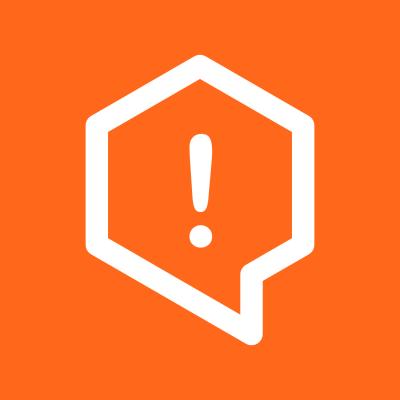
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
resp-modifier
Advanced tools
The resp-modifier npm package allows you to modify HTTP responses on the fly. It is particularly useful for tasks such as injecting scripts, modifying HTML content, or altering headers in HTTP responses.
Modify HTML Content
This feature allows you to modify the HTML content of the response. In this example, the title of the HTML document is changed from 'Original Title' to 'Modified Title'.
const http = require('http');
const respModifier = require('resp-modifier');
const server = http.createServer((req, res) => {
const modifier = respModifier({
modify: (req, res, body) => {
return body.replace(/<title>(.*?)<\/title>/, '<title>Modified Title</title>');
}
});
modifier(req, res, () => {
res.end('<html><head><title>Original Title</title></head><body>Hello World</body></html>');
});
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Inject Scripts
This feature allows you to inject scripts into the HTML content of the response. In this example, a script that shows an alert with the message 'Hello World' is injected before the closing </body> tag.
const http = require('http');
const respModifier = require('resp-modifier');
const server = http.createServer((req, res) => {
const modifier = respModifier({
modify: (req, res, body) => {
return body.replace('</body>', '<script>alert("Hello World");</script></body>');
}
});
modifier(req, res, () => {
res.end('<html><head><title>Test</title></head><body></body></html>');
});
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Modify Headers
This feature allows you to modify the headers of the HTTP response. In this example, a custom header 'X-Custom-Header' with the value 'ModifiedHeader' is added to the response.
const http = require('http');
const respModifier = require('resp-modifier');
const server = http.createServer((req, res) => {
const modifier = respModifier({
modify: (req, res, body) => {
res.setHeader('X-Custom-Header', 'ModifiedHeader');
return body;
}
});
modifier(req, res, () => {
res.end('<html><head><title>Test</title></head><body></body></html>');
});
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
The http-proxy-middleware package allows you to create a proxy server that can intercept and modify HTTP requests and responses. It is more versatile than resp-modifier as it supports a wide range of proxy configurations and middleware options.
The express-http-proxy package is used to proxy requests in an Express application. It allows you to intercept and modify requests and responses, similar to resp-modifier, but is specifically designed to work with Express.js.
The node-http-proxy package is a full-featured HTTP proxy for Node.js. It allows you to intercept and modify HTTP requests and responses, and offers more advanced features such as load balancing and WebSocket support.
All the good parts from connect-livereload without the livereload specific bits & with multiple replacements added.
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint and test your code using Gulp.
(Nothing yet)
Copyright (c) 2013 Shane Osbourne Licensed under the MIT license.
FAQs
Middleware for modifying a HTML response
The npm package resp-modifier receives a total of 548,297 weekly downloads. As such, resp-modifier popularity was classified as popular.
We found that resp-modifier demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.