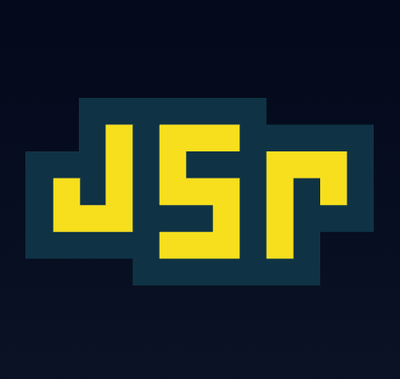
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
ShellJS is a portable (Windows/Linux/OS X) implementation of Unix shell commands on top of the Node.js API. It provides the ability to script shell commands within JavaScript files and is used for tasks such as file manipulation, program execution, and script automation.
File Operations
ShellJS can perform file operations such as copying, removing, and moving files or directories.
const shell = require('shelljs');
shell.cp('-R', 'source_folder', 'destination_folder');
shell.rm('-rf', 'folder_to_delete');
shell.mv('old_file.txt', 'new_file.txt');
Executing Shell Commands
It can execute any shell command and capture its output.
const shell = require('shelljs');
let output = shell.exec('echo hello world', {silent:true}).stdout;
console.log(output);
Searching and Modifying Files
ShellJS can search for files that match a pattern and perform text replacement within files.
const shell = require('shelljs');
let files = shell.find('.').filter(file => file.match(/\.js$/));
shell.sed('-i', 'original_text', 'new_text', 'file.js');
Environment Variables
It allows manipulation of environment variables within a script.
const shell = require('shelljs');
shell.env['VAR_NAME'] = 'value';
console.log(shell.env['VAR_NAME']);
Directory Navigation
ShellJS can change the current working directory and get the present working directory.
const shell = require('shelljs');
shell.cd('path/to/directory');
console.log(shell.pwd().stdout);
This is a core Node.js module that allows you to execute shell commands and is more low-level compared to ShellJS. It does not provide the Unix shell command syntax, requiring more manual setup for cross-platform compatibility.
Execa is a process execution tool that aims to be more feature-rich and have better Windows support than Node.js's child_process. It returns promises and can handle multiple processes simultaneously.
Cross-spawn is a cross-platform solution for spawning child processes with command-line arguments. It normalizes Node.js issues on Windows and is used when you need to spawn processes with command-line arguments that are shell-friendly.
This project is young and experimental. Use at your own risk.
ShellJS is a portable (Windows included) implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands.
The project is unit-tested and is being used at Mozilla's pdf.js.
require('shelljs/global');
// Copy files to release dir
mkdir('-p', 'out/Release');
cp('-R', 'stuff/*', 'out/Release');
// Replace macros in each .js file
cd('lib');
for (file in ls('*.js')) {
sed('-i', 'BUILD_VERSION', 'v0.1.2', file);
sed('-i', /.*REMOVE_THIS_LINE.*\n/, '', file);
sed('-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file);
}
cd('..');
// Run external tool synchronously
if (exec('git commit -am "Auto-commit"').code !== 0) {
echo('Error: Git commit failed');
exit(1);
}
The example above uses the convenience script shelljs/global
to reduce verbosity. If polluting your global namespace is not desirable, simply require shelljs
.
Example:
var shell = require('shelljs');
shell.echo('hello world');
A convenience script shelljs/make
is also provided to mimic the behavior of a Unix Makefile. In this case all shell objects are global, and command line arguments will cause the script to execute only the corresponding function in the global target
object. To avoid redundant calls, target functions are executed only once per script.
Example:
//
// Example file: make.js
//
require('shelljs/make');
target.all = function() {
target.bundle();
target.docs();
}
// Bundle JS files
target.bundle = function() {
cd(__dirname);
mkdir('build');
cd('lib');
cat('*.js').to('../build/output.js');
}
// Generate docs
target.docs = function() {
cd(__dirname);
mkdir('docs');
cd('lib');
for (file in ls('*.js')) {
var text = grep('//@', file); // extract special comments
text.replace('//@', ''); // remove comment tags
text.to('docs/my_docs.md');
}
}
To run the target all
, call the above script without arguments: $ node make
. To run the target docs
: $ node make docs
, and so on.
Via npm:
$ npm install shelljs
Or simply copy shell.js
into your project's directory, and require()
accordingly.
All commands run synchronously, unless otherwise stated.
Changes to directory dir
for the duration of the script
Returns the current directory.
