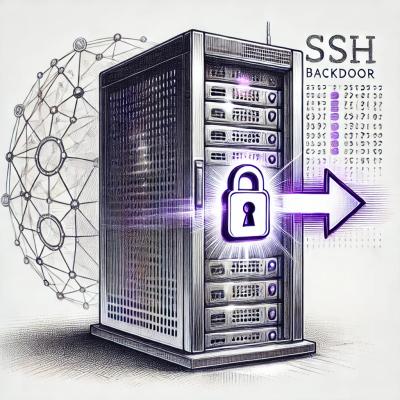
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Shades is a lodash inspired lens-like library.
A lens is a path into an object, which can be used to extract its values, or even "modify" them in place (by creating a new object with the value changed).
When writing immutable code, we very commonly end up with deeply nested data stores, e.g.:
const store = {
users: [
{
name: 'Jack Sparrow',
posts: [
{
title: 'Why is the rum always gone? A dissertation on Carribean trade deficit'
}
],
...
},
...
]
}
And updating a deeply nested structure will require heavy usage of the spread operator (or Object.assign
). E.g. To capitalize the title of the first post of the first user, you would write:
const userIdx = 0;
const postIdx = 0;
const capitalize = (string) => {...}
{...store,
users: store.users.map((user, idx) => (
idx === userIdx
? {...user,
posts: user.posts.map((post, idx) =>
idx === postIdx
? {...post,
title: capitalize(post.title)
}
: post)
}
: user
)
}
This is an enormous amount of obfuscating boiler plate code for a very simple update.
With lenses, we could write this update much more declaratively:
mod(`.users[${userIdx}].posts[${postIdx}]`)
(capitalize)
(store)
A lens is simply a string which describes a path into an object. It can include object accesses and array indicies.
The focus
of the lens is the final value referenced in the path.
Lenses can be constructed with the lens function, or passed as string literals into the lens consumer functions (get, set, mod)
Combining lenses with ES6 template strings can be a concise way to use environment variables to create a dynamic path.
For more powerful dynamic or mutlifoci lenses, check out traversals.
> ".a.b[3].d" // focus is the d field
> const idx = 10
> `.a.b[${idx}]` // focus is the 11th element of b
get
consumes a lens and produces a function that takes in an object obj
and outputs the focus of its lens.
> get('.a.b.c')({a: {b: {c: 7}}})
7
set
consumes a lens and produces a function that takes in a constant value const
, and produces a function consuming an object obj
and outputs a clone of obj
with the focus of the lens replaced with const
> set('.a.b.c')(10)({a: {b: {c: 7}}})
{a: {b: {c: 10}}}
mod
consumes a lens and produces a function that takes in a modifiying function m
for the focus of the lens, and produces a function consuming an object obj
, then outputs a clone of obj
with the focus of the lens replaced with m
's output.
> const inc = n => n + 1
> mod('.a.b.c')(inc)({a: {b: {c: 7}}})
{a: {b: {c: 8}}}
lens
consumes a path into an object and produces a corresponding lens function. All lens consumers (get, set, mod) will accept a literal string. However, lenses are composable via compose, so lenses can be built piece by piece.
compose
composes the foci of multiple lenses
Note: It is unlikely that you will ever need to call this directly
> const l = compose('.a.b', '.c.d')
> get(l)({a: {b: {c: {d: 10}}}})
10
Traversals are lenses that have multiple focus points. These can be multiple elements in an array or multiple keys in an object. They can all still be used with the lens functions described above.
matching
consumes a predicate and produces a lens which will act over every element which returns true
for the predicate.
> const even = n => n % 2 == 0
> get(matching(even))([1, 2, 3, 4])
[2, 4]
> get(matching(even))({a: 1, b: 2, c: 3, d: 4})
{b: 2, d: 4}
> const mul10 = n => n * 10
> mod(matching(even))(mul10)([1, 2, 3, 4])
[1, 20, 3, 40]
> mod(matching(even))(mul10)([{a: 1, b: 2, c: 3, d: 4})
{a: 1, b: 20, c: 3, d: 40}
unless
is the opposite of matching
. It consumes a predicate and produces a lens which will act over every element which returns false
for the predicate.
> const even = n => n % 2 == 0
> get(all))([1, 2, 3, 4])
[1, 3]
> const mul10 = n => n * 10
> mod(unless(even))(mul10)([1, 2, 3, 4])
[10, 2, 30, 40]
all
is the identity traversal. It acts over every element. It optionally takes an additional lens, and for each element in the result set will apply the given lens.
