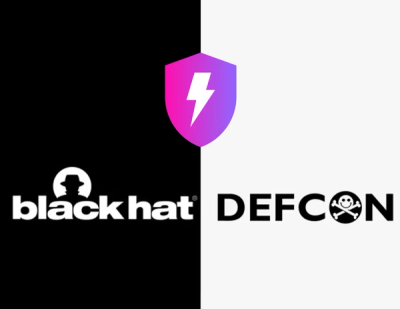
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
simple-sort
Advanced tools
simple-sort
provides a straightforward collection of sorting methods and sort functions.
simple-sort
?simple-sort
API.$ npm install simple-sort
Import the library:
import Sorter from 'simple-sort'; // or var Sorter = require('simple-sort').default;
Create an instance:
const sorter = new Sorter('en');
####Sorting methods:
// sample data array
const numArr = [2,3,1];
// sort values in ascending order
sorter.sortAsc(numArr);
// -> [1,2,3]
// sort values in descending order
sorter.sortDes(numArr);
// -> [3,2,1]
// sample array
const wordArr = ['Janeway', 'Picard', 'Cisco'];
// sort by locale in ascending order
sorter.sortByLocaleAsc(wordArr);
// -> ['Cisco','Janeway','Picard']
// sort by locale in descending order
sorter.sortByLocaleDes(wordArr);
// -> ['Picard','Janeway','Cisco']
// sample array
const objArr = [{num:2},{num:3},{num:1}];
// sort array of objects by property in ascending order
sorter.sortByPropAsc(objArr, 'num');
// -> [{num:1},{num:2},{num:3}]
// sort array of objects by property in descending order
sorter.sortByPropDes(objArr, 'num');
// -> [{num:3},{num:2},{num:1}]
// sample array
const objStrArr = [{name:'Janeway'},{name:'Picard'},{name:'Cisco'}];
// locale sort array of objects by property in ascending order
sorter.localeSortByPropAsc(objStrArr, 'name');
// -> [{name:'Cisco'},{name:'Janway'},{name:'Picard'}]
// locale sort array of objects by property in descending order
sorter.localSortByPropDes(objStrArr, 'name');
// -> [{name:'Picard'},{name:'Janway'},{name:'Cisco'}]
####Function-returning methods:
Each of the previous sort methods are also available as sort functions to pass into Array.sort()
. All function-returning methods have a Func
suffix.
// sample data array
const numArr = [2,3,1];
// sort values in asending order
numArr.sort(sorter.sortAscFunc());
// numArr -> [1,2,3]
// sort values in descending order
numArr.sort(sorter.sortDesFunc());
// numArr -> [3,2,1]
// sample array
const wordArr = ['Janeway', 'Picard', 'Cisco'];
// sort by locale in ascending order
wordArr.sort(sorter.sortByLocaleAscFunc());
// wordArr -> ['Cisco','Janeway','Picard']
// sort by locale in descending order
wordArr.sort(sorter.sortByLocaleDesFunc());
// wordArr -> ['Picard','Janeway','Cisco']
// sample array
const objArr = [{num:2},{num:3},{num:1}];
// sort array of objects by property in ascending order
objArr.sort(sorter.sortByPropAscFunc('num'));
// objArr -> [{num:1},{num:2},{num:3}]
// sort array of objects by property in descending order
objArr.sort(sorter.sortByPropDesFunc('num'));
// objArr -> [{num:3},{num:2},{num:1}]
// sample array
const objStrArr = [{name:'Janeway'},{name:'Picard'},{name:'Cisco'}];
// locale sort array of objects by property in ascending order
objStrArr.sort(sorter.localeSortByPropAscFunc('name'));
// objStrArr -> [{name:'Cisco'},{name:'Janway'},{name:'Picard'}]
// locale sort array of objects by property in descending order
objStrArr.sort(sorter.localSortByPropDesFunc('name'));
// objStrArr -> [{name:'Picard'},{name:'Janway'},{name:'Cisco'}]
Documentation of all available methods can be found here.
Contributions are welcome! If you have any issues and/or contributions you would like to make, feel free to file an issue and/or issue a pull request.
Apache License Version 2.0
Copyright (c) 2016 by Ryan Burgett.
FAQs
Straightforward collection of sorting methods and sort functions.
The npm package simple-sort receives a total of 1 weekly downloads. As such, simple-sort popularity was classified as not popular.
We found that simple-sort demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.