remote-pay-cloud
Advanced tools
Comparing version 3.2.1-5 to 3.2.2
@@ -24,4 +24,2 @@ "use strict"; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.CAPTURE_PREAUTH] = remote_pay_cloud_api_1.remotemessage.CapturePreAuthMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.INCREMENT_PREAUTH_RESPONSE] = remote_pay_cloud_api_1.remotemessage.IncrementPreAuthResponseMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.INCREMENT_PREAUTH_REQUEST] = remote_pay_cloud_api_1.remotemessage.IncrementPreAuthMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.CONFIRM_PAYMENT_MESSAGE] = remote_pay_cloud_api_1.remotemessage.ConfirmPaymentMessage; | ||
@@ -105,5 +103,2 @@ MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.LAST_MSG_REQUEST] = remote_pay_cloud_api_1.remotemessage.LastMessageRequestMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.SHOW_RECEIPT_OPTIONS_RESPONSE] = remote_pay_cloud_api_1.remotemessage.ShowReceiptOptionsResponseMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.BALANCE_INQUIRY_RESPONSE] = remote_pay_cloud_api_1.remotemessage.BalanceInquiryResponseMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.REQUEST_SIGNATURE_RESPONSE] = remote_pay_cloud_api_1.remotemessage.SignatureResponseMessage; | ||
MethodToMessage.methodToType[remote_pay_cloud_api_1.remotemessage.Method.REQUEST_TIP_RESPONSE] = remote_pay_cloud_api_1.remotemessage.RequestTipResponseMessage; | ||
}; | ||
@@ -110,0 +105,0 @@ MethodToMessage.methodToType = null; |
@@ -191,2 +191,3 @@ "use strict"; | ||
listener.onDeviceDisconnected(); // changed from onDisconnected in 1.3 | ||
listener.onDisconnected(); // left here for backwards compatibility. Deprecated in 1.3* | ||
} | ||
@@ -204,2 +205,3 @@ catch (e) { | ||
listener.onDeviceReady(merchantInfo); // changed from onReady in 1.3 | ||
listener.onReady(merchantInfo); // left here for backwards compatibility. Deprecated in 1.3* | ||
} | ||
@@ -259,14 +261,2 @@ catch (e) { | ||
}; | ||
CloverConnectorBroadcaster.prototype.notifyOnIncrementPreAuthResponse = function (response) { | ||
var _this = this; | ||
this.logger.debug('Sending IncrementPreAuth notification to listeners'); | ||
this.listeners.forEach(function (listener) { | ||
try { | ||
listener.onIncrementPreAuthResponse(response); | ||
} | ||
catch (e) { | ||
_this.logger.error(e); | ||
} | ||
}); | ||
}; | ||
CloverConnectorBroadcaster.prototype.notifyOnDeviceError = function (errorEvent) { | ||
@@ -509,35 +499,2 @@ var _this = this; | ||
}; | ||
CloverConnectorBroadcaster.prototype.notifyOnSignatureCollected = function (response) { | ||
var _this = this; | ||
this.listeners.forEach(function (listener) { | ||
try { | ||
listener.onRequestSignatureResponse(response); | ||
} | ||
catch (e) { | ||
_this.logger.error(e); | ||
} | ||
}); | ||
}; | ||
CloverConnectorBroadcaster.prototype.notifyOnCheckBalanceResponse = function (response) { | ||
var _this = this; | ||
this.listeners.forEach(function (listener) { | ||
try { | ||
listener.onCheckBalanceResponse(response); | ||
} | ||
catch (e) { | ||
_this.logger.error(e); | ||
} | ||
}); | ||
}; | ||
CloverConnectorBroadcaster.prototype.notifyOnTipResponse = function (response) { | ||
var _this = this; | ||
this.listeners.forEach(function (listener) { | ||
try { | ||
listener.onRequestTipResponse(response); | ||
} | ||
catch (e) { | ||
_this.logger.error(e); | ||
} | ||
}); | ||
}; | ||
return CloverConnectorBroadcaster; | ||
@@ -544,0 +501,0 @@ }()); |
@@ -132,3 +132,3 @@ "use strict"; | ||
else { | ||
var devicesEndpoint = Endpoints_1.Endpoints.getDevicesEndpoint(configuration.domain, configuration.merchantId, configuration.oauthToken); | ||
var devicesEndpoint = Endpoints_1.Endpoints.getDevicesEndpoint(configuration.domain, configuration.merchantId); | ||
this.httpSupport.getData(devicesEndpoint, function (devices) { | ||
@@ -142,3 +142,3 @@ this.handleDeviceResult(LegacyCloverConnector.buildMapOfSerialToDevice(devices), configuration); | ||
this.broadcaster.notifyOnDeviceError(errorResponse); | ||
}.bind(this)); | ||
}.bind(this), { "Authorization": "Bearer " + configuration.oauthToken }); | ||
} | ||
@@ -145,0 +145,0 @@ } |
@@ -22,3 +22,3 @@ "use strict"; | ||
}; | ||
CloverWebSocketClient.prototype.connect = function () { | ||
CloverWebSocketClient.prototype.connect = function (accessToken) { | ||
if (this.socket != null) { | ||
@@ -32,3 +32,3 @@ throw new Error("Socket already created. Must create a new CloverWebSocketClient"); | ||
this.socket.addListener(this); | ||
this.socket.connect(); | ||
this.socket.connect(accessToken); | ||
} | ||
@@ -35,0 +35,0 @@ catch (e) { |
@@ -66,5 +66,5 @@ "use strict"; | ||
// See http://jonnyreeves.co.uk/2013/making-xhr-request-to-https-domains-with-winjs/ for more information. | ||
this.httpSupport.getData(Endpoints_1.Endpoints.getMerchantEndpoint(this.cloverServer, this.merchantId, this.accessToken), function (data) { return _this.obtainWebSocketUrlAndSendPushAlert(); }, function (error) { | ||
this.httpSupport.getData(Endpoints_1.Endpoints.getDevicesEndpoint(this.cloverServer, this.merchantId), function (data) { return _this.obtainWebSocketUrlAndSendPushAlert(); }, function (error) { | ||
_this.logger.warn("IE 11 - Initial GET failed.", error); | ||
}); | ||
}, this.buildAuthorizationHeader(this.accessToken)); | ||
} | ||
@@ -93,7 +93,7 @@ else { | ||
// already running, but this is not harmful. | ||
var alertEndpoint = Endpoints_1.Endpoints.getAlertDeviceEndpoint(this.cloverServer, this.merchantId, this.accessToken); | ||
var alertEndpoint = Endpoints_1.Endpoints.getAlertDeviceEndpoint(this.cloverServer, this.merchantId); | ||
var deviceContactInfo = new DeviceContactInfo_1.DeviceContactInfo(this.deviceId.replace(/-/g, ""), true); | ||
this.httpSupport.postData(alertEndpoint, function (data) { return _this.deviceNotificationSent(data); }, function (error) { | ||
_this.connectionError(_this.cloverWebSocketClient, "Error sending alert to device. Details: " + error.message); | ||
}, deviceContactInfo); | ||
}, deviceContactInfo, this.buildAuthorizationHeader(this.accessToken)); | ||
}; | ||
@@ -114,3 +114,3 @@ /** | ||
if (!notificationResponse.hasOwnProperty('sent') || notificationResponse.sent) { | ||
var deviceWebSocketEndpoint = Endpoints_1.Endpoints.getDeviceWebSocketEndpoint(notificationResponse, this.friendlyId, this.forceConnect, this.merchantId, this.accessToken); | ||
