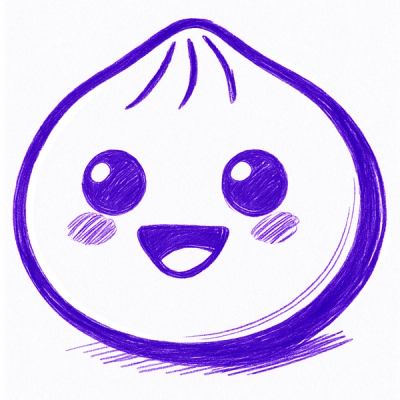
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
serialize-error
Advanced tools
The serialize-error npm package is used to serialize error objects into plain objects, retaining as much information as possible. It can be useful for logging errors or sending them over the network, as standard Error objects may not be properly serialized by default JSON.stringify.
Serialize an Error object
This feature allows you to serialize a standard Error object into a JSON-friendly format, including properties like name, message, and stack.
{"error": {"name": "Error", "message": "An error occurred!", "stack": "Error: An error occurred!\n at Object.<anonymous> (/path/to/file.js:2:15)\n at Module._compile (internal/modules/cjs/loader.js:1137:30)\n at Object.Module._extensions..js (internal/modules/cjs/loader.js:1157:10)\n at Module.load (internal/modules/cjs/loader.js:985:32)\n at Function.Module._load (internal/modules/cjs/loader.js:878:14)\n at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:71:12)\n at internal/main/run_main_module.js:17:47"}}
Serialize custom error properties
This feature allows you to serialize Error objects that have custom properties beyond the standard Error properties.
{"error": {"name": "CustomError", "message": "Something custom happened!", "customProperty": "Custom value", "stack": "CustomError: Something custom happened!\n at Object.<anonymous> (/path/to/file.js:2:15)\n at Module._compile (internal/modules/cjs/loader.js:1137:30)\n at Object.Module._extensions..js (internal/modules/cjs/loader.js:1157:10)\n at Module.load (internal/modules/cjs/loader.js:985:32)\n at Function.Module._load (internal/modules/cjs/loader.js:878:14)\n at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:71:12)\n at internal/main/run_main_module.js:17:47"}}
This package is similar to serialize-error in that it provides a way to serialize error objects to JSON. It may differ in the specific implementation or additional options provided for serialization.
While not exclusively for error objects, serialize-javascript allows for the serialization of JavaScript expressions, including errors. It can serialize functions, regexes, and dates, in addition to errors, which may make it more versatile in some scenarios compared to serialize-error.
Serialize/deserialize an error into a plain object
Useful if you for example need to JSON.stringify()
or process.send()
the error.
npm install serialize-error
import {serializeError, deserializeError} from 'serialize-error';
const error = new Error('π¦');
console.log(error);
//=> [Error: π¦]
const serialized = serializeError(error);
console.log(serialized);
//=> {name: 'Error', message: 'π¦', stack: 'Error: π¦\n at Object.<anonymous> β¦'}
const deserialized = deserializeError(serialized);
console.log(deserialized);
//=> [Error: π¦]
When a serialized error with a known name
is encountered, it will be deserialized using the corresponding error constructor, while unknown error names will be deserialized as regular errors:
import {deserializeError} from 'serialize-error';
const known = deserializeError({
name: 'TypeError',
message: 'π¦'
});
console.log(known);
//=> [TypeError: π¦] <-- Still a TypeError
const unknown = deserializeError({
name: 'TooManyCooksError',
message: 'π¦'
});
console.log(unknown);
//=> [Error: π¦] <-- Just a regular Error
The list of known errors can be extended globally. This also works if serialize-error
is a sub-dependency that's not used directly.
import {addKnownErrorConstructor} from 'serialize-error';
import {MyCustomError} from './errors.js'
addKnownErrorConstructor(MyCustomError);
Warning: The constructor must work without any arguments or this function will throw.
Serialize an Error
object into a plain object.
[object Buffer]
..toJSON()
method, then it's called instead of serializing the object's properties..toJSON()
implementation to handle circular references and enumerability of the properties.Type: Error | unknown
import {serializeError} from 'serialize-error';
class ErrorWithDate extends Error {
constructor() {
super();
this.date = new Date();
}
}
const error = new ErrorWithDate();
serializeError(error);
// => {date: '1970-01-01T00:00:00.000Z', name, message, stack}
import {serializeError} from 'serialize-error';
const error = new Error('Unicorn');
error.horn = {
toJSON() {
return 'x';
}
};
serializeError(error);
// => {horn: 'x', name, message, stack}
Deserialize a plain object or any value into an Error
object.
Error
objects are passed through.message
property are interpreted as errors.NonError
error.Type: {message: string} | unknown
Type: object
Type: number
Default: Number.POSITIVE_INFINITY
The maximum depth of properties to preserve when serializing/deserializing.
import {serializeError} from 'serialize-error';
const error = new Error('π¦');
error.one = {two: {three: {}}};
console.log(serializeError(error, {maxDepth: 1}));
//=> {name: 'Error', message: 'π¦', one: {}}
console.log(serializeError(error, {maxDepth: 2}));
//=> {name: 'Error', message: 'π¦', one: { two: {}}}
Type: boolean
Default: true
Indicate whether to use a .toJSON()
method if encountered in the object. This is useful when a custom error implements its own serialization logic via .toJSON()
but you prefer to not use it.
Predicate to determine whether a value looks like an error, even if it's not an instance of Error
. It must have at least the name
, message
, and stack
properties.
import {isErrorLike} from 'serialize-error';
const error = new Error('π¦');
error.one = {two: {three: {}}};
isErrorLike({
name: 'DOMException',
message: 'It happened',
stack: 'at foo (index.js:2:9)',
});
//=> true
isErrorLike(new Error('π¦'));
//=> true
isErrorLike(serializeError(new Error('π¦'));
//=> true
isErrorLike({
name: 'Bluberricious pancakes',
stack: 12,
ingredients: 'Blueberry',
});
//=> false
FAQs
Serialize/deserialize an error into a plain object
The npm package serialize-error receives a total of 8,395,856 weekly downloads. As such, serialize-error popularity was classified as popular.
We found that serialize-error demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainerβs token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.