Comparing version 1.3.0 to 1.3.1
@@ -99,2 +99,3 @@ declare module 'shoukaku' { | ||
export interface ShoukakuPlayOptions { | ||
noReplace?: boolean, | ||
startTime?: boolean | number; | ||
@@ -101,0 +102,0 @@ endTime?: boolean | number; |
{ | ||
"name": "shoukaku", | ||
"version": "1.3.0", | ||
"version": "1.3.1", | ||
"description": "A lavalink client for Discord.js v12 only", | ||
@@ -5,0 +5,0 @@ "main": "index.js", |
114
README.md
@@ -14,113 +14,3 @@ ## Shoukaku | ||
### A Full Blown Lavalink Wrapper designed around Discord.js v12 | ||
✅ Currently being used by: | ||
[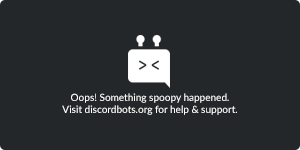](https://discordbots.org/bot/424137718961012737) | ||
### Why Shoukaku? | ||
✅ Designed to used in Discord.JS v12 | ||
✅ Straightforward, Maintained, and Reliable. | ||
✅ Stable for long term usage. | ||
✅ Offers features that other libraries don't have. | ||
✅ Very cute and reliable Shipgirl ❤ (Important) | ||
### Documentation | ||
https://deivu.github.io/Shoukaku/?api | ||
### Installation | ||
For Stable | ||
``` | ||
npm i shoukaku | ||
``` | ||
For Master | ||
``` | ||
npm i Deivu/Shoukaku | ||
``` | ||
### Changelogs | ||
You can view it on [CHANGELOGS.MD](https://github.com/Deivu/Shoukaku/blob/master/CHANGELOGS.MD) file in this repository. | ||
### Support Server | ||
If you need help on using this, Join Here [ShipGirls Community](https://discordapp.com/invite/FVqbtGu) and `ask at #support`. | ||
### Issues or Bugs | ||
Feel free to open an issue in the [Issues](https://github.com/Deivu/Shoukaku/issues) section of this repository. | ||
### Notes | ||
> If you want to help in development, you can use the wrapper and report the issues you experienced on using it, or Submit a PR if you think you can improve something. | ||
> There is a Discord.JS actual implementation and a simple implementation examples below. | ||
### Starting a Lavalink Server | ||
[View Lavalink README here](https://github.com/Frederikam/Lavalink/blob/master/README.md) | ||
### Discord.js example implementation | ||
[View Kongou's source code here](https://github.com/Deivu/Kongou) | ||
### Really simple example of using this | ||
```js | ||
const { Client } = require('discord.js'); | ||
const { Shoukaku } = require('shoukaku'); | ||
const LavalinkServer = [{ name: 'Localhost', host: 'localhost', port: 6969, auth: 'big_weeb' }]; | ||
const ShoukakuOptions = { moveOnDisconnect: false, resumable: false, resumableTimeout: 30, reconnectTries: 2, restTimeout: 10000 }; | ||
class ExampleBot extends Client { | ||
constructor(opts) { | ||
super(opts); | ||
this.shoukaku = new Shoukaku(this, LavalinkServer, ShoukakuOptions); | ||
} | ||
login() { | ||
this._setupShoukakuEvents(); | ||
this._setupClientEvents(); | ||
return super.login(); | ||
} | ||
_setupShoukakuEvents() { | ||
this.shoukaku.on('ready', (name) => console.log(`Lavalink Node: ${name} is now connected`)); | ||
// You must handle error event | ||
this.shoukaku.on('error', (name, error) => console.log(`Lavalink Node: ${name} emitted an error.`, error)); | ||
this.shoukaku.on('close', (name, code, reason) => console.log(`Lavalink Node: ${name} closed with code ${code}. Reason: ${reason || 'No reason'}`)); | ||
this.shoukaku.on('disconnected', (name, reason) => console.log(`Lavalink Node: ${name} disconnected. Reason: ${reason || 'No reason'}`)); | ||
} | ||
_setupClientEvents() { | ||
this.on('message', async (msg) => { | ||
if (msg.author.bot || !msg.guild) return; | ||
if (!msg.content.startsWith('$play')) return; | ||
if (this.shoukaku.getPlayer(msg.guild.id)) return; | ||