var deviceWebSocketEndpoint = Endpoints_1.Endpoints.getDeviceWebSocketEndpoint(notificationResponse, this.friendlyId, this.forceConnect, this.merchantId); | ||
this.doOptionsCallToAvoid401Error(deviceWebSocketEndpoint); | ||
@@ -143,3 +143,3 @@ } | ||
} | ||
this.httpSupport.options(httpUrl, function (data, xmlHttpReqImpl) { return _this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl); }, function (data, xmlHttpReqImpl) { return _this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl); }); | ||
this.httpSupport.options(httpUrl, function (data, xmlHttpReqImpl) { return _this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl); }, function (data, xmlHttpReqImpl) { return _this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl); }, this.buildAuthorizationHeader(this.accessToken)); | ||
}; | ||
@@ -183,4 +183,7 @@ /** | ||
} | ||
_super.prototype.initializeWithUri.call(this, deviceWebSocketEndpoint); | ||
_super.prototype.initializeWithUri.call(this, deviceWebSocketEndpoint, this.accessToken); | ||
}; | ||
WebSocketCloudCloverTransport.prototype.buildAuthorizationHeader = function (token) { | ||
return { "Authorization": "Bearer " + token }; | ||
}; | ||
/** | ||
@@ -187,0 +190,0 @@ * |
@@ -120,4 +120,5 @@ "use strict"; | ||
* @param deviceEndpoint | ||
* @param accessToken | ||
*/ | ||
WebSocketCloverTransport.prototype.initializeWithUri = function (deviceEndpoint) { | ||
WebSocketCloverTransport.prototype.initializeWithUri = function (deviceEndpoint, accessToken) { | ||
if (this.cloverWebSocketClient != null) { | ||
@@ -132,3 +133,3 @@ if (this.cloverWebSocketClient.isOpen() || this.cloverWebSocketClient.isConnecting()) { | ||
this.cloverWebSocketClient = new CloverWebSocketClient_1.CloverWebSocketClient(deviceEndpoint, this, this.webSocketImplClass); | ||
this.cloverWebSocketClient.connect(); | ||
this.cloverWebSocketClient.connect(accessToken); | ||
this.logger.info('Connection attempt complete.'); | ||
@@ -135,0 +136,0 @@ this.notifyConnectionAttemptComplete(); |
@@ -41,10 +41,9 @@ "use strict"; | ||
* | ||
* @param {any} - notificationResponse - The notification response from COS. | ||
* @param {any} notificationResponse - The notification response from COS. | ||
* @param {string} friendlyId - an id used to identify the POS. | ||
* @param {boolean} forceConnect - if true, then the attempt will overtake any existing connection | ||
* @param {boolean} merchantId - unique identifier for the merchant. | ||
* @param {boolean} accessToken - mid access token | ||
* @returns {string} The endpoint used to connect to a websocket on the server that will proxy to a device | ||
*/ | ||
Endpoints.getDeviceWebSocketEndpoint = function (notificationResponse, friendlyId, forceConnect, merchantId, accessToken) { | ||
Endpoints.getDeviceWebSocketEndpoint = function (notificationResponse, friendlyId, forceConnect, merchantId) { | ||
var variables = {}; | ||
@@ -55,6 +54,8 @@ variables[Endpoints.WEBSOCKET_TOKEN_KEY] = notificationResponse.token; | ||
variables[Endpoints.WEBSOCKET_FORCE_CONNECT_KEY] = forceConnect; | ||
variables[Endpoints.WEBSOCKET_MERCHANT_ID_KEY] = merchantId; | ||
var deviceWebSocketEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.WEBSOCKET_PATH + | ||
Endpoints.WEBSOCKET_TOKEN_SUFFIX + | ||
Endpoints.WEBSOCKET_FRIENDLY_ID_SUFFIX + | ||
Endpoints.WEBSOCKET_FORCE_CONNECT_SUFFIX; | ||
Endpoints.WEBSOCKET_FORCE_CONNECT_SUFFIX + | ||
Endpoints.WEBSOCKET_MERCHANT_ID_SUFFIX; | ||
if (notificationResponse.serverIdentifier) { | ||
@@ -70,11 +71,9 @@ deviceWebSocketEndpointPath += "&" + Endpoints.CLOUD_SERVER_IDENTIFIER_KEY + "=" + notificationResponse.serverIdentifier; | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* @returns {string} endpoint - the url to use to retrieve the merchant | ||
*/ | ||
Endpoints.getMerchantEndpoint = function (domain, merchantId, accessToken) { | ||
Endpoints.getMerchantEndpoint = function (domain, merchantId) { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
var merchantEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.MERCHANT_V3_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
var merchantEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.MERCHANT_V3_PATH; | ||
return Endpoints.setVariables(merchantEndpointPath, variables); | ||
@@ -87,12 +86,10 @@ }; | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* | ||
* @returns {string} | ||
*/ | ||
Endpoints.getDevicesEndpoint = function (domain, merchantId, accessToken) { | ||
Endpoints.getDevicesEndpoint = function (domain, merchantId) { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
var devicesEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.DEVICE_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
var devicesEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.DEVICE_PATH; | ||
return Endpoints.setVariables(devicesEndpointPath, variables); | ||
@@ -107,8 +104,7 @@ }; | ||
*/ | ||
Endpoints.getAlertDeviceEndpoint = function (domain, merchantId, accessToken) { | ||
Endpoints.getAlertDeviceEndpoint = function (domain, merchantId) { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
var alertDeviceEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.REMOTE_PAY_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
var alertDeviceEndpointPath = Endpoints.DOMAIN_PATH + Endpoints.REMOTE_PAY_PATH; | ||
return Endpoints.setVariables(alertDeviceEndpointPath, variables); | ||
@@ -167,4 +163,2 @@ }; | ||
}; | ||
Endpoints.ACCESS_TOKEN_KEY = "axsTkn"; | ||
Endpoints.ACCESS_TOKEN_SUFFIX = "?access_token={" + Endpoints.ACCESS_TOKEN_KEY + "}"; | ||
// The key of the server identifier that COS will return in the cloud server URL. | ||
@@ -202,2 +196,4 @@ // See WebSocketCloudCloverTransport.initialize | ||
Endpoints.WEBSOCKET_FORCE_CONNECT_SUFFIX = "&forceConnect={" + Endpoints.WEBSOCKET_FORCE_CONNECT_KEY + "}"; | ||
Endpoints.WEBSOCKET_MERCHANT_ID_KEY = "mid"; | ||
Endpoints.WEBSOCKET_MERCHANT_ID_SUFFIX = "&wsMid={" + Endpoints.WEBSOCKET_MERCHANT_ID_KEY + "}"; | ||
Endpoints.OAUTH_PATH = "oauth/authorize?response_type=token"; | ||
@@ -204,0 +200,0 @@ Endpoints.OAUTH_CLIENT_ID_KEY = "client_id"; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
var Logger_1 = require("../remote/client/util/Logger"); | ||
var addHeaders_1 = require("./addHeaders"); | ||
/** | ||
@@ -52,3 +53,3 @@ * Interface used to abstract implementation details to allow for NodeJS and | ||
*/ | ||
