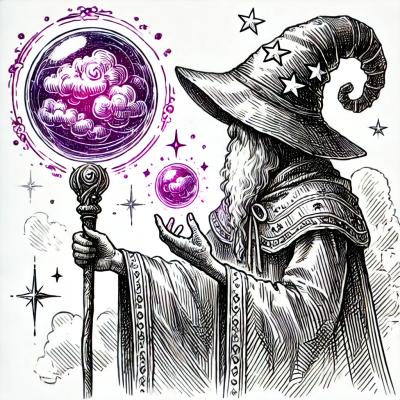
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
regexp-ast-analysis
Advanced tools
The 'regexp-ast-analysis' npm package provides tools for analyzing and manipulating the abstract syntax tree (AST) of regular expressions. It allows developers to parse regular expressions into an AST, traverse and modify the AST, and perform various analyses on the structure of the regular expression.
Parsing Regular Expressions
This feature allows you to parse a regular expression into its corresponding AST. The code sample demonstrates how to parse the regular expression '/abc/' and output its AST structure.
const { parseRegExpLiteral } = require('regexp-ast-analysis');
const ast = parseRegExpLiteral('/abc/');
console.log(JSON.stringify(ast, null, 2));
Traversing the AST
This feature allows you to traverse the AST of a regular expression. The code sample demonstrates how to visit each character node in the AST and log its raw value.
const { parseRegExpLiteral, visitRegExpAST } = require('regexp-ast-analysis');
const ast = parseRegExpLiteral('/abc/');
visitRegExpAST(ast, {
onCharacterEnter(node) {
console.log('Character:', node.raw);
}
});
Modifying the AST
This feature allows you to modify the AST of a regular expression. The code sample demonstrates how to change the character 'a' to 'x' in the AST.
const { parseRegExpLiteral, visitRegExpAST } = require('regexp-ast-analysis');
const ast = parseRegExpLiteral('/abc/');
visitRegExpAST(ast, {
onCharacterEnter(node) {
if (node.raw === 'a') {
node.raw = 'x';
}
}
});
console.log(JSON.stringify(ast, null, 2));
Analyzing the AST
This feature allows you to perform various analyses on the AST of a regular expression. The code sample demonstrates how to analyze the AST of the regular expression '/a|b/' and output the analysis results.
const { parseRegExpLiteral, analyzeRegExpAST } = require('regexp-ast-analysis');
const ast = parseRegExpLiteral('/a|b/');
const analysis = analyzeRegExpAST(ast);
console.log(analysis);
The 'regexpp' package is a regular expression parser and AST utility. It provides similar functionality to 'regexp-ast-analysis' in terms of parsing regular expressions into an AST and traversing the AST. However, 'regexpp' focuses more on providing a detailed and spec-compliant AST structure.
The 'regexp-tree' package offers a toolkit for working with regular expressions, including parsing, transforming, and optimizing them. It provides similar AST manipulation capabilities as 'regexp-ast-analysis' but also includes additional features like regular expression optimization and code generation.
The 'regex-parser' package is a simple utility for parsing regular expressions into an AST. While it provides basic parsing functionality similar to 'regexp-ast-analysis', it lacks the advanced analysis and traversal features found in 'regexp-ast-analysis'.
This is a library providing functionalities to analyse JavaScript RegExp.
All functions operate on AST nodes produced by regexpp. Characters are parsed by refa.
Install the library using npm:
npm i regexp-ast-analysis
Import the library:
import * as RAA from "regexp-ast-analysis";
Links to the full API documentation:
0.2.3 (2021-07-15)
FAQs
A library for analysing JS RegExp
The npm package regexp-ast-analysis receives a total of 393,158 weekly downloads. As such, regexp-ast-analysis popularity was classified as popular.
We found that regexp-ast-analysis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.