const args = msg.content.split(' '); | ||
if (!args[1]) return; | ||
const node = this.shoukaku.getNode(); | ||
let data = await node.rest.resolve(args[1]); | ||
if (!data) return; | ||
if (Array.isArray(data)) data = data[0]; | ||
const player = await node.joinVoiceChannel({ | ||
guildID: msg.guild.id, | ||
voiceChannelID: msg.member.voice.channelID | ||
}); | ||
const cleanFunction = (param) => { | ||
console.log(param); | ||
player.disconnect(); | ||
} | ||
player.on('end', cleanFunction); | ||
player.on('closed', cleanFunction); | ||
player.on('error', cleanFunction); | ||
player.on('nodeDisconnect', cleanFunction); | ||
await player.playTrack(data.track); | ||
await msg.channel.send("Now Playing: " + data.info.title); | ||
}); | ||
this.on('ready', () => console.log('Bot is now ready')); | ||
} | ||
} | ||
new ExampleBot() | ||
.login('token') | ||
.catch(console.error); | ||
``` | ||
### Shoukaku's module for Kashima | ||
> Not intended for public usage. |
@@ -69,2 +69,3 @@ /** | ||
* @typedef {Object} ShoukakuPlayOptions | ||
* @property {boolean} [noReplace=true] Specifies if the player will not replace the current track when executing this action. | ||
* @property {boolean|number} [startTime=false] In milliseconds on when to start. | ||
@@ -76,2 +77,3 @@ * @property {boolean|number} [endTime=false] In milliseconds on when to end. | ||
return { | ||
noReplace: true, | ||
startTime: false, | ||
@@ -78,0 +80,0 @@ endTime: false |
@@ -0,0 +0,0 @@ /** |
@@ -0,0 +0,0 @@ /** |
@@ -102,3 +102,3 @@ const { ShoukakuStatus } = require('../constants/ShoukakuConstants.js'); | ||
throw new ShoukakuError('No Options or Callback supplied.'); | ||
this._callback = callback; | ||
@@ -117,3 +117,3 @@ | ||
this.state = ShoukakuStatus.CONNECTING; | ||
const { guildID, voiceChannelID, deaf, mute } = options; | ||
@@ -128,4 +128,3 @@ this._sendDiscordWS({ guild_id: guildID, channel_id: voiceChannelID, self_deaf: deaf, self_mute: mute }); | ||
this.player.removeAllListeners(); | ||
this.player._clearTrack(); | ||
this.player._clearBands(); | ||
this.player._resetPlayer(); | ||
if (this.state !== ShoukakuStatus.DISCONNECTED) { | ||
@@ -162,3 +161,3 @@ this._destroy() | ||
_clearVoice() { | ||
_clearVoice() { | ||
this.lastServerUpdate = null; | ||
@@ -165,0 +164,0 @@ this.sessionID = null; |
const EventEmitter = require('events'); | ||
const { ShoukakuPlayOptions, ShoukakuStatus } = require('../constants/ShoukakuConstants.js'); | ||
const util = require('./util/ShoukakuUtil.js'); | ||
const ShoukakuLink = require('./ShoukakuLink.js'); | ||
@@ -49,2 +50,7 @@ const ShoukakuError = require('../constants/ShoukakuError.js'); | ||
this.position = 0; | ||
this.bassboost = false; | ||
this.vaporwave = false; | ||
this.nightcore = 1.0; | ||
this.karaoke = false; | ||
} | ||
@@ -149,2 +155,3 @@ | ||
if (!track) return false; | ||
options = util.mergeDefault(ShoukakuPlayOptions, options); | ||
const payload = {}; | ||
@@ -154,7 +161,7 @@ Object.defineProperty(payload, 'op', { value: 'play', enumerable: true }); | ||
Object.defineProperty(payload, 'track', { value: track, enumerable: true }); | ||
Object.defineProperty(payload, 'noReplace', { value: true, enumerable: true }); | ||
Object.defineProperty(payload, 'noReplace', { value: options.noReplace, enumerable: true }); | ||
if (options.startTime) Object.defineProperty(payload, 'startTime', { value: options.startTime, enumerable: true }); | ||
if (options.endTime) Object.defineProperty(payload, 'endTime', { value: options.endTime, enumerable: true }); | ||