HttpSupport.prototype.doXmlHttp = function (method, endpoint, onDataLoaded, onError) { | ||
HttpSupport.prototype.doXmlHttp = function (method, endpoint, onDataLoaded, onError, additionalHeaders) { | ||
var xmlHttp = new this.xmlHttpImplClass(); | ||
@@ -62,2 +63,3 @@ this.setXmlHttpCallback(xmlHttp, endpoint, onDataLoaded, onError); | ||
} | ||
addHeaders_1.addHeaders(additionalHeaders, xmlHttp); | ||
xmlHttp.send(); | ||
@@ -69,9 +71,3 @@ }; | ||
xmlHttp.open(method, endpoint, true); | ||
if (additionalHeaders) { | ||
for (var key in additionalHeaders) { | ||
if (additionalHeaders.hasOwnProperty(key)) { | ||
xmlHttp.setRequestHeader(key, additionalHeaders[key]); | ||
} | ||
} | ||
} | ||
addHeaders_1.addHeaders(additionalHeaders, xmlHttp); | ||
if (sendData) { | ||
@@ -95,4 +91,4 @@ xmlHttp.setRequestHeader("Content-Type", "application/json;charset=UTF-8"); | ||
*/ | ||
HttpSupport.prototype.getData = function (endpoint, onDataLoaded, onError) { | ||
this.doXmlHttp("GET", endpoint, onDataLoaded, onError); | ||
HttpSupport.prototype.getData = function (endpoint, onDataLoaded, onError, additionalHeaders) { | ||
this.doXmlHttp("GET", endpoint, onDataLoaded, onError, additionalHeaders); | ||
}; | ||
@@ -102,4 +98,4 @@ /** | ||
*/ | ||
HttpSupport.prototype.options = function (endpoint, onDataLoaded, onError) { | ||
this.doXmlHttp("OPTIONS", endpoint, onDataLoaded, onError); | ||
HttpSupport.prototype.options = function (endpoint, onDataLoaded, onError, additionalHeaders) { | ||
this.doXmlHttp("OPTIONS", endpoint, onDataLoaded, onError, additionalHeaders); | ||
}; | ||
@@ -106,0 +102,0 @@ /** |
@@ -12,3 +12,3 @@ "use strict"; | ||
*/ | ||
Version.CLOVER_CLOUD_SDK_VERSION = "4.0.0"; | ||
Version.CLOVER_CLOUD_SDK_VERSION = "3.2.2"; | ||
/** | ||
@@ -15,0 +15,0 @@ * @type {string} - The current SDK name. |
@@ -31,6 +31,8 @@ "use strict"; | ||
* @param endpoint - the url that will connected to | ||
* @param accessToken - Here the access token is passed as a second param to `new WebSocket()` and will be read | ||
* by the support server as a "subprotocol" in the Sec-WebSocket-Protocol header value. | ||
* @returns {WebSocket} - the specific implementation of a websocket | ||
*/ | ||
BrowserWebSocketImpl.prototype.createWebSocket = function (endpoint) { | ||
return new WebSocket(endpoint); | ||
BrowserWebSocketImpl.prototype.createWebSocket = function (endpoint, accessToken) { | ||
return new WebSocket(endpoint, accessToken); | ||
}; | ||
@@ -37,0 +39,0 @@ /** |
@@ -24,5 +24,5 @@ "use strict"; | ||
} | ||
CloverWebSocketInterface.prototype.connect = function () { | ||
CloverWebSocketInterface.prototype.connect = function (accessToken) { | ||
var _this = this; | ||
this.webSocket = this.createWebSocket(this.endpoint); | ||
this.webSocket = this.createWebSocket(this.endpoint, accessToken); | ||
if (typeof this.webSocket["addEventListener"] !== "function") { | ||
@@ -29,0 +29,0 @@ this.logger.error("FATAL: The websocket implementation being used must have an 'addEventListener' function. Either use a supported websocket implementation (https://www.npmjs.com/package/ws) or override the connect method on CloverWebSocketInterface."); |
@@ -60,3 +60,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
capturePreAuth(request: sdk.remotepay.CapturePreAuthRequest): void; | ||
incrementPreAuth(request: sdk.remotepay.IncrementPreAuthRequest): void; | ||
tipAdjustAuth(request: sdk.remotepay.TipAdjustAuthRequest): void; | ||
@@ -99,5 +98,2 @@ vaultCard(cardEntryMethods: number): void; | ||
startCustomActivity(request: sdk.remotepay.CustomActivityRequest): void; | ||
checkBalance(request: sdk.remotepay.CheckBalanceRequest): void; | ||
requestSignature(request: sdk.remotepay.SignatureRequest): void; | ||
requestTip(request: sdk.remotepay.TipRequest): void; | ||
static populateBaseResponse(response: sdk.remotepay.BaseResponse, success: boolean, responseCode: sdk.remotepay.ResponseCode, reason?: string, message?: string): void; | ||
@@ -153,4 +149,2 @@ static populatePaymentResponse(response: sdk.remotepay.PaymentResponse, success: boolean, responseCode: sdk.remotepay.ResponseCode, payment: sdk.payments.Payment, signature?: sdk.base.Signature, reason?: string, message?: string): void; | ||
onCapturePreAuth(capturePreAuthResponseMessage: sdk.remotemessage.CapturePreAuthResponseMessage): void; | ||
onIncrementPreAuthError(result: sdk.remotepay.ResponseCode, reason: string, message: string): void; | ||
onIncrementPreAuthResponse(responseMsg: sdk.remotemessage.IncrementPreAuthResponseMessage): void; | ||
onCloseoutResponse(status: sdk.remotemessage.ResultStatus, reason: string, batch: sdk.payments.Batch): void; | ||
@@ -181,6 +175,13 @@ onDeviceDisconnected(device: CloverDevice, message?: string): void; | ||
onDisplayReceiptOptionsResponse(status: sdk.remotemessage.ResultStatus, reason: string): void; | ||
onSignatureCollected(signatureResponseMessage: sdk.remotemessage.SignatureResponseMessage): void; | ||
onBalanceInquiryResponse(balanceInquiryResponseMessage: sdk.remotemessage.BalanceInquiryResponseMessage): void; | ||
onRequestTipResponse(requestTipResponseMessage: sdk.remotemessage.RequestTipResponseMessage): void; | ||
} | ||
namespace InnerDeviceObserver { | ||
class SVR extends sdk.remotepay.VerifySignatureRequest { | ||
cloverDevice: CloverDevice; | ||
constructor(device: CloverDevice); | ||
accept(): void; | ||
reject(): void; | ||
setSignature(signature: sdk.base.Signature): void; | ||
setPayment(payment: sdk.payments.Payment): void; | ||
} | ||
} | ||
} |
@@ -32,3 +32,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
notifyOnCapturePreAuth(response: sdk.remotepay.CapturePreAuthResponse): void; | ||
notifyOnIncrementPreAuthResponse(response: sdk.remotepay.IncrementPreAuthResponse): void; | ||
notifyOnDeviceError(errorEvent: sdk.remotepay.CloverDeviceErrorEvent): void; | ||
@@ -54,5 +53,2 @@ notifyOnPrintRefundPaymentReceipt(printRefundPaymentReceiptResponse: sdk.remotepay.PrintRefundPaymentReceiptResponse): void; | ||
notifyOnInvalidStateTransitionResponse(response: sdk.remotepay.InvalidStateTransitionResponse): void; | ||
notifyOnSignatureCollected(response: sdk.remotepay.SignatureResponse): void; | ||
notifyOnCheckBalanceResponse(response: sdk.remotepay.CheckBalanceResponse): void; | ||
notifyOnTipResponse(response: sdk.remotepay.TipResponse): void; | ||
} |
@@ -132,3 +132,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
