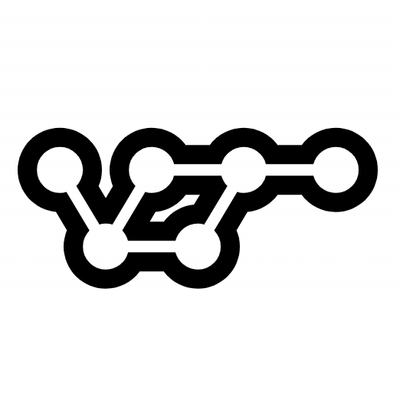
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
select2-aurora
Advanced tools
This library was generated with [Angular CLI](https://github.com/angular/angular-cli) version 11.2.8.
This library was generated with Angular CLI version 11.2.8.
for last version run
npm i select2-aurora@latest
for a special version run
npm i select2-aurora@1.0.0
After install the package, you must add Select2AuroraModule in app.modules.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { Select2AuroraModule } from 'select2-aurora'; // <-- here
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
Select2AuroraModule // <-- here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
We define a model named AuroraSelectModel in select2-aurora. You can prepare your list with this model. First of all import AuroraSelectModel in your component. Then define your list with this model. The AuroraSelectModel has two properties, one is id, and second is label.
import { AuroraSelectModel } from 'select2-aurora';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
countriesList: Array<AuroraSelectModel> = new Array<AuroraSelectModel>();
ngOnInit()
{
this.initOptionList();
}
initOptionList()
{
this.countriesList = new Array<AuroraSelectModel>();
this.countriesList.push(new AuroraSelectModel(1, 'Austria'));
this.countriesList.push(new AuroraSelectModel(2, 'Belgium'));
this.countriesList.push(new AuroraSelectModel(3, 'Finland'));
this.countriesList.push(new AuroraSelectModel(4, 'France'));
this.countriesList.push(new AuroraSelectModel(5, 'Germany'));
this.countriesList.push(new AuroraSelectModel(6, 'Spain'));
this.countriesList.push(new AuroraSelectModel(7, 'Portugal'));
}
}
Then you can define select2-aurora in your template.
<select2-aurora
[optionList]="countriesList"
>
</select2-aurora>
You can use select2-aurora in a form. Here we have an example
import { FormControl, FormGroup, FormBuilder } from '@angular/forms';
import { AuroraSelectModel } from 'select2-aurora';
export class AppComponent implements OnInit {
countriesList: Array<AuroraSelectModel> = new Array<AuroraSelectModel>();
formGroup: FormGroup;
constructor(private formBuilder: FormBuilder)
{}
ngOnInit()
{
this.initOptionList();
this.createForm();
}
createForm()
{
this.formGroup = this.formBuilder.group({
countryFC: new FormControl()
});
}
initOptionList()
{
// as previous example
}
onSaveForm()
{
console.log(this.formGroup.value);
}
}
You can set a default value for form control.
this.formGroup = this.formBuilder.group({
countryFC: new FormControl(2)
});
in template
<form [formGroup]="formGroup" (ngSubmit)="onSaveForm()">
<span>Countries:</span>
<select2-aurora
formControlName="countryFC"
[optionList]="countriesList"
>
</select2-aurora>
<br>
<button type="submit" name="button">Save</button>
</form>
You can fill the options list with an API service.
apiUrl = 'http://127.0.0.1:8000/view1/';
<select2-aurora
formControlName="countryFC"
[apiUrl]="apiUrl"
>
</select2-aurora>
If your API has JWT token, then you can pass your token to this module
apiUrl = 'http://127.0.0.1:8000/view1/';
jwtToken = 'dfsdfe3423i4jfhsdjnvsjhr3h4j23h4j23h4j232j4';
<select2-aurora
formControlName="countryFC"
[apiUrl]="apiUrl"
[jwtToken]="jwtToken"
>
</select2-aurora>
If you use both optionList and apiUrl simultaneously, the module just use apiUrl.
The project is open source, and you can access to source code here
FAQs
Select2-aurora is a new implementation for Select Control Form. In this module, you are able to add options list just like before. It is possible to search in list. In order to get information from an API server, the output of your service must be a list
The npm package select2-aurora receives a total of 3 weekly downloads. As such, select2-aurora popularity was classified as not popular.
We found that select2-aurora demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.