remote-storage
Advanced tools
Comparing version 0.0.6 to 0.0.7
{ | ||
"name": "remote-storage", | ||
"version": "0.0.6", | ||
"version": "0.0.7", | ||
"description": "remoteStorage is a Javascript library with the same API as localStorage that allows you to store state in a centralized location.", | ||
@@ -5,0 +5,0 @@ "type": "module", |
193
README.md
@@ -1,8 +0,9 @@ | ||
[](https://www.npmjs.com/package/@frigade/js) | ||
[](https://github.com/FrigadeHQ/javascript/actions/workflows/tests.yml) | ||
[](https://www.npmjs.com/package/@frigade/js) | ||
[](https://www.npmjs.com/package/global-storage) | ||
[](https://github.com/FrigadeHQ/react-native-onboard/actions/workflows/tests.yml) | ||
[](https://www.npmjs.com/package/react-native-onboard) | ||
[](https://github.com/prettier/prettier) | ||
<H3 align="center"><strong>Frigade Javascript SDK</strong></H3> | ||
<div align="center">The easiest way for developers to build high-quality product onboarding and education.</div> | ||
<H3 align="center"><strong>remoteStorage</strong></H3> | ||
<div align="center">remoteStorage is a simple library that combines the localStorage API with a remote server to persist data across browsers and devices.</div> | ||
<br /> | ||
@@ -20,168 +21,88 @@ <div align="center"> | ||
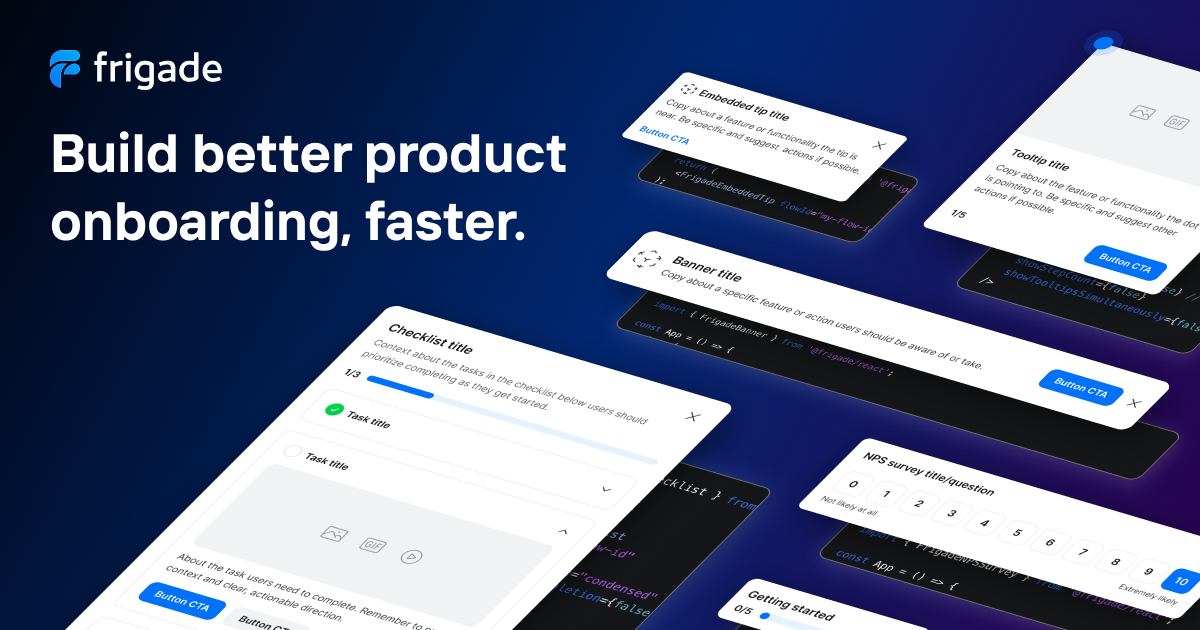 | ||
## Install | ||
## Why | ||
Storing data in localStorage is useful, but it's not a good solution when you store data that needs to be shared across multiple devices or browsers. | ||
Install the package from your command line. | ||
For instance, let's say you want to show a welcome modal to all new users that sign up for your product. If you use localStorage to track if a user has already seen this modal, your users will continue to get the experience over and over again every time they switch devices or browsers. | ||
#### With yarn | ||
That's where remoteStorage comes in. Using the same API as localStorage, remoteStorage allows you to easily read and write data on the fly while maintaining state across browsers and devices in order to provide a better user experience. | ||
```bash | ||
yarn add @frigade/js | ||
``` | ||
## Features | ||
#### With npm | ||
- 🔧 Simple API (same as localStorage) | ||
- 🚀 Works with all Javascript frameworks | ||
- 📦 Lightweight (~2 kB) | ||
- ✨ Open source server and client (MIT license) | ||
- 🍦 Free hosted community server | ||
## Quick start | ||
Install the library: | ||
```bash | ||
npm install @frigade/js | ||
npm install remote-storage | ||
``` | ||
## Usage | ||
Import the library and use it like you would localStorage: | ||
Simply `import { Frigade } from '@frigade/js'` and use it. Example: | ||
```javascript | ||
import { RemoteStorage } from 'remote-storage' | ||
```js | ||
import {Frigade} from 'packages/js-client' | ||
const frigade = new Frigade('FRIGADE_API_KEY') | ||
const remoteStorage = new RemoteStorage({ serverUrl: 'https://server.centralstorage.dev' }) | ||
await frigade.identify('USER_ID', { | ||
name: 'USER_NAME', | ||
email: 'USER_EMAIL', | ||
signed_up_at: 'USER_SIGNED_UP_AT' // ISO 8601 format | ||
}) | ||
// Optional: send organization/group id | ||
await frigade.group('ORGANIZATION_ID', { | ||
name: 'COMPANY_NAME', | ||
}) | ||
``` | ||
