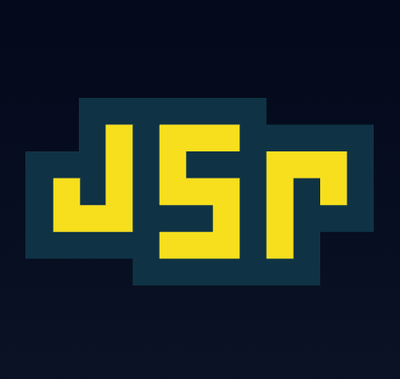
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
scrollreveal
Advanced tools
The simplest method is to copy paste this snippet just before your closing </body>
tag.
<script src="https://cdn.jsdelivr.net/scrollreveal.js/3.0.6/scrollreveal.min.js"></script>
But you can also:
npm install scrollreveal
bower install scrollreveal
The reveal()
method is the primary API, and makes it easy to create and manage various types of animations.
<!-- HTML -->
<div class="foo"> Foo </div>
<div class="bar"> Bar </div>
// JavaScript
window.sr = ScrollReveal();
sr.reveal('.foo');
sr.reveal('.bar');
The ScrollReveal constructor, and it's primary methods all support chaining.
window.sr = ScrollReveal();
sr.reveal('.foo');
sr.reveal('.bar');
// Is the same as...
window.sr = ScrollReveal().reveal('.foo, .bar');
Passing a configuration object to ScrollReveal()
changes the defaults for all reveals, and passing reveal()
a configuration object customizes that reveal set further.
// Changing the defaults
window.sr = ScrollReveal({ reset: true });
// Customizing a reveal set
sr.reveal( '.foo', { duration: 200 } );
// Animation
origin : 'bottom',
distance : '20px',
duration : 500,
delay : 0,
rotate : { x: 0, y: 0, z: 0 },
opacity : 0,
scale : 0.9,
easing : 'cubic-bezier( 0.6, 0.2, 0.1, 1 )',
// Options
container : null,
mobile : true,
reset : false,
useDelay : 'always',
viewFactor : 0.20,
viewOffset : { top: 0, right: 0, bottom: 0, left: 0 },
afterReveal : function( domEl ) {},
afterReset : function( domEl ) {}
key | type | values | notes |
---|---|---|---|
origin | string | 'top' 'right' 'bottom' 'left' | |
distance | string | '20px' '10vw' '5%' | Any valid CSS unit will work. |
duration | number | 500 | Time in milliseconds. |
delay | number | 0 | Time in milliseconds. |
rotate | object /number | { x: 0, y: 0, z: 0 } | Starting angle in degrees. |
opacity | number | 0 | Starting opacity. |
scale | number | 0.9 | Starting scale. |
easing | string | 'ease' 'ease-in' 'ease-out' 'ease-in-out' 'cubic-bezier()' | Any valid CSS easing will work. |
container | node | document.getElementById('foo') | |
mobile | boolean | true / false | Toggle animations on mobile |
reset | boolean | true / false | Elements reveal either once, or reset to reveal each time they are within viewport/container bounds. |
useDelay | string | 'always' 'once' 'onload' | Control when elements use animation delay. |
viewFactor | number | 0.20 | e.g. 20% of an element must be within viewport/container bounds before it reveals. |
viewOffset | object /number | { top: 48, bottom: 24 } | Increase viewport/container bounds in pixels. (See Diagram) |
afterReveal | function | function( domEl ) {} | Fires after reveal animations. |
afterReset | function | function( domEl ) {} | Fires after reset animations. |
reveal()
is equipped to handle calls on the same element, so it's easy to override element configuration.
<div class="foo"> Foo </div>
<div class="foo" id="chocolate"> Chip </div>
var fooReveal = {
delay : 200,
distance : '90px',
easing : 'ease-in-out',
rotate : { z: 10 },
scale : 1.1
};
window.sr = ScrollReveal()
.reveal( '.foo', fooReveal )
.reveal( '#chocolate', { delay: 500, scale: 0.9 } );
The default container is the viewport, but you assign any container to any reveal set.
Tip: ScrollReveal works just as well with horizontally scrolling containers too!
<div id="fooContainer">
<div class="foo"> Foo 1 </div>
<div class="foo"> Foo 2 </div>
<div class="foo"> Foo 3 </div>
</div>
<div id="barContainer">
<div class="bar"> Bar 1 </div>
<div class="bar"> Bar 2 </div>
<div class="bar"> Bar 3 </div>
</div>
var fooContainer = document.getElementById('fooContainer');
var barContainer = document.getElementById('barContainer');
window.sr = ScrollReveal()
.reveal( '.foo', { container: fooContainer } );
.reveal( '.bar', { container: barContainer } );
The sync()
method updates asynchronously loaded content with any existing reveal sets.