onCapturePreAuth(capturePreAuthResponseMessage: sdk.remotemessage.CapturePreAuthResponseMessage): void; | ||
onIncrementPreAuthResponse(incrementPreAuthResponseMessage: sdk.remotemessage.IncrementPreAuthResponseMessage): void; | ||
/** | ||
@@ -301,5 +300,2 @@ * Closeout Response | ||
onDisplayReceiptOptionsResponse(result: sdk.remotemessage.ResultStatus, reason: string): any; | ||
onSignatureCollected(msg: sdk.remotemessage.SignatureResponseMessage): any; | ||
onBalanceInquiryResponse(msg: sdk.remotemessage.BalanceInquiryResponseMessage): any; | ||
onRequestTipResponse(msg: sdk.remotemessage.RequestTipResponseMessage): any; | ||
} |
@@ -59,5 +59,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
* @param {sdk.order.VoidReason} reason | ||
* @param {boolean} disablePrinting | ||
* @param {boolean} disableReceiptSelection | ||
* @param {object} extras | ||
*/ | ||
@@ -83,10 +80,2 @@ abstract doVoidPayment(payment: sdk.payments.Payment, reason: sdk.order.VoidReason, disablePrinting: boolean, disableReceiptSelection: boolean, extras: object): void; | ||
/** | ||
* Capture Auth | ||
* | ||
* @param {string} paymentId | ||
* @param {number} amount | ||
* @param {number} amount | ||
*/ | ||
abstract doIncrementPreAuth(paymentId: string, amount: number): void; | ||
/** | ||
* Order Update | ||
@@ -120,3 +109,2 @@ * | ||
* @param {boolean} disableReceiptSelection | ||
* @param {object} extras | ||
*/ | ||
@@ -142,4 +130,2 @@ abstract doPaymentRefund(orderId: string, paymentId: string, amount: number, fullRefund: boolean, disablePrinting?: boolean, disableReceiptSelection?: boolean, extras?: object): void; | ||
* @param {Array<string>} textLines | ||
* @param {string} printRequestId - an optional id that will be used for the printjob. This id will be used in notification calls about the status of the job. | ||
* @param {string} printDeviceId - the printer id to use when printing. If left unset the default is used | ||
*/ | ||
@@ -170,3 +156,2 @@ abstract doPrintText(textLines: Array<string>, printRequestId?: string, printDeviceId?: string): void; | ||
* @param {string} reason | ||
* @param {string} deviceId (optional) | ||
*/ | ||
@@ -178,4 +163,2 @@ abstract doOpenCashDrawer(reason: string, deviceId?: string): void; | ||
* @param {any} bitmap | ||
* @param printRequestId | ||
* @param printDeviceId | ||
*/ | ||
@@ -187,4 +170,2 @@ abstract doPrintImageObject(bitmap: any, printRequestId?: string, printDeviceId?: string): void; | ||
* @param {string} url | ||
* @param printRequestId | ||
* @param printDeviceId | ||
*/ | ||
@@ -287,5 +268,2 @@ abstract doPrintImageUrl(url: string, printRequestId?: string, printDeviceId?: string): void; | ||
getSupportsVoidPaymentResponse(): boolean; | ||
abstract doCheckBalance(cardEntryMethods: number): void; | ||
abstract doCollectSignature(acknowledgementMessage: string): void; | ||
abstract doRequestTip(tippableAmount: number, suggestions: Array<sdk.merchant.TipSuggestion>): void; | ||
} |
@@ -89,4 +89,3 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
/** | ||
* @param transport - the way to notify is defined by the transport | ||
* @param message - the optional message to send | ||
* @param transport | ||
* @deprecated - see onDisconnected. | ||
@@ -190,3 +189,2 @@ */ | ||
notifyObserversCapturePreAuth(capturePreAuthResponseMessage: sdk.remotemessage.CapturePreAuthResponseMessage): void; | ||
notifyObserversIncrementPreAuth(incrementPreAuthResponseMessage: sdk.remotemessage.IncrementPreAuthResponseMessage): void; | ||
notifyObserversCloseout(closeoutResponseMessage: sdk.remotemessage.CloseoutResponseMessage): void; | ||
@@ -197,5 +195,2 @@ notifyObserversPendingPaymentsResponse(retrievePendingPaymentsResponseMessage: sdk.remotemessage.RetrievePendingPaymentsResponseMessage): void; | ||
notifyObserversFinishOk(finishOkMessage: sdk.remotemessage.FinishOkMessage): void; | ||
notifyObserverDisplayCollectedSignature(msg: sdk.remotemessage.SignatureResponseMessage): void; | ||
notifyObserverBalanceInquiryResponse(msg: sdk.remotemessage.BalanceInquiryResponseMessage): void; | ||
notifyObserverTipResponse(msg: sdk.remotemessage.RequestTipResponseMessage): void; | ||
notifyObserversInvalidStateTransitionResponse(invalidStateTransitionMessage: sdk.remotemessage.InvalidStateTransitionMessage): void; | ||
@@ -295,4 +290,2 @@ notifyObserverDisplayReceiptOptionsResponse(showReceiptOptionsResponseMessage: sdk.remotemessage.ShowReceiptOptionsResponseMessage): void; | ||
* @param {Array<string>} textLines | ||
* @param printRequestId - an optional id that will be used for the printjob. This id will be used in notification calls about the status of the job. | ||
* @param printDeviceId - the printer id to use when printing. If left unset the default is used | ||
*/ | ||
@@ -327,3 +320,3 @@ doPrintText(textLines: Array<string>, printRequestId?: string, printDeviceId?: string): void; | ||
* @param {sdk.payments.Payment} payment | ||
* @param {sdk.order.VoidReason} voidReason | ||
* @param {sdk.order.VoidReason} reason | ||
* @param {boolean} disablePrinting | ||
@@ -375,10 +368,2 @@ * @param {boolean} disableReceiptSelection | ||
/** | ||
* Capture Auth | ||
* | ||
* @param {string} paymentId | ||
* @param {number} amount | ||
* @param {number} amount | ||
*/ | ||
doIncrementPreAuth(paymentId: string, amount: number): void; | ||
/** | ||
* Accept Payment | ||
@@ -420,5 +405,2 @@ * | ||
doSetCustomerInfo(customerInfo: sdk.remotepay.CustomerInfo): void; | ||
doCheckBalance(cardEntryMethods: number): void; | ||
doCollectSignature(acknowledge: string): void; | ||
doRequestTip(tippableAmount: number, suggestions: Array<sdk.merchant.TipSuggestion>): void; | ||
/** | ||
@@ -425,0 +407,0 @@ * Dispose |
@@ -19,3 +19,3 @@ import { CloverWebSocketInterface } from '../../../../websocket/CloverWebSocketInterface'; | ||
getBufferedAmount(): number; | ||
connect(): void; | ||
connect(accessToken: string): void; | ||
close(code?: number, reason?: string): void; | ||
@@ -22,0 +22,0 @@ isConnecting(): boolean; |
@@ -82,2 +82,3 @@ import { HttpSupport } from '../../../../util/HttpSupport'; | ||
private afterOptionsCall; | ||
private buildAuthorizationHeader; | ||
/** | ||
@@ -84,0 +85,0 @@ * |
@@ -58,4 +58,5 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
* @param deviceEndpoint | ||
* @param accessToken | ||
*/ | ||
protected initializeWithUri(deviceEndpoint: string): void; | ||
protected initializeWithUri(deviceEndpoint: string, accessToken?: string): void; | ||
