What is sanity?
Sanity is a platform for structured content that comes with an open-source editing environment called Sanity Studio. It allows you to build and manage content with a flexible and customizable interface.
What are sanity's main functionalities?
Content Modeling
Sanity allows you to define your content models using JavaScript objects. This example shows a simple schema for a blog post with a title and body.
const schema = {
name: 'blogPost',
type: 'document',
fields: [
{ name: 'title', type: 'string' },
{ name: 'body', type: 'text' }
]
};
export default schema;
Real-time Collaboration
Sanity supports real-time collaboration, allowing multiple users to work on the same document simultaneously. This example demonstrates how to use the `useDocumentOperation` hook to update a document in real-time.
import { useDocumentOperation } from '@sanity/react-hooks';
function MyComponent({ id }) {
const { patch, commit } = useDocumentOperation(id, 'myDocumentType');
const handleChange = () => {
patch.execute([{ set: { title: 'New Title' } }]);
commit.execute();
};
return <button onClick={handleChange}>Change Title</button>;
}
Custom Input Components
Sanity allows you to create custom input components for your content models. This example shows how to create a simple custom input component using React.
import React from 'react';
import { FormField } from '@sanity/base/components';
const MyCustomInput = React.forwardRef((props, ref) => {
return (
<FormField label='My Custom Input'>
<input ref={ref} type='text' {...props} />
</FormField>
);
});
export default MyCustomInput;
Other packages similar to sanity
contentful
Contentful is a headless CMS that provides a similar set of features for content modeling and management. It offers a web-based interface for content creators and a robust API for developers. Compared to Sanity, Contentful has a more polished UI but may be less flexible in terms of customization.
strapi
Strapi is an open-source headless CMS that allows you to create and manage content with a customizable API. It offers a more developer-friendly experience with its plugin system and is highly customizable. Strapi is similar to Sanity in terms of flexibility but has a different approach to content modeling and API generation.
prismic-javascript
Prismic is a headless CMS that focuses on providing a user-friendly interface for content creators and a powerful API for developers. It offers features like content versioning and scheduling. Compared to Sanity, Prismic is more focused on ease of use and may have fewer customization options.
