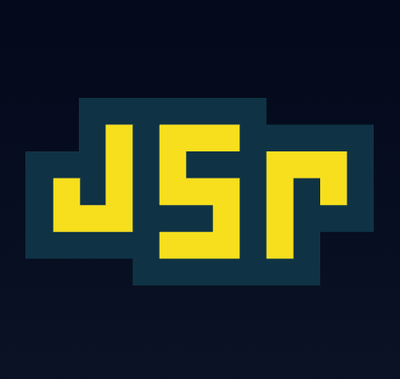
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
remark-stringify
Advanced tools
The remark-stringify package is a plugin for the remark ecosystem that allows you to serialize Markdown Abstract Syntax Trees (ASTs) into Markdown text. It is commonly used in conjunction with other remark plugins to process and transform Markdown content programmatically.
Basic Markdown Serialization
This feature allows you to convert a Markdown AST into a Markdown string. The example demonstrates how to serialize a simple AST representing a paragraph with the text 'Hello, world!'.
const remark = require('remark');
const stringify = require('remark-stringify');
const markdownAST = {
type: 'root',
children: [
{ type: 'paragraph', children: [{ type: 'text', value: 'Hello, world!' }] }
]
};
const markdownText = remark().use(stringify).stringify(markdownAST);
console.log(markdownText); // Outputs: 'Hello, world!\n'
Customizing Output
This feature allows you to customize the output format of the serialized Markdown. The example shows how to set options for bullet points and fenced code blocks.
const remark = require('remark');
const stringify = require('remark-stringify');
const markdownAST = {
type: 'root',
children: [
{ type: 'paragraph', children: [{ type: 'text', value: 'Hello, world!' }] }
]
};
const markdownText = remark()
.use(stringify, { bullet: '*', fences: true })
.stringify(markdownAST);
console.log(markdownText); // Outputs: 'Hello, world!\n'
Handling Complex ASTs
This feature demonstrates how to handle more complex ASTs, including headings, paragraphs, and lists. The example shows how to serialize an AST with multiple types of nodes.
const remark = require('remark');
const stringify = require('remark-stringify');
const markdownAST = {
type: 'root',
children: [
{ type: 'heading', depth: 1, children: [{ type: 'text', value: 'Title' }] },
{ type: 'paragraph', children: [{ type: 'text', value: 'This is a paragraph.' }] },
{ type: 'list', ordered: false, children: [
{ type: 'listItem', children: [{ type: 'paragraph', children: [{ type: 'text', value: 'Item 1' }] }] },
{ type: 'listItem', children: [{ type: 'paragraph', children: [{ type: 'text', value: 'Item 2' }] }] }
] }
]
};
const markdownText = remark().use(stringify).stringify(markdownAST);
console.log(markdownText); // Outputs: '# Title\n\nThis is a paragraph.\n\n* Item 1\n* Item 2\n'
Markdown-it is a fast and flexible Markdown parser that can also be used to render Markdown from an AST. It offers a wide range of plugins and customization options, making it a versatile alternative to remark-stringify.
Marked is a low-level Markdown compiler that allows for extensive customization. It is known for its speed and flexibility, making it a good choice for projects that require fine-grained control over Markdown rendering.
Showdown is a bidirectional Markdown to HTML converter that can also be used to serialize Markdown from an AST. It is easy to use and offers a range of extensions for additional functionality.
Compiler for unified. Stringifies an MDAST syntax tree to markdown. Used in the remark processor. Can be extended to change how markdown is compiled.
npm:
npm install remark-stringify
var unified = require('unified');
var createStream = require('unified-stream');
var parse = require('remark-parse');
var toc = require('remark-toc');
var stringify = require('remark-stringify');
var processor = unified()
.use(parse)
.use(toc)
.use(stringify, {
bullet: '*',
fence: '~',
fences: true,
incrementListMarker: false
});
process.stdin
.pipe(createStream(processor))
.pipe(process.stdout);
processor.use(stringify[, options])
Configure the processor
to stringify MDAST syntax trees
to markdown.
options
Options are passed directly, or passed later through processor.data()
.
options.gfm
Stringify with the required escapes for GFM compatible markdown (boolean
,
default: true
).
|
, for tables):
, for literal URLs)~
, for strike-through)options.commonmark
Stringify for CommonMark compatible markdown (boolean
, default: false
).
options.pedantic
Stringify for pedantic compatible markdown (boolean
, default: false
).
options.entities
How to stringify entities (string
or boolean
, default: false
):
true
— Entities are generated for special HTML characters
(&
> &
) and non-ASCII characters (©
> ©
).
If named entities are not (widely) supported, numbered character
references are used (’
> ’
)'numbers'
— Numbered entities are generated (&
> &
)
for special HTML characters and non-ASCII characters'escape'
— Special HTML characters are encoded (&
>
&
, ’
> ’
), non-ASCII characters not (ö persists)options.setext
Compile headings, when possible, in Setext-style (boolean
, default: false
).
Uses =
for level one headings and -
for level two headings. Other heading
levels are compiled as ATX (respecting closeAtx
).
options.closeAtx
Compile ATX headings with the same amount of closing hashes as opening hashes
(boolean
, default: false
).
options.looseTable
Create tables without fences: initial and final pipes (boolean
, default:
false
).
options.spacedTable
Create tables without spacing between pipes and content (boolean
, default:
true
).
options.paddedTable
Create tables with padding in each cell so that they are the same size
(boolean
, default: true
).
options.stringLength
Function passed to markdown-table
to detect the length of a
table cell (Function
, default: s => s.length
).
options.fence
Fence marker to use for code blocks ('~'
or '`'
, default: '`'
).
options.fences
Stringify code blocks without language with fences (boolean
, default:
false
).
options.bullet
Bullet marker to use for unordered list items ('-'
, '*'
, or '+'
,
default: '-'
).
options.listItemIndent
How to indent the content from list items ('tab'
, 'mixed'
or '1'
,
default: 'tab'
).
'tab'
: use tab stops (4 spaces)'1'
: use one space'mixed'
: use 1
for tight and tab
for loose list itemsoptions.incrementListMarker
Whether to increment ordered list item bullets (boolean
, default: true
).
options.rule
Marker to use for thematic breaks / horizontal rules ('-'
, '*'
, or '_'
,
default: '*'
).
options.ruleRepetition
Number of markers to use for thematic breaks / horizontal rules (number
,
default: 3
). Should be 3
or more.
options.ruleSpaces
Whether to pad thematic break (horizontal rule) markers with spaces (boolean
,
default true
).
options.strong
Marker to use for importance ('_'
or '*'
, default '*'
).
options.emphasis
Marker to use for emphasis ('_'
or '*'
, default '_'
).
stringify.Compiler
Access to the raw compiler, if you need it.
If this plug-in is used, it adds a Compiler
constructor
to the processor
. Other plug-ins can change and add visitors on
the compiler’s prototype to change how markdown is stringified.
The below plug-in modifies a visitor to add an extra blank line before level two headings.
module.exports = gap;
function gap() {
var Compiler = this.Compiler;
var visitors = Compiler.prototype.visitors;
var heading = visitors.heading;
visitors.heading = heading;
function heading(node) {
return (node.depth === 2 ? '\n' : '') + heading.apply(this, arguments);
}
}
Compiler#visitors
An object mapping node types to visitor
s.
function visitor(node[, parent])
Stringify node
.
string
, the compiled given node
.
FAQs
remark plugin to add support for serializing markdown
The npm package remark-stringify receives a total of 2,762,902 weekly downloads. As such, remark-stringify popularity was classified as popular.
We found that remark-stringify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.