dispose(): void; | ||
@@ -62,0 +63,0 @@ private drainQueue; |
@@ -9,4 +9,2 @@ /** | ||
export declare class Endpoints { | ||
static ACCESS_TOKEN_KEY: string; | ||
static ACCESS_TOKEN_SUFFIX: string; | ||
static CLOUD_SERVER_IDENTIFIER_KEY: string; | ||
@@ -42,2 +40,4 @@ static ACCOUNT_V3_KEY: string; | ||
static WEBSOCKET_FORCE_CONNECT_SUFFIX: string; | ||
static WEBSOCKET_MERCHANT_ID_KEY: string; | ||
static WEBSOCKET_MERCHANT_ID_SUFFIX: string; | ||
static OAUTH_PATH: string; | ||
@@ -66,10 +66,9 @@ static OAUTH_CLIENT_ID_KEY: string; | ||
* | ||
* @param {any} - notificationResponse - The notification response from COS. | ||
* @param {any} notificationResponse - The notification response from COS. | ||
* @param {string} friendlyId - an id used to identify the POS. | ||
* @param {boolean} forceConnect - if true, then the attempt will overtake any existing connection | ||
* @param {boolean} merchantId - unique identifier for the merchant. | ||
* @param {boolean} accessToken - mid access token | ||
* @returns {string} The endpoint used to connect to a websocket on the server that will proxy to a device | ||
*/ | ||
static getDeviceWebSocketEndpoint(notificationResponse: any, friendlyId: string, forceConnect: boolean, merchantId: string, accessToken: string): string; | ||
static getDeviceWebSocketEndpoint(notificationResponse: any, friendlyId: string, forceConnect: boolean, merchantId: string): string; | ||
/** | ||
@@ -80,6 +79,5 @@ * The endpoint used to obtain a merchant | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* @returns {string} endpoint - the url to use to retrieve the merchant | ||
*/ | ||
static getMerchantEndpoint(domain: string, merchantId: string, accessToken: string): string; | ||
static getMerchantEndpoint(domain: string, merchantId: string): string; | ||
/** | ||
@@ -90,7 +88,6 @@ * The endpoint used to obtain a list of devices | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* | ||
* @returns {string} | ||
*/ | ||
static getDevicesEndpoint(domain: string, merchantId: string, accessToken: string): string; | ||
static getDevicesEndpoint(domain: string, merchantId: string): string; | ||
/** | ||
@@ -103,3 +100,3 @@ * Builds the endpoint to send the message to the server to let the device know we want to talk to it. | ||
*/ | ||
static getAlertDeviceEndpoint(domain: string, merchantId: string, accessToken: string): string; | ||
static getAlertDeviceEndpoint(domain: string, merchantId: string): string; | ||
static parseQueryString(queryString: String): {}; | ||
@@ -106,0 +103,0 @@ /** |
@@ -25,3 +25,3 @@ import { Logger } from '../remote/client/util/Logger'; | ||
*/ | ||
doXmlHttp(method: string, endpoint: string, onDataLoaded: Function, onError: Function): void; | ||
doXmlHttp(method: string, endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: any): void; | ||
doXmlHttpSendJson(method: string, sendData: any, endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: any): void; | ||
@@ -35,7 +35,7 @@ /** | ||
*/ | ||
getData(endpoint: string, onDataLoaded: Function, onError: Function): void; | ||
getData(endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: object): void; | ||
/** | ||
* Make the REST call to get the data | ||
*/ | ||
options(endpoint: string, onDataLoaded: Function, onError: Function): void; | ||
options(endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: object): void; | ||
/** | ||
@@ -42,0 +42,0 @@ * Make the REST call to get the data |
@@ -13,5 +13,7 @@ import { CloverWebSocketInterface } from './CloverWebSocketInterface'; | ||
* @param endpoint - the url that will connected to | ||
* @param accessToken - Here the access token is passed as a second param to `new WebSocket()` and will be read | ||
* by the support server as a "subprotocol" in the Sec-WebSocket-Protocol header value. | ||
* @returns {WebSocket} - the specific implementation of a websocket | ||
*/ | ||
createWebSocket(endpoint: string): any; | ||
createWebSocket(endpoint: string, accessToken?: string): any; | ||
/** | ||
@@ -18,0 +20,0 @@ * Browser implementations do not do pong frames |
@@ -29,5 +29,6 @@ import { WebSocketListener } from './WebSocketListener'; | ||
* @param endpoint - the uri to connect to | ||
* @param accessToken - the access token that is used to ensure the merchant and device are associated to the token | ||
*/ | ||
abstract createWebSocket(endpoint: string): any; | ||
connect(): CloverWebSocketInterface; | ||
abstract createWebSocket(endpoint: string, accessToken: any): any; | ||
connect(accessToken: string): CloverWebSocketInterface; | ||
private notifyOnOpen; | ||
@@ -34,0 +35,0 @@ private notifyOnMessage; |
{ | ||
"name": "remote-pay-cloud", | ||
"version": "3.2.1-5", | ||
"version": "3.2.2", | ||
"description": "Access Clover devices through the cloud.", | ||
@@ -35,3 +35,3 @@ "keywords": [ | ||
"eventemitter3": "3.1.0", | ||
"remote-pay-cloud-api": "4.0.0" | ||
"remote-pay-cloud-api": "3.1.0" | ||
}, | ||
@@ -47,3 +47,3 @@ "devDependencies": { | ||
"jsdoc": "3.4.3", | ||
"merge2": "^1.3.0", | ||
"merge2": "^1.2.3", | ||
"mocha": "4.0.1", | ||
@@ -50,0 +50,0 @@ "path": "0.12.7", |
@@ -12,3 +12,3 @@ 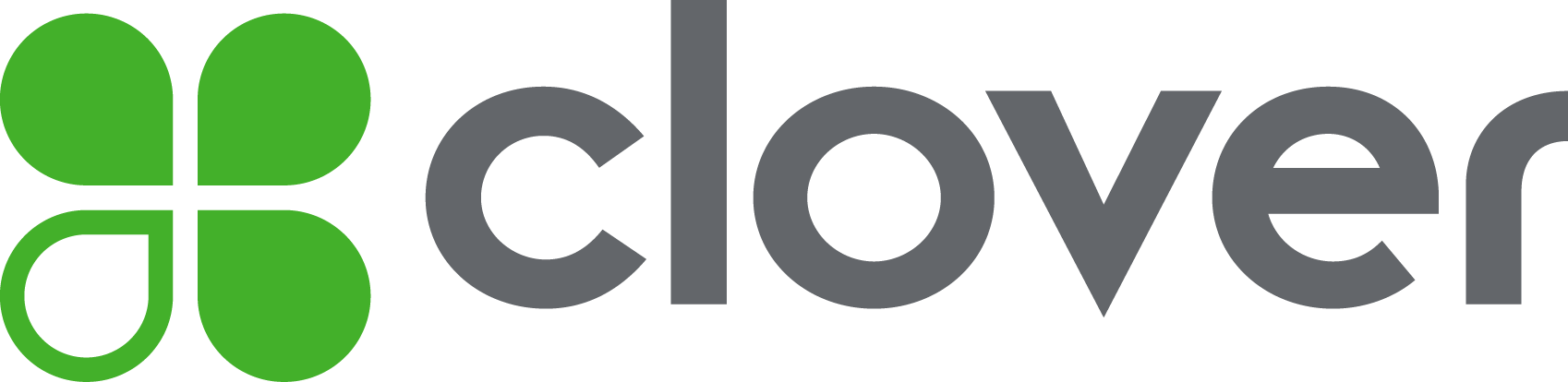 | ||
Current version: 4.0.0 | ||
Current version: 3.2.2 | ||
@@ -43,7 +43,7 @@ ## Platforms supported | ||
### Additional resources | ||
- [Online Docs](http://clover.github.io/remote-pay-cloud/3.2.0/) | ||
- [Online Docs](http://clover.github.io/remote-pay-cloud/1.4.3/) | ||
- [Release Notes](https://github.com/clover/remote-pay-cloud/releases) | ||
- [Secure Network Pay Display](https://docs.clover.com/clover-platform/docs/pay-display-apps#section--secure-network-pay-display-) | ||
- [Tutorial for the Cloud SDK](https://docs.clover.com/clover-platform/docs/cloud-sdk-v3) | ||
- [API Class Documentation](http://clover.github.io/remote-pay-cloud-api/3.2.0/) | ||
- [API Class Documentation](http://clover.github.io/remote-pay-cloud-api/1.4.2/) | ||
- [Clover Developer Community](https://community.clover.com/index.html) | ||
@@ -50,0 +50,0 @@ |
@@ -25,4 +25,2 @@ import {remotemessage} from 'remote-pay-cloud-api'; | ||
MethodToMessage.methodToType[remotemessage.Method.CAPTURE_PREAUTH] = remotemessage.CapturePreAuthMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.INCREMENT_PREAUTH_RESPONSE] = remotemessage.IncrementPreAuthResponseMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.INCREMENT_PREAUTH_REQUEST] = remotemessage.IncrementPreAuthMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.CONFIRM_PAYMENT_MESSAGE] = remotemessage.ConfirmPaymentMessage; | ||
