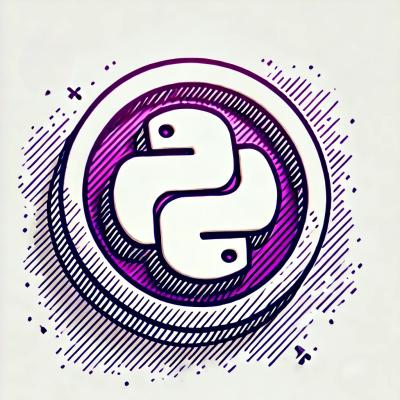
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The rfc4648 npm package provides utilities for encoding and decoding data in Base16, Base32, and Base64 formats, adhering to the specifications outlined in RFC 4648.
Base16 Encoding
This feature allows you to encode data into Base16 (hexadecimal) format. The code sample demonstrates encoding a Uint8Array into a Base16 string.
const rfc4648 = require('rfc4648');
const data = new Uint8Array([72, 101, 108, 108, 111]);
const encoded = rfc4648.base16.stringify(data);
console.log(encoded); // Outputs: 48656C6C6F
Base16 Decoding
This feature allows you to decode Base16 (hexadecimal) strings back into their original data format. The code sample demonstrates decoding a Base16 string into a Uint8Array.
const rfc4648 = require('rfc4648');
const encoded = '48656C6C6F';
const decoded = rfc4648.base16.parse(encoded);
console.log(decoded); // Outputs: Uint8Array(5) [ 72, 101, 108, 108, 111 ]
Base32 Encoding
This feature allows you to encode data into Base32 format. The code sample demonstrates encoding a Uint8Array into a Base32 string.
const rfc4648 = require('rfc4648');
const data = new Uint8Array([72, 101, 108, 108, 111]);
const encoded = rfc4648.base32.stringify(data);
console.log(encoded); // Outputs: JBSWY3DP
Base32 Decoding
This feature allows you to decode Base32 strings back into their original data format. The code sample demonstrates decoding a Base32 string into a Uint8Array.
const rfc4648 = require('rfc4648');
const encoded = 'JBSWY3DP';
const decoded = rfc4648.base32.parse(encoded);
console.log(decoded); // Outputs: Uint8Array(5) [ 72, 101, 108, 108, 111 ]
Base64 Encoding
This feature allows you to encode data into Base64 format. The code sample demonstrates encoding a Uint8Array into a Base64 string.
const rfc4648 = require('rfc4648');
const data = new Uint8Array([72, 101, 108, 108, 111]);
const encoded = rfc4648.base64.stringify(data);
console.log(encoded); // Outputs: SGVsbG8=
Base64 Decoding
This feature allows you to decode Base64 strings back into their original data format. The code sample demonstrates decoding a Base64 string into a Uint8Array.
const rfc4648 = require('rfc4648');
const encoded = 'SGVsbG8=';
const decoded = rfc4648.base64.parse(encoded);
console.log(decoded); // Outputs: Uint8Array(5) [ 72, 101, 108, 108, 111 ]
The base-x package provides utilities for encoding and decoding data in various base formats, including Base16, Base32, and Base64. It is highly customizable and allows for defining custom alphabets for encoding. Compared to rfc4648, base-x offers more flexibility in terms of custom base encoding but may require more configuration for standard use cases.
The base64-js package focuses specifically on Base64 encoding and decoding. It is lightweight and optimized for performance. Compared to rfc4648, base64-js is more specialized and may be a better choice if you only need Base64 functionality without the additional Base16 and Base32 support.
The js-base64 package provides Base64 encoding and decoding utilities with a simple API. It is widely used and well-documented. Compared to rfc4648, js-base64 is more user-friendly and may be easier to integrate for projects that only require Base64 encoding and decoding.
This library implements encoding and decoding for the data formats specified in rfc4648:
Each encoding has a simple API inspired by Javascript's built-in JSON
object:
import { base32 } from 'rfc4648'
base32.stringify([42, 121, 160]) // -> 'FJ42A==='
base32.parse('FJ42A===') // -> Uint8Array([42, 121, 160])
The library has tree-shaking support, so tools like rollup.js or Webpack 2+ can automatically trim away any encodings you don't use.
The library provides the following top-level modules:
base64
base64url
base32
base32hex
base16
codec
Each module exports a parse
and stringify
function.
Each stringify
function takes array-like object of bytes and returns a string.
If you pass the option { pad: false }
in the second parameter, the encoder will not output padding characters (=
).
Each parse
function takes a string and returns a Uint8Array
of bytes. If you would like a different return type, such as plain Array
or a Node.js Buffer
, pass its constructor in the second argument:
base64.parse('AOk=', { out: Array })
base64.parse('AOk=', { out: Buffer.allocUnsafe })
The constructor will be called with new
, and should accept a single integer for the output length, in bytes.
If you pass the option { loose: true }
in the second parameter, the parser will not validate padding characters (=
):
base64.parse('AOk', { loose: true }) // No error
The base32 codec will also fix common typo characters in loose mode:
base64.parse('He1l0==', { loose: true }) // Auto-corrects as 'HELLO==='
To define your own encodings, use the codec
module:
const codec = require('rfc4648').codec
const myEncoding = {
chars: '01234567',
bits: 3
}
codec.stringify([220, 10], myEncoding) // '670050=='
codec.parse('670050', myEncoding, { loose: true }) // [ 220, 10 ]
The encoding
structure should have two members, a chars
member giving the alphabet and a bits
member giving the bits per character. The codec.parse
function will extend this with a third member, codes
, the first time it's called. The codes
member is a lookup table mapping from characters back to numbers.
FAQs
Encoding and decoding for base64, base32, base16, and friends
The npm package rfc4648 receives a total of 943,656 weekly downloads. As such, rfc4648 popularity was classified as popular.
We found that rfc4648 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.