Example:
<!-- index.html -->
<div id="container">
<div class="foo">foo</div>
<div class="foo">foo</div>
<div class="foo">foo</div>
</div>
<!-- ajax.html -->
<div class="foo">foo async</div>
<div class="foo">foo async</div>
<div class="foo">foo async</div>
var fooContainer, content, sr, xmlhttp;
fooContainer = document.getElementById('fooContainer');
sr = ScrollReveal();
sr.reveal( '.foo', { container: fooContainer } );
// Setup a new asynchronous request...
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if ( xmlhttp.readyState == XMLHttpRequest.DONE ) {
if ( xmlhttp.status == 200 ) {
// Turn our response into HTML...
var content = document.createElement('div');
content.innerHTML = xmlhttp.responseText;
content = content.childNodes;
// Add each element to the DOM...
for ( var i = 0; i < content.length; i++ ) {
fooContainer.appendChild( content[ i ]);
};
// Finally!
sr.sync();
}
}
}
xmlhttp.open('GET', 'ajax.html', true);
xmlhttp.send();
It’s important that ScrollReveal be called (as close to) last in your page as possible, so that:
Example:
<!DOCTYPE html>
<html>
<body>
<!-- All the things... -->
<script src="js/scrollreveal.min.js"></script>
<script>
window.sr = ScrollReveal();
</script>
</body>
</html>
In most cases, your elements will start at opacity: 0
so they can fade in. However, since JavaScript loads after the page begins rendering, you might see your elements flickering as they begin rendering before being hidden by ScrollReveal's JavaScript.
The ideal solution is to set your reveal elements visibility to hidden in the <head>
of your page, to ensure they render hidden while your JavaScript loads:
Continuing our example from 4.1.
<!DOCTYPE html>
<html>
<head>
<script>
// If JavaScript is enabled, add '.js-enabled' to <html> element
document.documentElement.classList.add('js-enabled');
</script>
<style>
/* Ensure elements load hidden before ScrollReveal runs */
.js-enabled .fooReveal { visibility: hidden; }
</style>
</head>
<body>
<!-- All the things... -->
<script src="js/scrollreveal.min.js"></script>
<script>
window.sr = ScrollReveal();
sr.reveal('.fooReveal');
</script>
</body>
</html>
Note: If you prefer not to put styles in the
<head>
of your page, including this style in your primary stylesheet will still help with element flickering since your CSS will likely load before your JavaScript.
ScrollReveal supports 3d rotation out of the box, but you may want to emphasize the effect by specifying a perspective property on your container.
Continuing our example from 4.2.
<!DOCTYPE html>
<html>
<head>
<script>
document.documentElement.classList.add('js-enabled');
</script>
<style>
.js-enabled .fooReveal { visibility: hidden; }
.fooContainer { perspective: 800px; }
</style>
</head>
<body>
<div class="fooContainer">
<div class="fooReveal"> Foo </div>
<div class="fooReveal"> Foo </div>
<div class="fooReveal"> Foo </div>
</div>
<script src="js/scrollreveal.min.js"></script>
<script>
window.sr = ScrollReveal();
sr.reveal( '.fooReveal', { rotate: {x: 65} } );
</script>
</body>
</html>
Open source under the MIT License. ©2014–2016 Julian Lloyd.
Please search existing issues, before creating a new one; every issue is labeled and attended carefully. If you open a duplicate issue, it will be closed immediately.
If you cannot find your issue/bug in a previous ticket, please include details such as your browser, any other 3rd party JavaScript libraries you are using, and ideally a code sample demonstrating the problem. (Try JSBin)
Feeling inspired? Please contribute! Optimizations, compatibility and bug fixes are greatly preferred over new features, but don’t be shy. One thing sorely missing from ScrollReveal right now is a test suite.
Here are some cool sites using ScrollReveal:
Want to see your page here? Please send me your work (or of others) using ScrollReveal on Twitter (@jlmakes)
ScrollReveal was inspired by the talented Manoela Ilic and her cbpScroller.js.
FAQs
Animate elements as they scroll into view
The npm package scrollreveal receives a total of 16,638 weekly downloads. As such, scrollreveal popularity was classified as popular.
We found that scrollreveal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.