@@ -106,6 +104,3 @@ MethodToMessage.methodToType[remotemessage.Method.LAST_MSG_REQUEST] = remotemessage.LastMessageRequestMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.SHOW_RECEIPT_OPTIONS_RESPONSE] = remotemessage.ShowReceiptOptionsResponseMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.BALANCE_INQUIRY_RESPONSE] = remotemessage.BalanceInquiryResponseMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.REQUEST_SIGNATURE_RESPONSE] = remotemessage.SignatureResponseMessage; | ||
MethodToMessage.methodToType[remotemessage.Method.REQUEST_TIP_RESPONSE] = remotemessage.RequestTipResponseMessage; | ||
} | ||
} |
@@ -181,2 +181,3 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
listener.onDeviceDisconnected(); // changed from onDisconnected in 1.3 | ||
listener.onDisconnected(); // left here for backwards compatibility. Deprecated in 1.3* | ||
} catch (e) { | ||
@@ -193,2 +194,3 @@ this.logger.error(e); | ||
listener.onDeviceReady(merchantInfo); // changed from onReady in 1.3 | ||
listener.onReady(merchantInfo); // left here for backwards compatibility. Deprecated in 1.3* | ||
} catch (e) { | ||
@@ -244,13 +246,2 @@ this.logger.error(e); | ||
public notifyOnIncrementPreAuthResponse(response: sdk.remotepay.IncrementPreAuthResponse): void { | ||
this.logger.debug('Sending IncrementPreAuth notification to listeners'); | ||
this.listeners.forEach((listener: sdk.remotepay.ICloverConnectorListener) => { | ||
try { | ||
listener.onIncrementPreAuthResponse(response); | ||
} catch (e) { | ||
this.logger.error(e); | ||
} | ||
}); | ||
} | ||
public notifyOnDeviceError(errorEvent: sdk.remotepay.CloverDeviceErrorEvent): void { | ||
@@ -474,32 +465,2 @@ this.logger.debug('Sending DeviceError notification to listeners'); | ||
public notifyOnSignatureCollected(response: sdk.remotepay.SignatureResponse): void { | ||
this.listeners.forEach((listener: sdk.remotepay.ICloverConnectorListener) => { | ||
try { | ||
listener.onRequestSignatureResponse(response); | ||
} catch (e) { | ||
this.logger.error(e); | ||
} | ||
}); | ||
} | ||
public notifyOnCheckBalanceResponse(response: sdk.remotepay.CheckBalanceResponse): void { | ||
this.listeners.forEach((listener: sdk.remotepay.ICloverConnectorListener) => { | ||
try { | ||
listener.onCheckBalanceResponse(response); | ||
} catch (e) { | ||
this.logger.error(e); | ||
} | ||
}); | ||
} | ||
public notifyOnTipResponse(response: sdk.remotepay.TipResponse): void { | ||
this.listeners.forEach((listener: sdk.remotepay.ICloverConnectorListener) => { | ||
try { | ||
listener.onRequestTipResponse(response); | ||
} catch (e) { | ||
this.logger.error(e); | ||
} | ||
}); | ||
} | ||
} |
@@ -140,3 +140,3 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
let devicesEndpoint: string = Endpoints.getDevicesEndpoint( | ||
configuration.domain, configuration.merchantId, configuration.oauthToken); | ||
configuration.domain, configuration.merchantId); | ||
this.httpSupport.getData(devicesEndpoint, | ||
@@ -152,3 +152,4 @@ function (devices) { | ||
this.broadcaster.notifyOnDeviceError(errorResponse); | ||
}.bind(this) | ||
}.bind(this), | ||
{"Authorization": `Bearer ${configuration.oauthToken}`} | ||
); | ||
@@ -155,0 +156,0 @@ } |
@@ -152,4 +152,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
onIncrementPreAuthResponse(incrementPreAuthResponseMessage: sdk.remotemessage.IncrementPreAuthResponseMessage): void; | ||
/** | ||
@@ -347,7 +345,2 @@ * Closeout Response | ||
onSignatureCollected(msg: sdk.remotemessage.SignatureResponseMessage); | ||
onBalanceInquiryResponse(msg: sdk.remotemessage.BalanceInquiryResponseMessage); | ||
onRequestTipResponse(msg: sdk.remotemessage.RequestTipResponseMessage); | ||
} |
@@ -80,5 +80,2 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
* @param {sdk.order.VoidReason} reason | ||
* @param {boolean} disablePrinting | ||
* @param {boolean} disableReceiptSelection | ||
* @param {object} extras | ||
*/ | ||
@@ -107,11 +104,2 @@ public abstract doVoidPayment(payment: sdk.payments.Payment, reason: sdk.order.VoidReason, disablePrinting: boolean, disableReceiptSelection: boolean, extras: object): void; | ||
/** | ||
* Capture Auth | ||
* | ||
* @param {string} paymentId | ||
* @param {number} amount | ||
* @param {number} amount | ||
*/ | ||
public abstract doIncrementPreAuth(paymentId: string, amount: number): void; | ||
/** | ||
* Order Update | ||
@@ -148,3 +136,2 @@ * | ||
* @param {boolean} disableReceiptSelection | ||
* @param {object} extras | ||
*/ | ||
@@ -173,4 +160,2 @@ public abstract doPaymentRefund(orderId: string, paymentId: string, amount: number, fullRefund: boolean, disablePrinting?: boolean, disableReceiptSelection?: boolean, extras?: object): void; | ||
* @param {Array<string>} textLines | ||
* @param {string} printRequestId - an optional id that will be used for the printjob. This id will be used in notification calls about the status of the job. | ||
* @param {string} printDeviceId - the printer id to use when printing. If left unset the default is used | ||
*/ | ||
@@ -207,3 +192,2 @@ public abstract doPrintText(textLines: Array<string>, printRequestId?: string, printDeviceId?: string): void; | ||
* @param {string} reason | ||
* @param {string} deviceId (optional) | ||
*/ | ||
@@ -216,4 +200,2 @@ public abstract doOpenCashDrawer(reason: string, deviceId?: string): void; | ||
* @param {any} bitmap | ||
* @param printRequestId | ||
* @param printDeviceId | ||
*/ | ||
@@ -226,4 +208,2 @@ public abstract doPrintImageObject(bitmap: any, printRequestId?: string, printDeviceId?: string): void; | ||
* @param {string} url | ||
* @param printRequestId | ||
* @param printDeviceId | ||
*/ | ||
@@ -354,8 +334,2 @@ public abstract doPrintImageUrl(url: string, printRequestId?: string, printDeviceId?: string): void; | ||
} | ||
public abstract doCheckBalance(cardEntryMethods: number): void; | ||
public abstract doCollectSignature(acknowledgementMessage: string): void; | ||
public abstract doRequestTip(tippableAmount: number, suggestions: Array<sdk.merchant.TipSuggestion>): void; | ||
} |
@@ -35,3 +35,3 @@ import {CloverWebSocketInterface} from '../../../../websocket/CloverWebSocketInterface'; | ||
public connect(): void { | ||
public connect(accessToken: string): void { | ||
