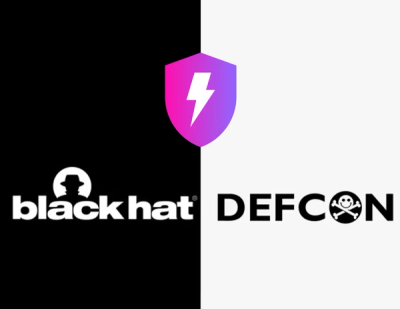
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
simple-oauth2-stack-exchange
Advanced tools
A simple Node.js client library for Stack Exchange OAuth2.
This library is a wrapper around Simple OAuth2 Library
Specially made for Authorization Code Flow with StackExchange.
Latest Node 8 LTS or newer versions.
npm install --save simple-oauth2 simple-oauth2-stack-exchange
or
yarn add simple-oauth2 simple-oauth2-stack-exchange
const simpleOAuth2StackExchange = require('simple-oauth2-stack-exchange');
const stackExchange = simpleOAuth2StackExchange.create(options);
stackExchange
object exposes 3 keys:
access_token
Required options
Option | Description |
---|---|
clientId | Your App Id. |
clientSecret | Your App Secret Id. |
callbackURL | Callback configured when you created the app. |
Other options
Option | Default | Description |
---|---|---|
scope | [] | https://api.stackexchange.com/docs/authentication |
state | '' | Your CSRF anti-forgery token. More at: https://auth0.com/docs/protocols/oauth2/oauth-state |
returnError | false | When is false (default), will call the next middleware with the error object. When is true, will set req.tokenError to the error, and call the next middleware as if there were no error. |
authorizeHost | 'https://stackoverflow.com' | |
authorizePath | '/oauth' | |
tokenHost | 'https://stackoverflow.com' | |
tokenPath | '/oauth/access_token' | |
authorizeOptions | {} | Pass extra parameters when requesting authorization. |
tokenOptions | {} | Pass extra parameters when requesting access_token. |
const oauth2 = require('simple-oauth2').create({
client: {
id: process.env.STACK_EXCHANGE_APP_ID,
secret: process.env.STACK_EXCHANGE_APP_SECRET
},
auth: {
authorizeHost: 'https://stackoverflow.com'
authorizePath: '/oauth',
tokenHost: 'https://stackoverflow.com',
tokenPath: '/oauth/access_token'
}
});
router.get('/auth/stack-exchange', (req, res) => {
const authorizationUri = oauth2.authorizationCode.authorizeURL({
redirect_uri: 'http://localhost:3000/auth/stack-exchange/callback',
});
res.redirect(authorizationUri);
});
router.get('/auth/stack-exchange/callback', async(req, res) => {
const code = req.query.code;
const options = {
code,
redirect_uri: 'http://localhost:3000/auth/stack-exchange/callback'
};
try {
// The resulting token.
const result = await oauth2.authorizationCode.getToken(options);
// Exchange for the access token.
const token = oauth2.accessToken.create(result);
return res.status(200).json(token);
} catch (error) {
console.error('Access Token Error', error.message);
return res.status(500).json('Authentication failed');
}
});
const simpleOAuth2StackExchange = require('simple-oauth2-stack-exchange');
const stackExchange = simpleOAuth2StackExchange.create({
clientId: process.env.STACK_EXCHANGE_APP_ID,
clientSecret: process.env.STACK_EXCHANGE_APP_SECRET,
callbackURL: 'http://localhost:3000/auth/stack-exchange/callback'
});
// Ask the user to authorize.
router.get('/auth/stack-exchange', stackExchange.authorize);
// Exchange the token for the access token.
router.get('/auth/stack-exchange/callback', stackExchange.accessToken, (req, res) => {
return res.status(200).json(req.token);
});
FAQs
A simple Node.js client library for Stack Exchange OAuth2.
The npm package simple-oauth2-stack-exchange receives a total of 1 weekly downloads. As such, simple-oauth2-stack-exchange popularity was classified as not popular.
We found that simple-oauth2-stack-exchange demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.