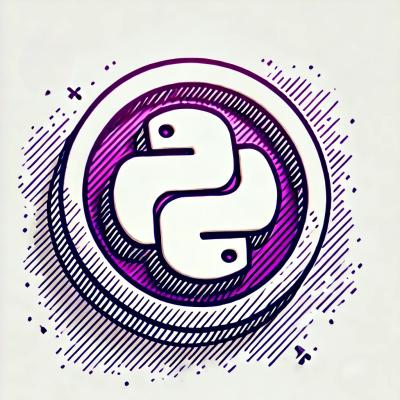
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The 'replace' npm package is a utility for performing search and replace operations on files. It allows you to replace text in files using a simple API, making it useful for tasks such as updating configuration files, modifying source code, or performing batch text replacements.
Basic Text Replacement
This feature allows you to replace occurrences of a specified string (in this case, 'foo') with another string ('bar') in the specified file ('file.txt'). The 'recursive' option allows the operation to be performed in all files within the specified directory, and 'silent' suppresses the output.
const replace = require('replace');
replace({
regex: 'foo',
replacement: 'bar',
paths: ['file.txt'],
recursive: true,
silent: true
});
Regular Expression Replacement
This feature allows you to use regular expressions for more complex search patterns. In this example, any string matching the pattern 'foo' followed by one or more digits will be replaced with 'bar'.
const replace = require('replace');
replace({
regex: /foo\d+/g,
replacement: 'bar',
paths: ['file.txt'],
recursive: true,
silent: true
});
Replacement with Function
This feature allows you to use a function to determine the replacement text. In this example, any occurrence of 'foo' will be replaced with its uppercase version.
const replace = require('replace');
replace({
regex: 'foo',
replacement: (match) => match.toUpperCase(),
paths: ['file.txt'],
recursive: true,
silent: true
});
The 'replace-in-file' package provides similar functionality to 'replace' but with a more modern API and additional features such as dry-run mode and custom encoding support. It is often preferred for its ease of use and additional options.
The 'string-replace-loader' package is a webpack loader that allows you to perform string replacements in your source files during the build process. It is useful for build-time replacements and integrates seamlessly with webpack.
The 'gulp-replace' package is a plugin for the Gulp task runner that allows you to perform string replacements in your Gulp pipelines. It is ideal for automating text replacements as part of your build and deployment workflows.
replace
is a command line utility for performing search-and-replace on files. It's similar to sed but there are a few differences:
npm install replace -g
You can now use replace
and search
from the command line.
Replace all occurrences of "foo" with "bar" in files in the current directory:
replace 'foo' 'bar' *
Replace in all files in a recursive search of the current directory:
replace 'foo' 'bar' . -r
Replace only in test/file1.js and test/file2.js:
replace 'foo' 'bar' test/file1.js test/file2.js
Replace all word pairs with "_" in middle with a "-":
replace '(\w+)_(\w+)' '$1-$2' *
Replace only in files with names matching *.js:
replace 'foo' 'bar' . -r --include="*.js"
Don't replace in files with names matching *.min.js and *.py:
replace 'foo' 'bar' . -r --exclude="*.min.js,*.py"
Preview the replacements without modifying any files:
replace 'foo' 'bar' . -r --preview
See all the options:
replace -h
There's also a search
command. It's like grep
, but with replace
's syntax.
search "setTimeout" . -r
You can use replace from your JS program:
var replace = require("replace");
replace({
regex: "foo",
replacement: "bar",
paths: ['.'],
recursive: true,
silent: true,
});
By default, replace
and search
will exclude files (binaries, images, etc) that match patterns in the "defaultignore"
located in this directory.
If replace
is taking too long on a large directory, try turning on the quiet flag with -q
, only including the necessary file types with --include
or limiting the lines shown in a preview with -n
.
FAQs
Command line search and replace utility
The npm package replace receives a total of 17,019 weekly downloads. As such, replace popularity was classified as popular.
We found that replace demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.