if (this.socket != null) { | ||
@@ -45,3 +45,3 @@ throw new Error("Socket already created. Must create a new CloverWebSocketClient"); | ||
this.socket.addListener(this); | ||
this.socket.connect(); | ||
this.socket.connect(accessToken); | ||
} catch (e) { | ||
@@ -48,0 +48,0 @@ this.logger.error('connect, connectionError', e); |
@@ -79,7 +79,8 @@ import {HttpSupport} from '../../../../util/HttpSupport'; | ||
// See http://jonnyreeves.co.uk/2013/making-xhr-request-to-https-domains-with-winjs/ for more information. | ||
this.httpSupport.getData(Endpoints.getMerchantEndpoint(this.cloverServer, this.merchantId, this.accessToken), | ||
this.httpSupport.getData(Endpoints.getDevicesEndpoint(this.cloverServer, this.merchantId), | ||
(data) => this.obtainWebSocketUrlAndSendPushAlert(), | ||
(error) => { | ||
this.logger.warn("IE 11 - Initial GET failed.", error); | ||
}); | ||
}, | ||
this.buildAuthorizationHeader(this.accessToken)); | ||
} else { | ||
@@ -107,3 +108,3 @@ // We aren't using IE, make the initial POST. | ||
// already running, but this is not harmful. | ||
let alertEndpoint: string = Endpoints.getAlertDeviceEndpoint(this.cloverServer, this.merchantId, this.accessToken); | ||
let alertEndpoint: string = Endpoints.getAlertDeviceEndpoint(this.cloverServer, this.merchantId); | ||
let deviceContactInfo: DeviceContactInfo = new DeviceContactInfo(this.deviceId.replace(/-/g, ""), true); | ||
@@ -115,3 +116,4 @@ this.httpSupport.postData(alertEndpoint, | ||
}, | ||
deviceContactInfo); | ||
deviceContactInfo, | ||
this.buildAuthorizationHeader(this.accessToken)); | ||
} | ||
@@ -133,3 +135,3 @@ | ||
if (!notificationResponse.hasOwnProperty('sent') || notificationResponse.sent) { | ||
const deviceWebSocketEndpoint: string = Endpoints.getDeviceWebSocketEndpoint(notificationResponse, this.friendlyId, this.forceConnect, this.merchantId, this.accessToken); | ||
const deviceWebSocketEndpoint: string = Endpoints.getDeviceWebSocketEndpoint(notificationResponse, this.friendlyId, this.forceConnect, this.merchantId); | ||
this.doOptionsCallToAvoid401Error(deviceWebSocketEndpoint); | ||
@@ -162,3 +164,4 @@ } else { | ||
(data, xmlHttpReqImpl) => this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl), | ||
(data, xmlHttpReqImpl) => this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl)); | ||
(data, xmlHttpReqImpl) => this.afterOptionsCall(deviceWebSocketEndpoint, xmlHttpReqImpl), | ||
this.buildAuthorizationHeader(this.accessToken)); | ||
} | ||
@@ -202,4 +205,8 @@ | ||
} | ||
super.initializeWithUri(deviceWebSocketEndpoint); | ||
super.initializeWithUri(deviceWebSocketEndpoint, this.accessToken); | ||
} | ||
private buildAuthorizationHeader(token: string): object { | ||
return {"Authorization": `Bearer ${token}`}; | ||
} | ||
@@ -206,0 +213,0 @@ /** |
@@ -129,4 +129,5 @@ import * as sdk from 'remote-pay-cloud-api'; | ||
* @param deviceEndpoint | ||
* @param accessToken | ||
*/ | ||
protected initializeWithUri(deviceEndpoint: string): void { // synchronized | ||
protected initializeWithUri(deviceEndpoint: string, accessToken?: string): void { // synchronized | ||
if (this.cloverWebSocketClient != null) { | ||
@@ -140,3 +141,3 @@ if (this.cloverWebSocketClient.isOpen() || this.cloverWebSocketClient.isConnecting()) { | ||
this.cloverWebSocketClient = new CloverWebSocketClient(deviceEndpoint, this, this.webSocketImplClass); | ||
this.cloverWebSocketClient.connect(); | ||
this.cloverWebSocketClient.connect(accessToken); | ||
this.logger.info('Connection attempt complete.'); | ||
@@ -143,0 +144,0 @@ this.notifyConnectionAttemptComplete(); |
@@ -9,6 +9,2 @@ /** | ||
export class Endpoints { | ||
static ACCESS_TOKEN_KEY: string = "axsTkn"; | ||
static ACCESS_TOKEN_SUFFIX: string = "?access_token={" + Endpoints.ACCESS_TOKEN_KEY + "}"; | ||
// The key of the server identifier that COS will return in the cloud server URL. | ||
@@ -53,2 +49,4 @@ // See WebSocketCloudCloverTransport.initialize | ||
static WEBSOCKET_FORCE_CONNECT_SUFFIX = "&forceConnect={" + Endpoints.WEBSOCKET_FORCE_CONNECT_KEY + "}"; | ||
static WEBSOCKET_MERCHANT_ID_KEY: string = "mid"; | ||
static WEBSOCKET_MERCHANT_ID_SUFFIX = "&wsMid={" + Endpoints.WEBSOCKET_MERCHANT_ID_KEY + "}"; | ||
@@ -95,10 +93,9 @@ static OAUTH_PATH: string = "oauth/authorize?response_type=token"; | ||
* | ||
* @param {any} - notificationResponse - The notification response from COS. | ||
* @param {any} notificationResponse - The notification response from COS. | ||
* @param {string} friendlyId - an id used to identify the POS. | ||
* @param {boolean} forceConnect - if true, then the attempt will overtake any existing connection | ||
* @param {boolean} merchantId - unique identifier for the merchant. | ||
* @param {boolean} accessToken - mid access token | ||
* @returns {string} The endpoint used to connect to a websocket on the server that will proxy to a device | ||
*/ | ||
public static getDeviceWebSocketEndpoint(notificationResponse: any, friendlyId: string, forceConnect: boolean, merchantId: string, accessToken: string): string { | ||
public static getDeviceWebSocketEndpoint(notificationResponse: any, friendlyId: string, forceConnect: boolean, merchantId: string): string { | ||
const variables = {}; | ||
@@ -109,2 +106,3 @@ variables[Endpoints.WEBSOCKET_TOKEN_KEY] = notificationResponse.token; | ||
variables[Endpoints.WEBSOCKET_FORCE_CONNECT_KEY] = forceConnect; | ||
variables[Endpoints.WEBSOCKET_MERCHANT_ID_KEY] = merchantId; | ||
@@ -114,3 +112,4 @@ let deviceWebSocketEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.WEBSOCKET_PATH + | ||
Endpoints.WEBSOCKET_FRIENDLY_ID_SUFFIX + | ||
Endpoints.WEBSOCKET_FORCE_CONNECT_SUFFIX | ||
Endpoints.WEBSOCKET_FORCE_CONNECT_SUFFIX + | ||
Endpoints.WEBSOCKET_MERCHANT_ID_SUFFIX; | ||
@@ -129,12 +128,10 @@ if (notificationResponse.serverIdentifier) { | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* @returns {string} endpoint - the url to use to retrieve the merchant | ||
*/ | ||
public static getMerchantEndpoint(domain: string, merchantId: string, accessToken: string): string { | ||
public static getMerchantEndpoint(domain: string, merchantId: string): string { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
let merchantEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.MERCHANT_V3_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
let merchantEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.MERCHANT_V3_PATH; | ||
