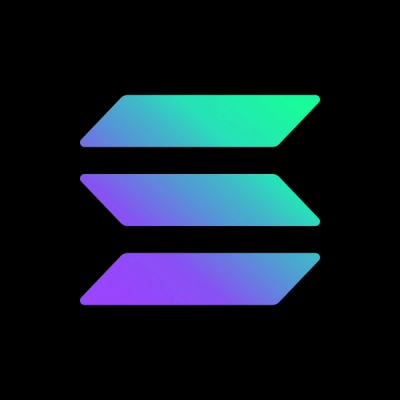
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
rxjs-transducer
Advanced tools
Transducer implementation leveraging RxJS operators to map, filter, reduce, take, skip on potentially infinite sequences
A transducer implementation using the excellent and well known operators from RxJS. The benefits are:
filter().map().Math.max()
on an array with 1,000,000 items is roughly three times as fast with the transducer as with normal array operations.map
, filter
, reduce
, skip
, take
, takeWhile
, and many othersnpm install rxjs-transducer --save
import { transducer } from 'rxjs-transducer';
import { map, filter, reduce, skip, take } from 'rxjs/operators';
const source = ['a', 'ab', 'abc', 'abcd', 'abcde'];
// Works as standard array map and filter, but faster (only one iteration)
const result = transducer(source)(
map(word => word.toUpperCase()),
filter(word => word.length > 2)
);
// result -> ['ABC', 'ABCD', 'ABCDE']
// Note that results is always an array, even if the final operator
// only produces a single result
const result = transducer(source)(
map(word => word.toUpperCase()),
filter(word => word.length > 2),
reduce((acc, s) => `${acc}-${s}`)
);
// result -> ['ABC-ABCD-ABCDE']
// Works with infinte sequences too
const result = transducer(integers())(
map(i => i * 2),
filter(i => i % 10 === 0),
skip(10),
take(5)
);
// result -> [100, 110, 120, 130, 140]
// Infinite sequence of integers from zero -> infinite
function* integers() {
let i = 0;
while (true) {
yield i++;
}
}
const { map, filter, reduce, take, skip } = require('rxjs/operators');
const transducer = require('rxjs-transducer');
const source = ['a', 'ab', 'abc', 'abcd', 'abcde'];
const result = transducer(source)(
map(word => word.toUpperCase()),
filter(word => word.length > 2)
);
// result -> ['ABC', 'ABCD', 'ABCDE']
npm run test
FAQs
Transducer implementation leveraging RxJS operators to map, filter, reduce, take, skip on potentially infinite sequences
The npm package rxjs-transducer receives a total of 14 weekly downloads. As such, rxjs-transducer popularity was classified as not popular.
We found that rxjs-transducer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.