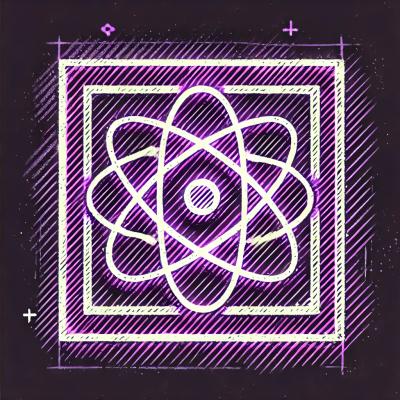
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
resilient-fetch
Advanced tools
Resilient Fetch is a powerful utility library designed to enhance your HTTP requests by providing built-in resilience features. It's especially useful when dealing with unreliable network conditions, ensuring your applications remain robust and responsive even in challenging environments.
Key Features:
onResponse
and onError
callbacks for custom handling of responses and errors.# Using npm
npm install --save resilient-fetch
# Using yarn
yarn add resilient-fetch
import { resilientFetch } from 'resilient-fetch';
// Example 1: Fetch data with default options
const fetchDataExample1 = async () => {
try {
const { promise, cancel } = resilientFetch<string>(
'https://api.example.com/data'
);
const response = await promise;
if (response.loading) {
console.log('Loading...');
} else if (response.status === 'success') {
console.log('Success:', response.data);
console.log('Status:', response.status);
console.log('Headers:', response.headers);
} else {
console.error('Error:', response.error);
console.log('Status:', response.status);
}
// Example cancellation:
// cancel();
} catch (error) {
console.error('Catch Block Error:', error.error);
console.log('Catch Block Status:', error.status);
}
};
// Call the example function
fetchDataExample1();
// Example 2: Fetch data with custom options
const fetchDataExample2 = async () => {
try {
const { promise, cancel } = resilientFetch<string>(
'https://api.example.com/data',
{
headers: { Authorization: 'Bearer token123' },
method: 'POST',
// Add more options as needed
},
{
timeout: 15000,
retryAttempts: 3,
onResponse: async (response) => {
// Handle response interceptors if needed
},
onError: (error) => {
// Handle global error handling
console.error('Global Error Handling:', error);
},
}
);
const response = await promise;
if (response.loading) {
console.log('Loading...');
} else if (response.status === 'success') {
console.log('Success:', response.data);
console.log('Status:', response.status);
console.log('Headers:', response.headers);
} else {
console.error('Error:', response.error);
console.log('Status:', response.status);
}
// Example cancellation:
// cancel();
} catch (error) {
console.error('Catch Block Error:', error.error);
console.log('Catch Block Status:', error.status);
}
};
// Call the example function
fetchDataExample2();
resilientFetch<T>(url: string, options?: RequestInit, customOptions?: ResilientFetchOptions<T>): { promise: Promise<ResilientFetchResponse<T>>, cancel: () => void }
url
: The URL to fetch data from.options
: Optional request options (same as the standard fetch
function).customOptions
: Optional custom options for resilientFetch
: - timeout
: Timeout duration in milliseconds (default: 5000). - retryAttempts
: Number of retry attempts (default: 3). - onResponse
: Callback function to handle the response before resolving the promise. - onError
: Callback function to handle global errors.
Returns an object with a promise
that resolves to a ResilientFetchResponse<T>
and a cancel
function to abort the request.ResilientFetchResponse<T>
loading
: Boolean indicating whether the request is still loading.data
: The fetched data (nullable).status
: The status of the request ('success', 'error', or null).error
: An object containing error details (nullable).headers
: The response headers.ResilientFetchError
status
: The HTTP status code of the error.message
: The error message.This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A resilient HTTP fetch utility
The npm package resilient-fetch receives a total of 0 weekly downloads. As such, resilient-fetch popularity was classified as not popular.
We found that resilient-fetch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.