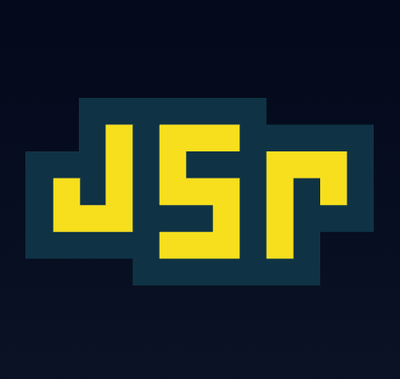
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Satchel is a data store based on the Flux architecture. It is characterized by exposing an observable state that makes view updates painless and efficient.
Satchel is an attempt to synthesize the best of several dataflow patterns typically used to drive a React-based UI. In particular:
There are a number of advantages to using Satchel to maintain your application state. (Each of the frameworks above has some, but not all, of these qualities.)
Install via NPM:
npm install satcheljs --save
In order to use Satchel with React, you'll also need MobX and the MobX React bindings:
npm install mobx --save
npm install mobx-react --save
The following examples assume you're developing in Typescript.
interface MyStoreSchema {
foo: number;
bar: string;
}
var myStore = createStore<MyStoreSchema>(
"mystore",
{
foo: 1,
bar: "baz"
});
Notice the @observer decorator on the component---this is what tells MobX to rerender the component if any of the data it relies on changes.
@observer
class ApplicationComponent extends React.Component<any, any> {
render() {
return (<div>foo is {myStore.foo}</div>);
}
}
let updateFoo =
function updateFoo(newFoo: number) {
myStore.foo = newFoo;
};
updateFoo = action("updateFoo")(updateFoo);
Note that the above is just syntactic sugar for applying an @action decorator. Typescript doesn't support decorators on function expressions yet, but it will in 2.0. At that point the syntax for creating an action will be simply:
let updateFoo =
@action("updateFoo")
function updateFoo(newFoo: number) {
myStore.foo = newFoo;
};
It's just a function:
updateFoo(2);
Often actions will need to do some sort of asynchronous work (such as making a server request) and then update the state based on the result. Since the asynchronous callback happens outside of the context of the original action the callback itself must be an action too. (Again, this syntax will be simplified once Typescript 2.0 is available.)
let updateFooAsync =
function updateFooAsync(newFoo: number) {
// You can modify the state in the original action
myStore.loading = true;
// doSomethingAsync returns a promise
doSomethingAsync().then(
action("doSomethingAsyncCallback")(
() => {
// Modify the state again in the callback
myStore.loading = false;
myStore.foo = newFoo;
}));
};
updateFooAsync = action("updateFooAsync")(updateFooAsync);
FAQs
Store implementation for functional reactive flux.
The npm package satcheljs receives a total of 161 weekly downloads. As such, satcheljs popularity was classified as not popular.
We found that satcheljs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.