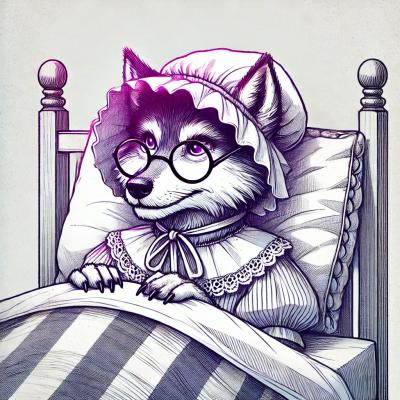
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The ripemd160 npm package is a JavaScript implementation of the RIPEMD-160 cryptographic hash function. It allows users to generate 160-bit hash values from input data. This hash function is commonly used for integrity checks, digital signatures, and other cryptographic operations.
Hashing Data
This feature allows users to create a RIPEMD-160 hash of any given string or buffer. The example code demonstrates how to hash the string 'hello world' and output the result in hexadecimal format.
"use strict";\nconst ripemd160 = require('ripemd160');\nconst hash = new ripemd160().update('hello world').digest('hex');\nconsole.log(hash); // Outputs the RIPEMD-160 hash of 'hello world' in hexadecimal format
The sha1 package is another npm package that provides a JavaScript implementation of the SHA-1 hash function. SHA-1 is also a cryptographic hash function but produces a 160-bit hash value, similar in length to RIPEMD-160. However, SHA-1 is considered less secure than RIPEMD-160 due to vulnerabilities that have been discovered over time.
The sha256 package is used for computing SHA-256 hashes. SHA-256 is part of the SHA-2 family of cryptographic hash functions and produces a 256-bit hash. It is more secure than both RIPEMD-160 and SHA-1, but it generates a longer hash value.
The md5 package is a JavaScript implementation of the MD5 hash function. MD5 produces a 128-bit hash value and is widely used for checksums and fingerprints. However, MD5 is considered cryptographically broken and unsuitable for further use due to its vulnerabilities to collision attacks.
JavaScript component to compute the RIPEMD-160 hash of strings or bytes. This hash is commonly used in crypto currencies like Bitcoin.
npm install --save ripemd160
input
should be either a string
, Buffer
, or an Array
. It returns a Buffer
.
example 1:
var ripemd16 = require('ripemd160')
var data = 'hello'
var result = ripemd160(data)
console.log(result.toString('hex'))
// => 108f07b8382412612c048d07d13f814118445acd
** example 2**:
var ripemd16 = require('ripemd160')
var data = new Buffer('hello', 'utf8')
var result = ripemd160(data)
console.log(result.toString('hex'))
// => 108f07b8382412612c048d07d13f814118445acd
If you're not familiar with the Node.js ecosystem, type Buffer
is a common way that a developer can pass around
binary data. Buffer
also exists in the Browserify environment. Converting to and from Buffers is very easy.
// from string
var buf = new Buffer('some string', 'utf8')
// from hex string
var buf = new Buffer('3f5a4c22', 'hex')
// from array
var buf = new Buffer([1, 2, 3, 4])
// to string
var str = buf.toString('utf8')
// to hex string
var hex = buf.toString('hex')
// to array
var arr = [].slice.call(buf)
npm install --development
npm run test
Testing in the browser uses the excellent Mochify. Mochify can use either PhantomJS
or an actual browser. You must have Selenium installed if you want to use an actual browser. The easiest way is to
npm install -g start-selenium
and then run start-selenium
.
Then run:
npm run browser-test
Licensed: BSD3-Clause
FAQs
Compute ripemd160 of bytes or strings.
The npm package ripemd160 receives a total of 3,206,858 weekly downloads. As such, ripemd160 popularity was classified as popular.
We found that ripemd160 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.