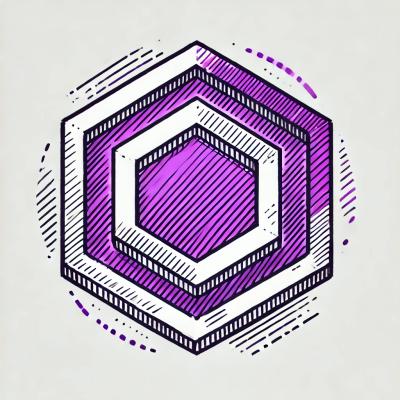
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
A package that provides functions for performing actions on roblox, mostly for use with their HttpService feature.
Roblox-js is a node module that provides functions for performing actions on roblox, mostly for use with their HttpService feature.
This is mostly a collection of functions to perform actions for groups, though others are included. The list of main functions is in the contents section, they all have detailed documentation.
Many of the functions use caches in order to server requests faster. Cache time can be changed in settings.json. Cached items include XCSRF tokens and group roles: unless you change your group roles often the default cache settings should be fine. The cache works by saving request responses for a set amount of time (of course), but will refresh immediately if the item has expired and, for specific things, serve the cached item but then refresh silently even if the cached item has not expired.
To use this with HttpService simply set up API's on your node server for accessing the functions, this module does not provide examples or support for doing that specifically.
Simply install with npm: npm install roblox-js
, no need to download anything manually.
ROBLOX user sessions are stored in a CookieJar
, which can be created like so:
var request = require('request');
var jar = request.jar();
Be default, however, there is a single global cookie jar stored in the module which will automatically be used if you don't specify a custom jar. You can get the global cookie jar with the getJar
function and set a new one with setJar
. There is a custom property of the CookieJar user
which is an object containing the id
and name
of the logged in player retrieved during the login.
The login function populates the cookie jar with the users cookies, including their .ROBLOSECURITY
(session), if successful and any functions that perform non-guest actions need a cookie jar to do so. If you are only using this module for a single group with one promotion user I recommend simply using the default global cookie jar.
Be aware that you must set something to refresh this token every once in a while: otherwise it will expire. Logging in every server restart and making a login interval of 1 day should be enough.
Also remember to check the scripts in the examples and tests folder to see the module in action.
Function usage is below.
All functions have alternate forms, arguments are either passed:
The options object has all the arguments listed are manually named.
For example, you could do:
login('shedletsky','hunter2',jar, function() {
console.log('It worked!');
});
or
var options = {
username: 'shedletsky',
password: 'hunter2',
jar: jar,
success: function() {
console.log('It worked!');
}
}
login(options);
Note that raw functions (required individually) do not support alternate forms. Furthermore, their arguments may not be the same or be in the same order: to find them you have to go into the source and check for yourself.
Success, failure, and always callbacks are executed when the goal of the function: succeeds, fails, or runs at all, respectively.
Cookie jars are all optional, if one isn't specified the function will automatically use the default global cookie jar.
Changes the role of target
(UserID) in group
to roleset
or returns a general error if unsuccessful. Token is the X-CSRF-TOKEN and should only be included if you intend to manually handle them (normally they are automatically retrieved every request).
options [object]:
error [string]
Accepts or denies username
's join request in group
. Token is the X-CSRF-TOKEN and should only be included if you intend to manually handle them (normally they are automatically retrieved every request).
options [object]:
error [string]
Exiles target
in group
and does not return an error if the action was unsuccessful. Token is the X-CSRF-TOKEN and should only be included if you intend to manually handle them (normally they are automatically retrieved every request).
options [object]:
getRolesetInGroupWithJar
will be used.error [string]
Message recipient
with the message body
and subject subject
and returns a detailed error if unsuccessful. Token is the X-CSRF-TOKEN and should only be included if you intend to manually handle them (normally they are automatically retrieved every request).
options [object]:
error [string]
Shouts message
in group
and returns a general error if unsuccessful.
options [object]:
error [string]
Posts message
on group
wall and returns a general error if unsuccessful.
options [object]:
error [string]
Logs in with username
and password
and puts the new cookie into jar
(or the default global jar if unspecified) or returns a detailed error if unsuccessful.
options [object]:
error [string]
Sends the X-CSRF-TOKEN used by the URL to callback
. This only needs to be used if you want to custom handle tokens, normally it is handled automatically.
options [object]:
token [string]
error [string]
Returns role information of a group in the form [{"ID":number,"Name":"string","Rank":number},{"ID":number,"Name":"string","Rank":number}]
. To best used with setRank
.
options [object]:
roles [object]
error [string]
Returns the UserId
that the logged in user had. This is simply a saved ID and is not an accurate check if the user is still logged in.
options [object]:
Gets the current user from the ROBLOX website and feeds option
or all options if successful, otherwise returns detailed error.
options [object]:
UserID
, UserName
, RobuxBalance
, TicketsBalance
, ThumbnailUrl
, IsAnyBuildersClubMember
option [string]
or all options [object]error [string]
Gets the rank of player
in group group
.
options [object]:
rank [number]
error [string]
Gets the roleset ID of player
in group group
.
options [object]:
roleset [number]
error [string]
Gets the roleset ID of player logged into jar
in group group
.
options [object]:
roleset [number]
error [string]
Returns the .ROBLOSECURITY cookie extracted from jar
if it exists.
options [object]:
Returns the global cookie jar in use by the module.
Sets the global cookie jar for the module to use.
Returns verification inputs on the page with the names in find - or all inputs if not provided. Typically used for ROBLOX requests working with ASP.NET.
options [object]:
Short for getInputs(html,['__VIEWSTATE','__VIEWSTATEGENERATOR','__EVENTVALIDATION]')
. Typically used for ROBLOX requests working with ASP.NET.
options [object]:
FAQs
A node module that provides an interface for performing actions on ROBLOX, mostly for use with their HttpService feature.
The npm package roblox-js receives a total of 57 weekly downloads. As such, roblox-js popularity was classified as not popular.
We found that roblox-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.