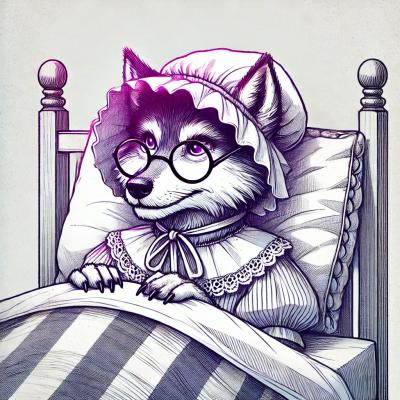
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Control Raspberry Pi GPIO pins with node.js
See this guide on how to get node.js running on Raspberry Pi.
This module can be installed with npm:
npm install rpi-gpio
Please see the examples below. Make make sure you are running as root or with sudo, else the Raspberry Pi will not let you output to the GPIO. All of the functions relating to the pins within this module are asynchronous, so where necessary - for example in reading the value of a pin - a callback must be provided.
Please note that there are two different ways to reference a channel; either using the Raspberry Pi or the BCM/SoC naming schema (sadly, neither of which match the physical pins!). This module supports both schemas, with Raspberry Pi being the default. Please see this page for more details.
var gpio = require('rpi-gpio');
gpio.setup(7, gpio.DIR_IN, readInput);
function readInput() {
gpio.read(7, function(err, value) {
console.log('The value is ' + value);
});
}
The GPIO module inherits from EventEmitter
so any of the EventEmitter functions can be used. The example below shows how to listen for a change in value to a channel.
var gpio = require('rpi-gpio');
gpio.on('change', function(channel, value) {
console.log('Channel ' + channel + ' value is now ' + value);
});
gpio.setup(7, gpio.DIR_IN);
var gpio = require('rpi-gpio');
gpio.setup(7, gpio.DIR_OUT, write);
function write() {
gpio.write(7, true, function(err) {
if (err) throw err;
console.log('Written to pin');
});
}
This will close any pins that were opened by the module.
var gpio = require('../rpi-gpio');
gpio.on('export', function(channel) {
console.log('Channel set: ' + channel);
});
gpio.setup(7, gpio.DIR_OUT);
gpio.setup(15, gpio.DIR_OUT);
gpio.setup(16, gpio.DIR_OUT, pause);
function pause() {
setTimeout(closePins, 2000);
}
function closePins() {
gpio.destroy(function() {
console.log('All pins unexported');
return process.exit(0);
});
}
This example shows how to set up a channel for output mode. After it is set up, it executes a callback which in turn calls another, causing the voltage to alternate up and down three times.
var gpio = require('rpi-gpio');
var pin = 7,
delay = 2000,
count = 0,
max = 3;
gpio.on('change', function(channel, value) {
console.log('Channel ' + channel + ' value is now ' + value);
});
gpio.setup(pin, gpio.DIR_OUT, on);
function on() {
if (count >= max) {
gpio.destroy(function() {
console.log('Closed pins, now exit');
return process.exit(0);
});
return;
}
setTimeout(function() {
gpio.write(pin, 1, off);
count += 1;
}, delay);
}
function off() {
setTimeout(function() {
gpio.write(pin, 0, on);
}, delay);
}
Due to the asynchronous nature of this module, using an asynchronous flow control module can help to simplify development. This example uses async.js to turn pins on and off in series.
var gpio = require('rpi-gpio');
var async = require('async');
gpio.on('change', function(channel, value) {
console.log('Channel ' + channel + ' value is now ' + value);
});
async.parallel([
function(callback) {
gpio.setup(7, gpio.DIR_OUT, callback)
},
function(callback) {
gpio.setup(15, gpio.DIR_OUT, callback)
},
function(callback) {
gpio.setup(16, gpio.DIR_OUT, callback)
},
], function(err, results) {
console.log('Pins set up');
write();
});
function write() {
async.series([
function(callback) {
delayedWrite(7, true, callback);
},
function(callback) {
delayedWrite(15, true, callback);
},
function(callback) {
delayedWrite(16, true, callback);
},
function(callback) {
delayedWrite(7, false, callback);
},
function(callback) {
delayedWrite(15, false, callback);
},
function(callback) {
delayedWrite(16, false, callback);
},
], function(err, results) {
console.log('Writes complete, pause then unexport pins');
setTimeout(function() {
gpio.destroy(function() {
console.log('Closed pins, now exit');
return process.exit(0);
});
}, 500);
});
};
function delayedWrite(pin, value, callback) {
setTimeout(function() {
gpio.write(pin, value, callback);
}, 500);
}
FAQs
Control Raspberry Pi GPIO pins with node.js
The npm package rpi-gpio receives a total of 221 weekly downloads. As such, rpi-gpio popularity was classified as not popular.
We found that rpi-gpio demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.