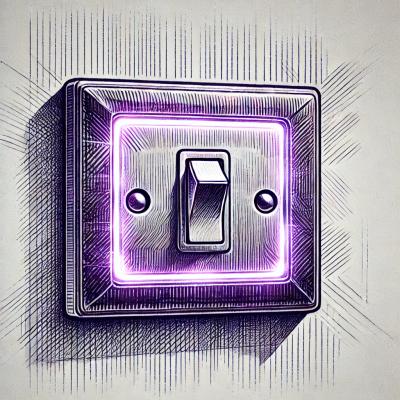
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
scaled-number
Advanced tools
A class for managing large numbers with a decimal scale, useful for web3 development
A class for managing large numbers with a decimal scale, useful for web3 development.
yarn add scaled-number
import { ScaledNumber } from 'scaled-number';
const scaledNumber = ScaledNumber.fromUnscaled(123);
console.log(scaledNumber.mul(10).toString());
import { ScaledNumber } from 'scaled-number';
import { getTokenDecimals, getContract, getPrice } from "helpers";
export interface Pool {
symbol: string;
tvl: ScaledNumber;
apy: ScaledNumber;
}
// Returns some key info about a Pool
export const getPoolInfo = async (poolAddress: string): Pool => {
const pool = getContract(poolAddress);
// Getting Pool info
const [
underlyingAddress,
tvlBN,
apyBN
] = await Promise.all([
pool.underlyingAddress(),
pool.tvl(),
pool.apy(),
]);
// Getting the underlying info
const underlying = getContract(underlyingAddress);
const [
decimals,
symbol
] = await Promise.all([
underlying.decimals(),
underlying.symbol()
]);
// Getting the TVL as a Scaled Number (using the underlying decimals)
const tvl = new ScaledNumber(tvlBN, decimals);
// Getting the APY as a Scaled Number (uses default 18 decimals)
const apy = new ScaledNumber(apyBN);
return {
symbol
tvl,
apy
}
}
// Logs key information about a pool
export const logPoolInfo = async (pool: Pool): void => {
// Getting the price of the underlying
const price = await getPrice(pool.symbol);
console.log(`Underlying Balance: ${pool.tvl.toCryptoString()} ${pool.symbol}`);
// Output: `Underlying Balance: 12,456.87 ETH`
console.log(`TVL: ${pool.tvl.toCompactUsdValue(price)}`);
// Output: `TVL: $13.4m`
console.log(pool.apy.toPercent())
// Output: `24.34%`
}
new ScaledNumber(bigInt: bigint, decimals?: number);
import { ScaledNumber } from 'scaled-number';
const scaledNumber = new ScaledNumber(BigInt(123));
new ScaledNumber(bigNumber: BigNumber, decimals?: number);
import { ScaledNumber } from 'scaled-number';
import { BigNumber } from '@ethersproject/bignumber';
const scaledNumber = new ScaledNumber(BigNumber.from(123));
fromUnscaled(value: number | string = 0, decimals = 18)
import { ScaledNumber } from 'scaled-number';
const scaledNumber = ScaledNumber.fromUnscaled(123, 8);
fromPlain(value: PlainScaledNumber)
import { ScaledNumber } from 'scaled-number';
const scaledNumber = ScaledNumber.fromPlain({
value: '123000000',
decimals: 6,
});
add(other: ScaledNumber)
const one = ScaledNumber.fromUnscaled(1);
const two = ScaledNumber.fromUnscaled(2);
const three = one.add(two);
console.log(three.toString()); // 3
sub(other: ScaledNumber)
const three = ScaledNumber.fromUnscaled(3);
const two = ScaledNumber.fromUnscaled(2);
const one = three.sub(two);
console.log(one.toString()); // 1
max(other: ScaledNumber)
const three = ScaledNumber.fromUnscaled(3);
const two = ScaledNumber.fromUnscaled(2);
const max = three.max(two);
console.log(max.toString()); // 3
min(other: ScaledNumber)
const three = ScaledNumber.fromUnscaled(3);
const two = ScaledNumber.fromUnscaled(2);
const min = three.min(two);
console.log(min.toString()); // 2
mul(value: number | string | ScaledNumber)
const three = ScaledNumber.fromUnscaled(3);
const six = three.mul(2);
console.log(six.toString()); // 6
div(value: number | string | ScaledNumber)
const six = ScaledNumber.fromUnscaled(3);
const three = three.div(2);
console.log(three.toString()); // 3
toString(): string
const sn = ScaledNumber.fromUnscaled(1.234);
console.log(sn.toString()); // 1.234
toNumber(): number
const sn = ScaledNumber.fromUnscaled(1.234);
console.log(sn.toNumber()); // 1.234
toCryptoString(): string
const sn = ScaledNumber.fromUnscaled('12345678.12345678');
console.log(sn.toCryptoString()); // 12,345,678
const sn = ScaledNumber.fromUnscaled('12.12345678');
console.log(sn.toCryptoString()); // 12.123
const sn = ScaledNumber.fromUnscaled('0.0000000123');
console.log(sn.toCryptoString()); // 0.0000000123
toCryptoString(): string
const sn = ScaledNumber.fromUnscaled('12345678.12345678');
console.log(sn.toCryptoString()); // 12,345,678
const sn = ScaledNumber.fromUnscaled('12.12345678');
console.log(sn.toCryptoString()); // 12.123
const sn = ScaledNumber.fromUnscaled('0.0000000123');
console.log(sn.toCryptoString()); // 0.0000000123
toUsdValue(price: number): string
const sn = ScaledNumber.fromUnscaled('12345678.12345678');
console.log(sn.toUsdValue(7)); // $86,419,746.86
toCompactUsdValue(price: number): string
const sn = ScaledNumber.fromUnscaled('12345678.12345678');
console.log(sn.toCompactUsdValue(7)); // $86,4m
toPercent(): string
const sn = ScaledNumber.fromUnscaled('0.12345678');
console.log(sn.toPercent()); // 12.34%
value: bigint;
const sn = ScaledNumber.fromUnscaled('0.123', 5);
console.log(sn.value.toString()); // 12300
decimals: number;
const sn = ScaledNumber.fromUnscaled('0.123', 5);
console.log(sn.decimals); // 5
toPlain(): PlainScaledNumber
const sn = ScaledNumber.fromUnscaled('0.123', 5);
console.log(sn.toPlain()); // { value: "12300", decimals: 5 }
isZero(): boolean
const sn = ScaledNumber.fromUnscaled('0.123', 5);
console.log(sn.isZero()); // false
const sn = ScaledNumber.fromUnscaled();
console.log(sn.isZero()); // true
isNegative(): boolean
const sn = ScaledNumber.fromUnscaled('0.123', 5);
console.log(sn.isNegative()); // false
const sn = ScaledNumber.fromUnscaled('-0.123', 5);
console.log(sn.isNegative()); // true
eq(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(2);
console.log(first.eq(second)); // false
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(1);
console.log(first.eq(second)); // true
gt(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(2);
console.log(first.gt(second)); // false
gte(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(1);
console.log(first.gte(second)); // true
lt(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(2);
console.log(first.lt(second)); // true
lte(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(1);
console.log(first.lte(second)); // true
lte(): boolean
const first = ScaledNumber.fromUnscaled(1);
const second = ScaledNumber.fromUnscaled(1);
console.log(first.lte(second)); // true
FAQs
A class for managing large numbers with a decimal scale, useful for web3 development
The npm package scaled-number receives a total of 3 weekly downloads. As such, scaled-number popularity was classified as not popular.
We found that scaled-number demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.