const hasSeenNewFeature = await remoteStorage.getItem('hasSeenNewFeature') | ||
## API Reference | ||
#### Get a flow: | ||
```js | ||
const flow = await frigade.getFlow('FLOW_ID') | ||
// Flow data defined in config.yml in the Frigade dashboard | ||
console.log('Flow status:', flow.isCompleted) | ||
console.log('Flow data:', flow.rawData) | ||
if (!hasSeenNewFeature) { | ||
await remoteStorage.setItem('hasSeenNewFeature', true) | ||
} | ||
``` | ||
#### Marking a flow as completed: | ||
That's it! | ||
```js | ||
const flow = await frigade.getFlow('FLOW_ID') | ||
await flow.complete() | ||
``` | ||
## Documentation | ||
#### Marking a step in a flow as completed: | ||
### User IDs | ||
```js | ||
const flow = await frigade.getFlow('FLOW_ID') | ||
const step = flow.steps.get('STEP_ID') | ||
await step.start() | ||
await step.complete() | ||
``` | ||
remoteStorage uses user IDs to identify users. A user ID is a string that uniquely identifies a user. It can be anything you want, but we recommend using a UUID. | ||
#### Sending tracking events: | ||
The User ID is set when you create a new instance of remoteStorage: | ||
```js | ||
await frigade.track('EVENT_NAME', { | ||
property1: 'value1', | ||
property2: 'value2', | ||
```javascript | ||
const remoteStorage = new RemoteStorage({ | ||
serverUrl: 'https://server.centralstorage.dev', | ||
userId: '123e4567-e89b-12d3-a456-426614174000' | ||
}) | ||
``` | ||
#### Event handlers | ||
Global event handlers can be registered to be notified when any state change occurs in any flow. For example, to be notified when a flow is completed: | ||
### Instance IDs | ||
```js | ||
// This callback will be called when a the current user/group changes state in any flow | ||
const callback = (updatedFlow, previousFlow) => { | ||
console.log('Flow state changed:', flow.isCompleted) | ||
console.log('Step state changed:', flow.steps.get('STEP_ID').isCompleted) | ||
}; | ||
remoteStorage uses instance IDs to identify the application instance that is making the request. An instance ID is a string that uniquely identifies an application instance. Typically you would use the same instance ID for all requests from the same application instance. | ||
frigade.onStateChange(callback); | ||
// To remove the callback use: | ||
frigade.removeOnFlowStateChangeHandler(callback); | ||
``` | ||
Flow specific event handlers can be registered to be notified when a specific flow changes state. For example, to be notified when a flow is completed: | ||
The instance ID is set when you create a new instance of remoteStorage: | ||
```js | ||
// This callback will be called when a the current user/group changes state in the flow with id FLOW_ID | ||
const flow = await frigade.getFlow('FLOW_ID') | ||
const callback = (updatedFlow, previousFlow) => { | ||
console.log('Flow state changed:', flow.isCompleted) | ||
console.log('Step state changed:', flow.steps.get('STEP_ID').isCompleted) | ||
}; | ||
flow.onStateChange(callback); | ||
```javascript | ||
const remoteStorage = new RemoteStorage({ | ||
serverUrl: 'https://server.centralstorage.dev', | ||
userId: '123e4567-e89b-12d3-a456-426614174000', | ||
instanceId: 'my-cool-app' | ||
}) | ||
``` | ||
To only target a specific step in a flow, use: | ||
```js | ||