Available options:
-R
: recursive-a
: all files (include files beginning with .
)Examples:
ls('projs/*.js');
ls('-R', '/users/me', '/tmp');
ls('-R', ['/users/me', '/tmp']); // same as above
Returns list of files in the given path, or in current directory if no path provided.
For convenient iteration via for (file in ls())
, the format returned is a hash object:
{ 'file1':null, 'dir1/file2':null, ...}
.
Examples:
find('src', 'lib');
find(['src', 'lib']); // same as above
for (file in find('.')) {
if (!file.match(/\.js$/))
continue;
// all files at this point end in '.js'
}
Returns list of all files (however deep) in the given paths. For convenient iteration
via for (file in find(...))
, the format returned is a hash object:
{ 'file1':null, 'dir1/file2':null, ...}
.
The main difference from ls('-R', path)
is that the resulting file names
include the base directories, e.g. lib/resources/file1
instead of just file1
.
Available options:
-f
: force-r, -R
: recursiveExamples:
cp('file1', 'dir1');
cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
Copies files. The wildcard *
is accepted.
Available options:
-f
: force-r, -R
: recursiveExamples:
rm('-rf', '/tmp/*');
rm('some_file.txt', 'another_file.txt');
rm(['some_file.txt', 'another_file.txt']); // same as above
Removes files. The wildcard *
is accepted.
Available options:
f
: forceExamples:
mv('-f', 'file', 'dir/');
mv('file1', 'file2', 'dir/');
mv(['file1', 'file2'], 'dir/'); // same as above
Moves files. The wildcard *
is accepted.
Available options:
p
: full path (will create intermediate dirs if necessary)Examples:
mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
Creates directories.
Available expression primaries:
'-d', 'path'
: true if path is a directory'-f', 'path'
: true if path is a regular fileExamples:
if (test('-d', path)) { /* do something with dir */ };
if (!test('-f', path)) continue; // skip if it's a regular file
Evaluates expression using the available primaries and returns corresponding value.
Examples:
var str = cat('file*.txt');
var str = cat('file1', 'file2');
var str = cat(['file1', 'file2']); // same as above
Returns a string containing the given file, or a concatenated string
containing the files if more than one file is given (a new line character is
introduced between each file). Wildcard *
accepted.
Examples:
cat('input.txt').to('output.txt');
Analogous to the redirection operator >
in Unix, but works with JavaScript strings (such as
those returned by cat
, grep
, etc). Like Unix redirections, to()
will overwrite any existing file!
Available options:
-i
: Replace contents of 'file' in-place. Note that no backups will be created!Examples:
sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
Reads an input string from file
and performs a JavaScript replace()
on the input
using the given search regex and replacement string. Returns the new string after replacement.
Examples:
grep('GLOBAL_VARIABLE', '*.js');
Reads input string from given files and returns a string containing all lines of the
file that match the given regex_filter
. Wildcard *
accepted.
Examples:
var nodeExec = which('node');
Searches for command
in the system's PATH. On Windows looks for .exe
, .cmd
, and .bat
extensions.
Returns string containing the absolute path to the command.
Examples:
echo('hello world');
var str = echo('hello world');
Prints string to stdout, and returns string with additional utility methods
like .to()
.
Exits the current process with the given exit code.
Object containing environment variables (both getter and setter). Shortcut to process.env.
Available options (all false
by default):
async
: Asynchronous execution. Needs callback.silent
: Do not echo program output to console.Examples:
var version = exec('node --version', {silent:true}).output;
Executes the given command
synchronously, unless otherwise specified.
When in synchronous mode returns the object { code:..., output:... }
, containing the program's
output
(stdout + stderr) and its exit code
. Otherwise the callback
gets the
arguments (code, output)
.
Searches and returns string containing a writeable, platform-dependent temporary directory. Follows Python's tempfile algorithm.
Tests if error occurred in the last command. Returns null
if no error occurred,
otherwise returns string explaining the error
Example:
var silentState = silent();
silent(true);
/* ... */
silent(silentState); // restore old silent state
Suppresses all output if state = true
. Returns state if no arguments given.
This function is being deprecated. Use test()
instead.
Returns true if all the given paths exist.
This function is being deprecated. Use silent(false) instead.
Enables all output (default)
v0.0.2 (2012-03-15)
FAQs
Portable Unix shell commands for Node.js
The npm package shelljs receives a total of 5,763,570 weekly downloads. As such, shelljs popularity was classified as popular.
We found that shelljs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.