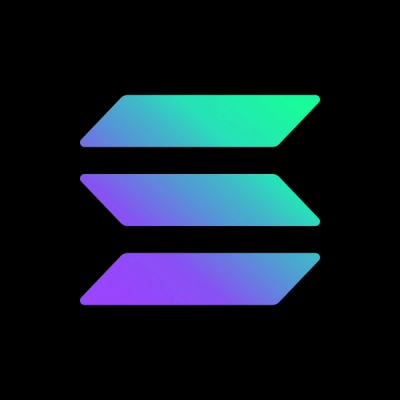
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
yarn add rejoiner2
or
npm install rejoiner2 --save
var Rejoiner2 = require('rejoiner2')
var apiClient = new Rejoiner2({
// Your seven character Rejoiner2 Site ID
siteId: 'eXaMpLe',
// Your account's 40 character API key
apiKey: 'tHiSaPiKeYiSjUsTaNeXaMpLeAnDyOuCaNtUsEiT',
})
The ping
endpoint can be used to verify your credentials are working.
apiClient.verify.ping()
.then(...)
.catch(...)
apiClient.customer.convert({
email: 'test@example.com',
cart_data: {
cart_value: 20000,
cart_item_count: 2,
promo: 'COUPON_CODE',
return_url: 'https://www.example.com/return_url',
...
},
cart_items: [
{
product_id: 'example',
name: 'Example Product',
price: 10000,
description: 'Information about Example Product.',
category: [
'Example Category 1',
'Example Category 2',
],
item_qty: 1,
qty_price: 10000,
product_url: 'https://www.example.com/products/example',
image_url: 'https://www.example.com/products/example/images/example.jpg',
...
},
{
product_id: 'example2',
name: 'Example Product 2',
price: 10000,
description: 'Information about Example Product 2.',
category: [
'Example Category 2',
'Example Category 3',
],
item_qty: 1,
qty_price: 10000,
product_url: 'https://www.example.com/products/example2',
image_url: 'https://www.example.com/products/example2/images/example.jpg',
...
},
...
],
})
.then(...)
.catch(...)
apiClient.customer.cancel('test@example.com')
.then(...)
.catch(...)
apiClient.customer.unsubscribe('test@example.com')
.then(...)
.catch(...)
apiClient.customer.optIn('test@example.com')
.then(...)
.catch(...)
apiClient.lists.get()
.then(...)
.catch(...)
apiClient.lists.contacts('eXaMpLeLiStId').get()
.then(...)
.catch(...)
apiClient.lists.contacts('eXaMpLeLiStId').get(2)
.then(...)
.catch(...)
apiClient.lists.contacts('eXaMpLeLiStId').add('test@example.com')
.then(...)
.catch(...)
apiClient.lists.contacts('eXaMpLeLiStId').remove('test@example.com')
.then(...)
.catch(...)
FAQs
Rejoiner2 REST API client wrapper for Node.js
We found that rejoiner2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.