await this.voiceConnection.node.send(payload); | ||
this.track = track; | ||
if (track !== this.track) this.track = track; | ||
return true; | ||
@@ -189,3 +196,3 @@ } | ||
}); | ||
this.paused = pause; | ||
if (pause !== this.paused) this.paused = pause; | ||
return true; | ||
@@ -207,2 +214,4 @@ } | ||
}); | ||
this.bands = JSON.parse(JSON.stringify(bands)); | ||
this.bassboost = false; | ||
return true; | ||
@@ -224,3 +233,3 @@ } | ||
}); | ||
this.volume = volume; | ||
if (volume !== this.volume) this.volume = volume; | ||
return true; | ||
@@ -244,12 +253,68 @@ } | ||
async setNightcore(speed, hq = false) { | ||
if (!speed) return false; | ||
await this.voiceConnection.node.send({ | ||
op: 'nightcore', | ||
guildId: this.voiceConnection.guildID, | ||
speed, | ||
hq | ||
}); | ||
if (speed !== this.nightcore) this.nightcore = speed; | ||
return true; | ||
} | ||
_clearTrack() { | ||
async setKaraoke(enabled = false) { | ||
await this.voiceConnection.node.send({ | ||
op: 'karaoke', | ||
guildId: this.voiceConnection.guildID, | ||
enabled | ||
}); | ||
if (enabled !== this.karaoke) this.karaoke = enabled; | ||
return true; | ||
} | ||
async setBassBoost(enabled = false) { | ||
await this.voiceConnection.node.send({ | ||
op: 'bassboost', | ||
guildId: this.voiceConnection.guildID, | ||
enabled | ||
}); | ||
if (enabled !== this.bassboost) this.bassboost = enabled; | ||
this.bands.length = 0; | ||
return true; | ||
} | ||
async setVaporWave(enabled = false) { | ||
await this.voiceConnection.node.send({ | ||
op: 'vaporwave', | ||
guildId: this.voiceConnection.guildID, | ||
enabled | ||
}); | ||
if (enabled !== this.vaporwave) this.vaporwave = enabled; | ||
return true; | ||
} | ||
async resetFilters(reset) { | ||
if (!reset) return false; | ||
await this.voiceConnection.node.send({ | ||
op: 'reset', | ||
guildId: this.voiceConnection.guildID, | ||
reset | ||
}); | ||
let oldpos = Number(this.position); | ||
this._resetPlayer(); | ||
this.position = oldpos; | ||
return true; | ||
} | ||
_resetPlayer() { | ||
this.track = null; | ||
this.position = 0; | ||
this.bands.length = 0; | ||
this.vaporwave = false; | ||
this.bassboost = false; | ||
this.nightcore = 1.0; | ||
this.karaoke = false; | ||
} | ||
_clearBands() { | ||
this.bands.length = 0; | ||
} | ||
async _resume() { | ||
@@ -261,2 +326,6 @@ try { | ||
if (this.volume !== 100) await this.setVolume(this.volume); | ||
if (this.vaporwave) await this.setVaporWave(this.vaporwave); | ||
if (this.bassboost) await this.setBassBoost(this.bassboost); | ||
if (this.karaoke) await this.setKaraoke(this.karaoke); | ||
if (this.nightcore !== 1.0) await this.setNightcore(this.nightcore); | ||
this._listen('resumed', null); | ||
@@ -268,7 +337,11 @@ } catch (error) { | ||
_listen(event, data) { | ||
if (endEvents.includes(event)) { | ||
if (event === 'nodeDisconnect') this._clearTrack() && this._clearBands(); | ||
else this._clearTrack(); | ||
if (event === 'nodeDisconnect') { | ||
this._resetPlayer(); | ||
} else { | ||
this.track = null; | ||
this.position = 0; | ||
} | ||
this.emit(event, data); | ||
@@ -275,0 +348,0 @@ return; |
@@ -0,0 +0,0 @@ const Websocket = require('ws'); |
@@ -0,0 +0,0 @@ const Fetch = require('node-fetch'); |
@@ -0,0 +0,0 @@ const { ShoukakuStatus } = require('../constants/ShoukakuConstants.js'); |
@@ -0,0 +0,0 @@ const { RawRouter, ReconnectRouter } = require('./router/ShoukakuRouter.js'); |
@@ -0,0 +0,0 @@ const ShoukakuError = require('../constants/ShoukakuError.js'); |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
1616
65863
16