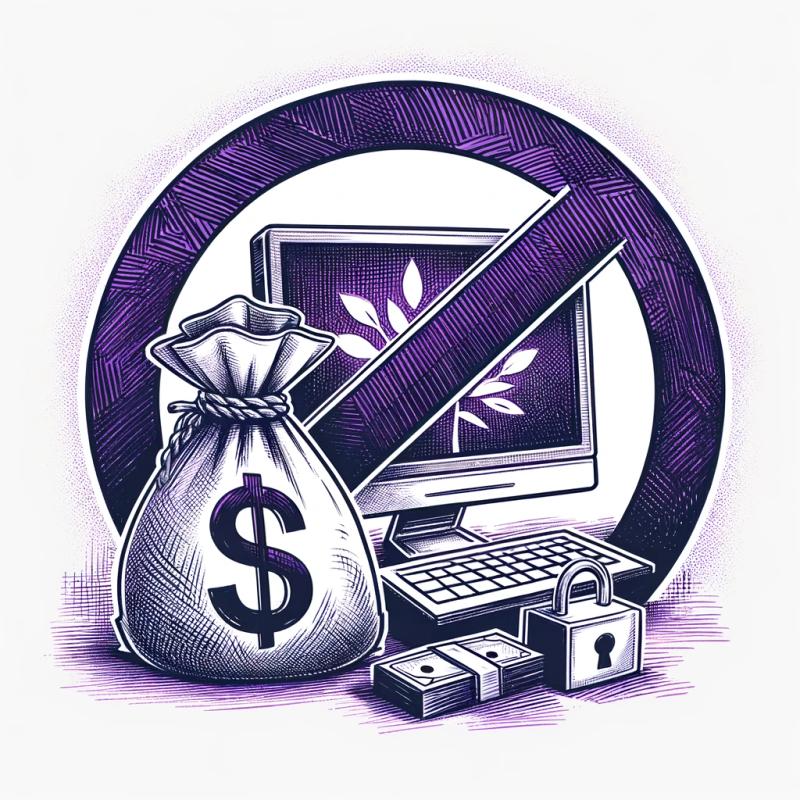
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
selderee
Advanced tools
Package description
The selderee npm package is a tool for processing and transforming selectors. It allows you to parse CSS selectors and then make decisions based on the structure of those selectors. It can be used to filter, modify, or otherwise manipulate selectors programmatically.
Parsing and transforming selectors
This feature allows you to parse CSS selectors and apply transformations to them. The code sample demonstrates how to create a transformer function that processes a selector and returns it unchanged.
const { builder } = require('selderee');
const myTransformer = builder((decisionMap) => {
decisionMap.default(decision => decision.selector);
});
const transformedSelector = myTransformer.transform('.my-class > .my-element');
Custom decision functions
This feature enables you to define custom decision functions for specific types of selectors. In the code sample, a decision function is defined to replace 'div' tags with the class '.replaced-div'.
const { builder } = require('selderee');
const myTransformer = builder((decisionMap) => {
decisionMap.onTag('div', () => '.replaced-div');
decisionMap.default(decision => decision.selector);
});
const transformedSelector = myTransformer.transform('div > .my-element');
postcss-selector-parser is a parser for CSS selectors that allows you to traverse and manipulate selectors. It is similar to selderee in that it provides a way to work with CSS selectors, but it is built on top of PostCSS and is more focused on the parsing and manipulation of selectors within the PostCSS ecosystem.
css-what is a simple CSS selector parser. It can parse selectors into an abstract syntax tree (AST) but does not include the decision map feature that selderee offers. It is more lightweight and less feature-rich compared to selderee.
Readme
Selectors decision tree - pick matching selectors, fast.
The problem statement: there are multiple CSS selectors with attached handlers, and a HTML DOM to process. For each HTML Element a matching handler has to be found and applied.
The naive approach is to walk through the DOM and test each and every selector against each Element. This means O(n*m) complexity.
It is pretty clear though that if we have selectors that share something in common then we can reduce the number of checks.