return Endpoints.setVariables(merchantEndpointPath, variables); | ||
@@ -148,13 +145,11 @@ } | ||
* @param {string} merchantId - the id of the merchant to use when getting the merchant. | ||
* @param {string} accessToken - the OAuth token used when accessing the server | ||
* | ||
* @returns {string} | ||
*/ | ||
public static getDevicesEndpoint(domain: string, merchantId: string, accessToken: string): string { | ||
public static getDevicesEndpoint(domain: string, merchantId: string): string { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
let devicesEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.DEVICE_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
let devicesEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.DEVICE_PATH; | ||
return Endpoints.setVariables(devicesEndpointPath, variables); | ||
@@ -170,9 +165,8 @@ } | ||
*/ | ||
public static getAlertDeviceEndpoint(domain: string, merchantId: string, accessToken: string): string { | ||
public static getAlertDeviceEndpoint(domain: string, merchantId: string): string { | ||
var variables = {}; | ||
variables[Endpoints.MERCHANT_V3_KEY] = merchantId; | ||
variables[Endpoints.ACCESS_TOKEN_KEY] = accessToken; | ||
variables[Endpoints.DOMAIN_KEY] = domain; | ||
let alertDeviceEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.REMOTE_PAY_PATH + Endpoints.ACCESS_TOKEN_SUFFIX; | ||
let alertDeviceEndpointPath: string = Endpoints.DOMAIN_PATH + Endpoints.REMOTE_PAY_PATH; | ||
return Endpoints.setVariables(alertDeviceEndpointPath, variables); | ||
@@ -179,0 +173,0 @@ } |
import {Logger} from '../remote/client/util/Logger'; | ||
import {addHeaders} from "./addHeaders"; | ||
@@ -65,3 +66,3 @@ /** | ||
*/ | ||
public doXmlHttp(method: string, endpoint: string, onDataLoaded: Function, onError: Function): void { | ||
public doXmlHttp(method: string, endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: any): void { | ||
const xmlHttp = new this.xmlHttpImplClass(); | ||
@@ -75,3 +76,3 @@ this.setXmlHttpCallback(xmlHttp, endpoint, onDataLoaded, onError); | ||
} | ||
addHeaders(additionalHeaders, xmlHttp); | ||
xmlHttp.send(); | ||
@@ -85,9 +86,3 @@ } | ||
xmlHttp.open(method, endpoint, true); | ||
if (additionalHeaders) { | ||
for (var key in additionalHeaders) { | ||
if (additionalHeaders.hasOwnProperty(key)) { | ||
xmlHttp.setRequestHeader(key, additionalHeaders[key]); | ||
} | ||
} | ||
} | ||
addHeaders(additionalHeaders, xmlHttp); | ||
if (sendData) { | ||
@@ -113,4 +108,4 @@ xmlHttp.setRequestHeader("Content-Type", "application/json;charset=UTF-8"); | ||
*/ | ||
public getData(endpoint: string, onDataLoaded: Function, onError: Function): void { | ||
this.doXmlHttp("GET", endpoint, onDataLoaded, onError) | ||
public getData(endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: object): void { | ||
this.doXmlHttp("GET", endpoint, onDataLoaded, onError, additionalHeaders) | ||
} | ||
@@ -121,4 +116,4 @@ | ||
*/ | ||
public options(endpoint: string, onDataLoaded: Function, onError: Function): void { | ||
this.doXmlHttp("OPTIONS", endpoint, onDataLoaded, onError) | ||
public options(endpoint: string, onDataLoaded: Function, onError: Function, additionalHeaders?: object): void { | ||
this.doXmlHttp("OPTIONS", endpoint, onDataLoaded, onError, additionalHeaders) | ||
} | ||
@@ -125,0 +120,0 @@ |
@@ -9,3 +9,3 @@ /** | ||
*/ | ||
public static CLOVER_CLOUD_SDK_VERSION = "4.0.0"; | ||
public static CLOVER_CLOUD_SDK_VERSION = "3.2.2"; | ||
@@ -12,0 +12,0 @@ /** |
@@ -18,6 +18,8 @@ import {CloverWebSocketInterface} from './CloverWebSocketInterface' | ||
* @param endpoint - the url that will connected to | ||
* @param accessToken - Here the access token is passed as a second param to `new WebSocket()` and will be read | ||
* by the support server as a "subprotocol" in the Sec-WebSocket-Protocol header value. | ||
* @returns {WebSocket} - the specific implementation of a websocket | ||
*/ | ||
public createWebSocket(endpoint: string): any { | ||
return new WebSocket(endpoint); | ||
public createWebSocket(endpoint: string, accessToken?: string): any { | ||
return new WebSocket(endpoint, accessToken); | ||
} | ||
@@ -24,0 +26,0 @@ |
@@ -41,7 +41,8 @@ import {WebSocketListener} from './WebSocketListener' | ||
* @param endpoint - the uri to connect to | ||
* @param accessToken - the access token that is used to ensure the merchant and device are associated to the token | ||
*/ | ||
public abstract createWebSocket(endpoint: string): any; | ||
public abstract createWebSocket(endpoint: string, accessToken): any; | ||
public connect(): CloverWebSocketInterface { | ||
this.webSocket = this.createWebSocket(this.endpoint); | ||
public connect(accessToken: string): CloverWebSocketInterface { | ||
this.webSocket = this.createWebSocket(this.endpoint, accessToken); | ||
if (typeof this.webSocket["addEventListener"] !== "function") { | ||
@@ -48,0 +49,0 @@ this.logger.error("FATAL: The websocket implementation being used must have an 'addEventListener' function. Either use a supported websocket implementation (https://www.npmjs.com/package/ws) or override the connect method on CloverWebSocketInterface."); |
@@ -11,4 +11,4 @@ export {Logger} from "../src/com/clover/remote/client/util/Logger"; | ||
export {WebSocketCloverDeviceConfiguration} from "../src/com/clover/remote/client/device/WebSocketCloverDeviceConfiguration"; | ||
export {WebSocketPairedCloverDeviceConfiguration, WebSocketPairedCloverDeviceConfigurationBuilder} from "../src/com/clover/remote/client/device/WebSocketPairedCloverDeviceConfiguration"; | ||
export {WebSocketCloudCloverDeviceConfiguration, WebSocketCloudCloverDeviceConfigurationBuilder} from "../src/com/clover/remote/client/device/WebSocketCloudCloverDeviceConfiguration"; | ||
export {WebSocketPairedCloverDeviceConfiguration} from "../src/com/clover/remote/client/device/WebSocketPairedCloverDeviceConfiguration"; | ||
export {WebSocketCloudCloverDeviceConfiguration} from "../src/com/clover/remote/client/device/WebSocketCloudCloverDeviceConfiguration"; | ||
@@ -33,3 +33,3 @@ export {CloverTransport} from "../src/com/clover/remote/client/transport/CloverTransport"; | ||
guid(): string; | ||
}; | ||
} | ||
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
242
2
5459959
80829
+ Addedremote-pay-cloud-api@3.1.0(transitive)
- Removedremote-pay-cloud-api@4.0.0(transitive)
Updatedremote-pay-cloud-api@3.1.0