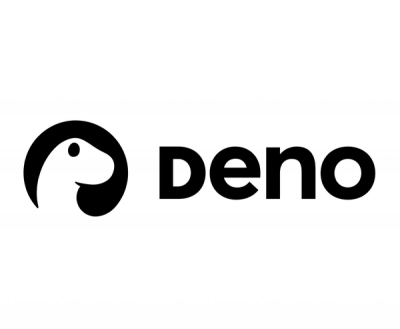
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A Node.js scraper for humans.
$ npm i --save scrape-it
const scrapeIt = require("scrape-it");
function parseStatusCurrent(description){
var status = {};
status.description = description.trim();
if(status.description == 'All Systems Operational'){
status.indicator = "Operational";
status.color = "green";
}
else if(status.description.indexOf('Minor')>-1){
status.indicator = "Not Fully Operational";
status.color = "yellow";
}
else {
status.indicator = "Not Fully Operational";
status.color = "red";
}
return status;
}
scrapeIt("https://status.airbrake.io/", {
status: {
selector: ".page-status span.status",
data: {}
convert: parseStatusCurrent
}
, provider: {
selector: ".powered-by"
, convert: (function (text) {
if(text == "Powered by StatusPage.io"){
return "StatusPage.io";
}
else {
return "Unknown";
}
})
}
, updated_at: {
convert: new Date().toISOString()
}
}).then(status => {
console.log(status);
}).catch(console.log);
scrapeIt(url, opts, cb)
A scraping module for humans.
url
: The page url or request options.opts
: The options passed to scrapeHTML
method.cb
: The callback function.scrapeIt.scrapeHTML($, opts)
Scrapes the data in the provided element.
Cheerio $
: The input element.
Object opts
: An object containing the scraping information.
If you want to scrape a list, you have to use the listItem
selector:
listItem
(String): The list item selector.data
(Object): The fields to include in the list objects:
<fieldName>
(Object|String): The selector or an object containing:
selector
(String): The selector.convert
(Function): An optional function to change the value.how
(Function|String): A function or function name to access the
value.attr
(String): If provided, the value will be taken based on
the attribute name.trim
(Boolean): If false
, the value will not be trimmed
(default: true
).eq
(Number): If provided, it will select the nth element.listItem
(Object): An object, keeping the recursive schema of
the listItem
object. This can be used to create nested lists.Example:
{
articles: {
listItem: ".article"
, data: {
createdAt: {
selector: ".date"
, convert: x => new Date(x)
}
, title: "a.article-title"
, tags: {
listItem: ".tags > span"
}
, content: {
selector: ".article-content"
, how: "html"
}
}
}
}
If you want to collect specific data from the page, just use the same
schema used for the data
field.
Example:
{
title: ".header h1"
, desc: ".header h2"
, avatar: {
selector: ".header img"
, attr: "src"
}
}
Have an idea? Found a bug? See how to contribute.
FAQs
A Node.js scraper for humans.
The npm package scrape-it receives a total of 4,941 weekly downloads. As such, scrape-it popularity was classified as popular.
We found that scrape-it demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.