The main selderee
package offers the tree structure. Working decision functions for specific DOM implementations are built via plugins.
~
), descendants (
) and same column combinators (||
) are also not supported.selderee
vs css-select
css-select - a CSS selector compiler & engine.
Feature | selderee | css-select |
---|---|---|
Support for htmlparser2 DOM AST | plugin | + |
"Compiles" into a function | + | + |
Pick selector(s) for a given Element | + | |
Query Element(s) for a given selector | + |
Package | Version | Folder | Changelog |
---|---|---|---|
selderee | /packages/selderee | changelog | |
@selderee/plugin-htmlparser2 | /packages/plugin-htmlparser2 | changelog |
> npm i selderee @selderee/plugin-htmlparser2
const htmlparser2 = require('htmlparser2');
const util = require('util');
const { DecisionTree, Treeify } = require('selderee');
const { hp2Builder } = require('@selderee/plugin-htmlparser2');
const selectorValuePairs = [
['p', 'A'],
['p.foo[bar]', 'B'],
['p[class~=foo]', 'C'],
['div.foo', 'D'],
['div > p.foo', 'E'],
['div > p', 'F'],
['#baz', 'G']
];
// Make a tree structure from all given selectors.
const selectorsDecisionTree = new DecisionTree(selectorValuePairs);
// `treeify` builder produces a string output for testing and debug purposes.
// `treeify` expects string values attached to each selector.
const prettyTree = selectorsDecisionTree.build(Treeify.treeify);
console.log(prettyTree);
const html = /*html*/`<html><body>
<div><p class="foo qux">second</p></div>
</body></html>`;
const dom = htmlparser2.parseDocument(html);
const element = dom.children[0].children[0].children[1].children[0];
// `hp2Builder` produces a picker that can pick values
// from the selectors tree.
const picker = selectorsDecisionTree.build(hp2Builder);
// Get all matches
const allMatches = picker.pickAll(element);
console.log(util.inspect(allMatches, { breakLength: 50, depth: null }));
// or get the value from the most specific match.
const bestMatch = picker.pick1(element);
console.log(`Best matched value: ${bestMatch}`);
▽
├─◻ Tag name
│ ╟─◇ = p
│ ║ ┠─▣ Attr value: class
│ ║ ┃ ╙─◈ ~= "foo"
│ ║ ┃ ┠─◨ Attr presence: bar
│ ║ ┃ ┃ ┖─◁ #1 [0,2,1] B
│ ║ ┃ ┠─◁ #2 [0,1,1] C
│ ║ ┃ ┖─◉ Push element: >
│ ║ ┃ └─◻ Tag name
│ ║ ┃ ╙─◇ = div
│ ║ ┃ ┖─◁ #4 [0,1,2] E
│ ║ ┠─◁ #0 [0,0,1] A
│ ║ ┖─◉ Push element: >
│ ║ └─◻ Tag name
│ ║ ╙─◇ = div
│ ║ ┖─◁ #5 [0,0,2] F
│ ╙─◇ = div
│ ┖─▣ Attr value: class
│ ╙─◈ ~= "foo"
│ ┖─◁ #3 [0,1,1] D
└─▣ Attr value: id
╙─◈ = "baz"
┖─◁ #6 [1,0,0] G
[ { index: 2, value: 'C', specificity: [ 0, 1, 1 ] },
{ index: 4, value: 'E', specificity: [ 0, 1, 2 ] },
{ index: 0, value: 'A', specificity: [ 0, 0, 1 ] },
{ index: 5, value: 'F', specificity: [ 0, 0, 2 ] } ]
Best matched value: E
Some gotcha: you may notice the check for #baz
has to be performed every time the decision tree is called. If it happens to be p#baz
or div#baz
or even .foo#baz
- it would be much better to write it like this. Deeper, narrower tree means less checks on average. (in case of .foo#baz
the class check might finally outweight the tag name check and rebalance the tree.)
FAQs
Selectors decision tree - choose matching selectors, fast
We found that selderee demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).