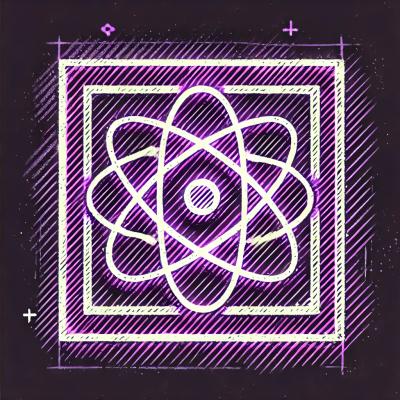
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
sequelize-search-builder
Advanced tools
Minimalist library for parsing search request to sequelize query
🚨 This package has moved! It is now maintained under @SequelizeSearchBuilder.
This is a lightweight library to convert search request (e.g. HTTP) to Sequelize ORM query.
npm install --save sequelize-search-builder
Example based on Express framework.
Direct generation of where/order/limit/offset query
const router = require('express').Router(),
models = require('../models'),
searchBuilder = require('sequelize-search-builder');
router.get('/search', async (req, res, next) => {
// Set req.query param to Search Builder constructor
const search = new searchBuilder(models.Sequelize, req.query),
whereQuery = search.getWhereQuery(),
orderQuery = search.getOrderQuery(),
limitQuery = search.getLimitQuery(),
offsetQuery = search.getOffsetQuery();
res.json({
data: await models.product.findAll({
include: [{ all: true, nested: true, duplicating: false }],
where: whereQuery,
order: orderQuery,
limit: limitQuery,
offset: offsetQuery,
logging: console.log,
})
});
});
Full query generation example (getFullQuery method)
res.json({
data: await models.product.findAll(search.getFullQuery({
include: [{ all: true, nested: true, duplicating: true }],
}))
});
You can set HTTP query string as second parameter for Seach Builder constructor (it will parse by 'qs' library to object).
// HTTP:
?filter[name]=John&filter[surname]=Smith
// req.query:
{ filter: { name: 'John', surname: 'Smith' } }
// getWhereQuery()
{ name: 'John', surname: 'Smith' }
// HTTP:
?filter[name]=John&filter[surname]=Smith&filter[_condition]=or
// req.query:
{ filter: { name: 'John', surname: 'Smith', _condition: 'or', } }
// getWhereQuery()
{ [Symbol(or)]: {name: 'John', surname: 'Smith'} }
// HTTP:
filter[age][gt]=100&filter[age][lt]=10&filter[age][_condition]=or&filter[name][iLike]=%john%&filter[_condition]=or
// req.query
{
filter: {
age: {
gt: 100,
lt: 10,
_condition: 'or',
},
name: {
iLike: '%john%',
},
_condition: 'or',
},
}
// getWhereQuery()
{
[Op.or]: {
[Op.or]: [{
age: {
[Op.gt]: 100,
},
}, {
age: {
[Op.lt]: 10,
},
}],
name: {
[Op.like]: '%john%',
},
},
}
If _condition parameter is absent - "and" will be used by default
// HTTP:
?filter[name]=desc
// req.query:
{ order: { name: 'desc' } }
// getOrderQuery()
[ [ 'name', 'desc' ] ]
You can find more examples in the tests of the project (test/index.js)
Git repository with DB tests: https://github.com/SequelizeSearchBuilder/sequelize-search-builder-db-tests
Request Option | Sequelize Symbol | Description |
---|---|---|
eq (=) | = (no Symbol) | Equal |
gt | Op.gt | Greater than |
gte | Op.gte | Greater than or equal |
lt | Op.lt | Less than |
lte | Op.lte | Less than or equal |
ne | Op.ne | Not equal |
between | Op.between | Between [value1, value2] |
notBetween | Op.notBetween | Not Between [value1, value2] |
in | Op.in | In value list [value1, value2, ...] |
notIn | Op.notIn | Not in value list [value1, value2, ...] |
like | Op.like | Like search (%value, value%, %value%) |
notLike | Op.notLike | Not like search (%value, value%, %value%) |
is | Op.is | is (used to check for NULL and boolean values) |
not | Op.not | not (used to check for NULL and boolean values) |
iLike | Op.iLike | case insensitive LIKE (PG only) |
notILike | Op.notILike | case insensitive NOT LIKE (PG only) |
regexp | Op.regexp | Regexp (MySQL and PG only) |
notRegexp | Op.notRegexp | Not Regexp (MySQL and PG only) |
iRegexp | Op.iRegexp | iRegexp (case insensitive) (PG only) |
notIRegexp | Op.notIRegexp | notIRegexp (case insensitive) (PG only) |
You can redefine configuration variables in rc file
Just create .sequelize-search-builderrc file in root folder of your project
Or use setter for 'config' parameter (setConfig)
RC file example:
{
"logging": false,
"fields": {
"filter" : "filter",
"order" : "order",
"limit" : "limit",
"offset" : "offset"
},
"default-limit": 10
}
Setter example:
new searchBuilder(models.Sequelize, req.query)
.setConfig({
logging: true,
});
You can use Sequelize Search Builder Client module for the generation request http search string on the client side.
You are Welcome =) Keep in mind:
npm run test
./node_modules/.bin/eslint .
FAQs
Minimalist library for parsing search request to sequelize query
The npm package sequelize-search-builder receives a total of 178 weekly downloads. As such, sequelize-search-builder popularity was classified as not popular.
We found that sequelize-search-builder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.