Sanity
Build powerful production-ready content workspaces
The Sanity Composable Content Cloud lets teams create remarkable digital experiences at scale. Sanity Studio is an open-source, single-page application that is fast to set up and quick to configure. The Studio comes with a complete studio customization framework that lets you tailor the workspace as your needs grow.
Sanity hosts your content in a real-time, hosted data store called Content Lake. Get started with the free plan with generous bandwidth and hosting included and pay-as-you-go for overages.
Quickstart
Initiate a new project by running the following command:
npm create sanity@latest
yarn create sanity@latest
pnpm create sanity@latest
Go here for a step-by-step guide on how to get up and running.
Check out the docs and plugins and start building.
Key Features
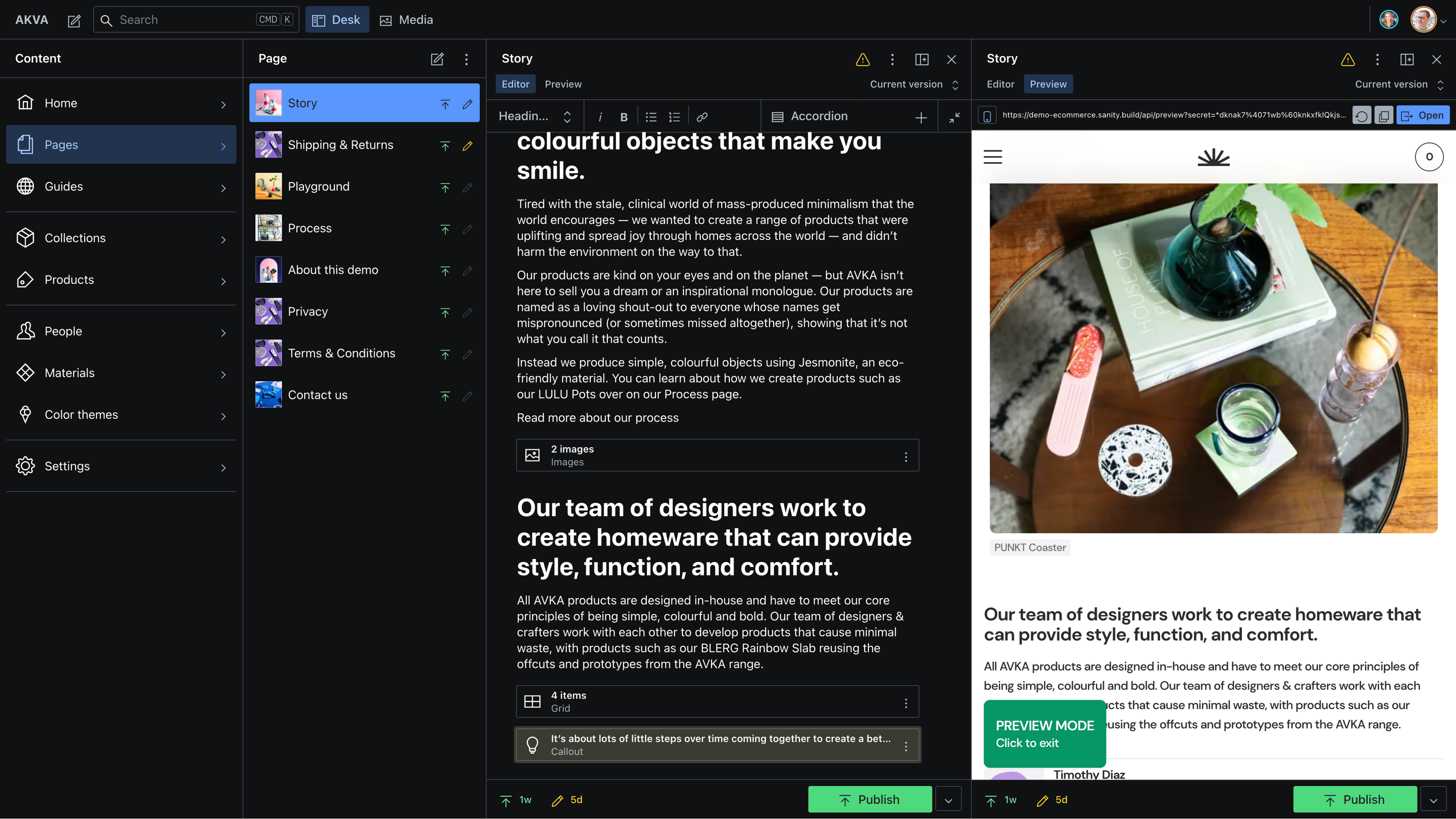
Sanity Studio is an open-source real-time CMS, that you can customize with JavaScript and React.
Stay up to date
Code of Conduct
We aim to be an inclusive, welcoming community for everyone. To make that explicit, we have a code of conduct that applies to communication around the project.
Want to contribute?
Found a bug, or want to contribute code? Pull requests and issues are most welcome. Read our contributing guidelines to learn how.
Help Improve Sanity Studio translations
We're always looking to make Sanity Studio more accessible and user-friendly, and your contributions can make a big difference. Whether you're a seasoned developer or just starting out, helping with translations is a fantastic way to get involved.
If you're fluent in a language other than English, we'd love your help in reviewing and improving translations. Your expertise can greatly enhance the experience for users worldwide.
How to Contribute:
- Visit our sanity-io/locales repository and try out a locale you are fluent in.
- Have ideas for improvements? Submit a PR following the contributing guide.
- See if there are open PRs in languages you are fluent in and help review them.
Interested in playing a bigger role? You can ask to be added as a maintainer to oversee translations for specific languages, where you will be automatically added as a reviewer on PRs that involve your language. See the sanity-io/locales README for more.
Your contributions not only improve Sanity Studio but also bring together a diverse and global community of users. We appreciate every effort, big or small, and we can't wait to see what you bring to the table!
License
Sanity Studio is available under the MIT License