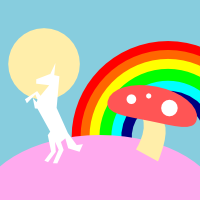
sanctuary-maybe
The Maybe type represents optional values: a value of type Maybe a
is
either Nothing (the empty value) or a Just whose value is of type a
.
Maybe a
satisfies the following Fantasy Land specifications:
> const Useless = require ('sanctuary-useless')
> const isTypeClass = x =>
. type (x) === 'sanctuary-type-classes/TypeClass@1'
> S.map (k => k + ' '.repeat (16 - k.length) +
. (Z[k].test (Just (Useless)) ? '\u2705 ' :
. Z[k].test (Nothing) ? '\u2705 * ' :
. '\u274C '))
. (S.keys (S.unchecked.filter (isTypeClass) (Z)))
[ 'Setoid ✅ * ',
. 'Ord ✅ * ',
. 'Semigroupoid ❌ ',
. 'Category ❌ ',
. 'Semigroup ✅ * ',
. 'Monoid ✅ * ',
. 'Group ❌ ',
. 'Filterable ✅ ',
. 'Functor ✅ ',
. 'Bifunctor ❌ ',
. 'Profunctor ❌ ',
. 'Apply ✅ ',
. 'Applicative ✅ ',
. 'Chain ✅ ',
. 'ChainRec ✅ ',
. 'Monad ✅ ',
. 'Alt ✅ ',
. 'Plus ✅ ',
. 'Alternative ✅ ',
. 'Foldable ✅ ',
. 'Traversable ✅ ',
. 'Extend ✅ ',
. 'Comonad ❌ ',
. 'Contravariant ❌ ' ]
Maybe type representative.
The empty value of type Maybe a
.
> Nothing
Nothing
Constructs a value of type Maybe a
from a value of type a
.
> Just (42)
Just (42)
empty (Maybe)
is equivalent to Nothing
> S.empty (Maybe)
Nothing
of (Maybe) (x)
is equivalent to Just (x)
> S.of (Maybe) (42)
Just (42)
> Z.chainRec (
. Maybe,
. (next, done, x) =>
. x <= 1 ? Nothing : Just (x >= 1000 ? done (x) : next (x * x)),
. 1
. )
Nothing
> Z.chainRec (
. Maybe,
. (next, done, x) =>
. x <= 1 ? Nothing : Just (x >= 1000 ? done (x) : next (x * x)),
. 2
. )
Just (65536)
zero (Maybe)
is equivalent to Nothing
> S.zero (Maybe)
Nothing
show (Nothing)
is equivalent to 'Nothing'
show (Just (x))
is equivalent to 'Just (' + show (x) + ')'
> show (Nothing)
'Nothing'
> show (Just (['foo', 'bar', 'baz']))
'Just (["foo", "bar", "baz"])'
Nothing
is equal to Nothing
Just (x)
is equal to Just (y)
iff x
is equal to y
according to Z.equals
Nothing
is never equal to Just (x)
> S.equals (Nothing) (Nothing)
true
> S.equals (Just ([1, 2, 3])) (Just ([1, 2, 3]))
true
> S.equals (Just ([1, 2, 3])) (Just ([3, 2, 1]))
false
> S.equals (Just ([1, 2, 3])) (Nothing)
false
Nothing
is (less than or) equal to Nothing
Just (x)
is less than or equal to Just (y)
iff x
is less
than or equal to y
according to Z.lte
Nothing
is always less than Just (x)
> S.filter (S.lte (Nothing)) ([Nothing, Just (0), Just (1), Just (2)])
[Nothing]
> S.filter (S.lte (Just (1))) ([Nothing, Just (0), Just (1), Just (2)])
[Nothing, Just (0), Just (1)]
concat (Nothing) (Nothing)
is equivalent to Nothing
concat (Just (x)) (Just (y))
is equivalent to
Just (concat (x) (y))
concat (Nothing) (Just (x))
is equivalent to Just (x)
concat (Just (x)) (Nothing)
is equivalent to Just (x)
> S.concat (Nothing) (Nothing)
Nothing
> S.concat (Just ([1, 2, 3])) (Just ([4, 5, 6]))
Just ([1, 2, 3, 4, 5, 6])
> S.concat (Nothing) (Just ([1, 2, 3]))
Just ([1, 2, 3])
> S.concat (Just ([1, 2, 3])) (Nothing)
Just ([1, 2, 3])
filter (p) (Nothing)
is equivalent to Nothing
filter (p) (Just (x))
is equivalent to p (x) ? Just (x) : Nothing
> S.filter (isFinite) (Nothing)
Nothing
> S.filter (isFinite) (Just (Infinity))
Nothing
> S.filter (isFinite) (Just (Number.MAX_SAFE_INTEGER))
Just (9007199254740991)
map (f) (Nothing)
is equivalent to Nothing
map (f) (Just (x))
is equivalent to Just (f (x))
> S.map (Math.sqrt) (Nothing)
Nothing
> S.map (Math.sqrt) (Just (9))
Just (3)
ap (Nothing) (Nothing)
is equivalent to Nothing
ap (Nothing) (Just (x))
is equivalent to Nothing
ap (Just (f)) (Nothing)
is equivalent to Nothing
ap (Just (f)) (Just (x))
is equivalent to Just (f (x))
> S.ap (Nothing) (Nothing)
Nothing
> S.ap (Nothing) (Just (9))
Nothing
> S.ap (Just (Math.sqrt)) (Nothing)
Nothing
> S.ap (Just (Math.sqrt)) (Just (9))
Just (3)
chain (f) (Nothing)
is equivalent to Nothing
chain (f) (Just (x))
is equivalent to f (x)
> const head = xs => xs.length === 0 ? Nothing : Just (xs[0])
> S.chain (head) (Nothing)
Nothing
> S.chain (head) (Just ([]))
Nothing
> S.chain (head) (Just (['foo', 'bar', 'baz']))
Just ('foo')
alt (Nothing) (Nothing)
is equivalent to Nothing
alt (Just (x)) (Nothing)
is equivalent to Just (x)
alt (Nothing) (Just (x))
is equivalent to Just (x)
alt (Just (y)) (Just (x))
is equivalent to Just (x)
> S.alt (Nothing) (Nothing)
Nothing
> S.alt (Just (1)) (Nothing)
Just (1)
> S.alt (Nothing) (Just (2))
Just (2)
> S.alt (Just (4)) (Just (3))
Just (3)
reduce (f) (x) (Nothing)
is equivalent to x
reduce (f) (x) (Just (y))
is equivalent to f (x) (y)
> S.reduce (S.concat) ('abc') (Nothing)
'abc'
> S.reduce (S.concat) ('abc') (Just ('xyz'))
'abcxyz'
traverse (A) (f) (Nothing)
is equivalent to of (A) (Nothing)
traverse (A) (f) (Just (x))
is equivalent to map (Just) (f (x))
> S.traverse (Array) (S.words) (Nothing)
[Nothing]
> S.traverse (Array) (S.words) (Just ('foo bar baz'))
[Just ('foo'), Just ('bar'), Just ('baz')]
extend (f) (Nothing)
is equivalent to Nothing
extend (f) (Just (x))
is equivalent to Just (f (Just (x)))
> S.extend (S.reduce (S.add) (1)) (Nothing)
Nothing
> S.extend (S.reduce (S.add) (1)) (Just (99))
Just (100)