_Note: this is only necessary for get
. mod
and set
will already do the appropriate thing if all
appears in the middle of a lens
> const mul10 = n => n * 10
> mod(all())(mul10)({a: 1, b: 2, c: 3, d: 4})
{a: 10, b: 20, c: 30, d: 40}
> const even = n => n % 2 == 0
> get('a', all('b.c'))({a: [{b: {c: 1}}, {b: {c: 2}}, {b: {c: 3}}]})
[1, 2, 3]
> mod('a', all(), 'b.c')(mul10)({a: [{b: {c: 1}}, {b: {c: 2}}, {b: {c: 3}}]})
[10, 20, 30]
has
is a predicate construction function. It takes a pattern of keys and values and produces a function that takes value and returns true
if the given value at least has equivalent keys and values the given pattern
> has({a: {b: 3}})({a: {b: 3, c: 4}, d: 5})
true
has
composes well filter
and matching
pipelines
> [{type: 'oper': expr: '+'}, {type: 'lambda', expr: 'a => a + 1'}].filter(has({type: 'oper'}))
[{type: 'oper': expr: '+'}]
> const id = 5
> const users = [{id: 1, name: 'Elizabeth', likes: 1,000,000,000}, {id: 3, name: 'Bootstrap Bill', likes: 12}, {id: 5, name: 'Jack', likes: 41}]
> mod(matching(has({id})), '.likes')(inc)(users)
[{id: 1, name: 'Elizabeth', likes: 1,000,000,000}, {id: 3, name: 'Bootstrap Bill', likes: 12}, {id: 5, name: 'Jack', likes: 42}]
The keys in the pattern may also be predicate functions. In this case, values from the input object will be passed to the predicates.
> users.map(has({name: _.isString, likes: n => n > 1000}))
[true, false, false]
A more generic, curried map
. If applied to a list, it behaves like Array::map
. Applied to a an object, it transforms the values (although the key will be supplied as a second argument)
> map(inc)([1, 2, 3, 4])
[2, 3, 4, 5]
> map((key, value) => `${value} was at {key}`)({a: 1, b: 2})
{a: '1 was at a', b: '2 was at b'}
A more generic, curried filter
. If applied to a list, it behaves like Array::filter
. Applied to a an object, it filters based on the values (although the key will be supplied as a second argument)
> filter(isEven)([1, 2, 3, 4])
[2, 4]
> filter((key, value) => isEven(key) && isOdd(value))({2: 1, 3: 1})
{2: 1}
Consumes a variadic number of transformers (i.e. Lens
es that have already been applied to a path and a transforming function) and a state function and applies each of them in order to a state object, producing a transformed object
> const state = {
modal: {
isOpen: true,
idx: 5,
}
}
> updateAll(
mod('.modal.isOpen')(toggle),
set('.modal.idx')(0),
)(state)
{
modal: {
isOpen: false,
idx: 0,
}
}
Negates a boolean
> toggle(true)
false
Increments a number
> inc(5)
6
Consumes an element x
and an array xs
and returns a new array with x
APPENDED to xs
(not prepended, which is more typical with cons
and lists. This is to make it easier to use in pipelined scenarios)
A function level equivalent of the &&
operator. It consumes an arbitrary number of functions that take the same argument types and produce booleans, and returns a single function that takes the same arguments, and returns true
if all of the functions return true
> and(isEven, greaterThan(3))(6)
true
> [42, 2, 63].filter(and(isEven, greaterThan(3)))
[42]
A function level equivalent of the ||
operator. It consumes an arbitrary number of functions that take the same argument types and produce booleans, and returns a single function that takes the same arguments, and returns true
if any of the functions return true
> or(isEven, greaterThan(3))(5)
true
> or(isEven, greaterThan(3))(1)
false
A function level equivalent of the !
operator. It consumes a function that produces a boolean, and returns a function that takes the same arguments, and returns the negation of the output
const isOdd = not(isEven)
Produces the given value forever
> [1, 2, 3].map(always(5))
[5, 5, 5]
Takes a 2-curried function and flips the order of the arguments
> const lessThanEq = flip(greaterThanEq)
FAQs
Lens-like functionality with a lodash-style interface.
The npm package shades receives a total of 7,296 weekly downloads. As such, shades popularity was classified as popular.
We found that shades demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.