// This callback will be called when a the current user/group changes state in the flow with id FLOW_ID and step with id STEP_ID | ||
const flow = await frigade.getFlow('FLOW_ID') | ||
const step = flow.steps.get('STEP_ID') | ||
const callback = (updatedStep, previousStep) => { | ||
console.log('Step state changed:', step.isCompleted) | ||
}; | ||
step.onStateChange(callback); | ||
``` | ||
### Server | ||
## Cross-platform support | ||
We offer a free hosted community server at `https://server.centraldstorage.dev`. The server should not be used for production apps, but it's great for testing and prototyping. | ||
All non-UI related functionality of the SDK works in all JavaScript environments (Node.js, browser, React Native, etc.). | ||
The server can be spun up using Docker in a few minutes. To get started, simply clone the repository and run `docker-compose up`: | ||
## TypeScript support | ||
```bash | ||
git clone git@github.com:FrigadeHQ/remote-storage.git | ||
cd remote-storage/apps/server | ||
docker-compose up | ||
``` | ||
This package contains TypeScript definitions of the `frigade` object. | ||
The server runs on port 4000 by default. | ||
## About Frigade | ||
[Frigade](<https://frigade.com>) is a developer-first platform for building quality product onboarding. A powerful, | ||
flexible API and native SDKs allow you to build onboarding 10x faster, experiment more easily, and drive customer | ||
success. | ||
Frigade supports a series of use cases such as: | ||
- **Registration**: Maximize the number of users getting through your sign up flows with beautiful explainers, progress | ||
bars, and forms. | ||
- **Activation**: Convert more customers by taking them through a series of key onboarding items specific to their role, | ||
permissions, or goals. | ||
- **Adoption**: Introduce audiences to specific features that deliver value with native hotspots, tooltips, tours, and | ||
interactive product guides. | ||
- **Engagement**: Keep active customers engaged, announce new product features, and create lifecycle specific | ||
re-onboarding flows for dormant or churned customers. | ||
- **Retention**: Increase retention by delivering the right content at the right time, and by asking your users for | ||
feedback on the product. | ||
# Features | ||
**Component Library** | ||
Unstyled, ready-made components for building high‑quality user onboarding, faster. Onboarding checklists, tooltips, | ||
product walkthroughs, and much more. [See components](https://frigade.com/components) | ||
**Integrations** | ||
Integrations with Segment, Mixpanel, Posthog, and more to power targeting, analytics, and communications. | ||
**Content Management** | ||
Lightweight CMS built-in to update and test onboarding copy and content. | ||
**Versioning** | ||
Frigade makes it easy to manage multiple versions of onboarding across staging and production. Revisit previous versions | ||
of onboarding to see how they performed and make improvements. | ||
**Customer Journeys** | ||
Frigade automatically tracks state management and onboarding progress. Give your team full observability into the | ||
customer journey, and use Frigade to kick off automated workflows. | ||
To learn more, visit [frigade.com](<https://frigade.com>) | ||
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
0
15948
108