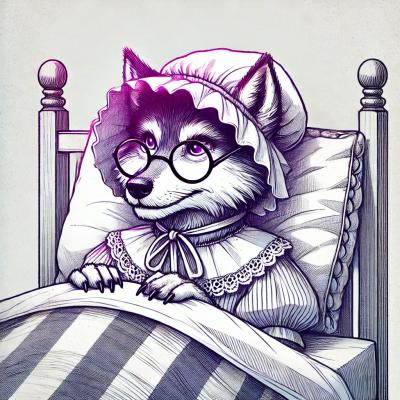
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
simple-statistics
Advanced tools
The simple-statistics npm package provides a comprehensive set of statistical tools and functions for performing various statistical analyses. It is designed to be easy to use and covers a wide range of statistical operations, from basic descriptive statistics to more complex regression analysis.
Descriptive Statistics
This feature allows you to calculate basic descriptive statistics such as mean, median, and variance. The code sample demonstrates how to compute these statistics for a given dataset.
const ss = require('simple-statistics');
const data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const mean = ss.mean(data);
const median = ss.median(data);
const variance = ss.variance(data);
console.log(`Mean: ${mean}, Median: ${median}, Variance: ${variance}`);
Probability Distributions
This feature provides functions to work with various probability distributions, including normal and binomial distributions. The code sample shows how to generate a random normal value and a binomial distribution.
const ss = require('simple-statistics');
const normal = ss.randomNormal(0, 1);
const binomial = ss.binomialDistribution(10, 0.5);
console.log(`Random Normal Value: ${normal}, Binomial Distribution: ${binomial}`);
Regression Analysis
This feature allows you to perform regression analysis, including linear regression. The code sample demonstrates how to compute a linear regression line for a given dataset.
const ss = require('simple-statistics');
const data = [[0, 1], [1, 2], [2, 3], [3, 4], [4, 5]];
const regression = ss.linearRegression(data);
const regressionLine = ss.linearRegressionLine(regression);
console.log(`Regression Line: y = ${regression.m}x + ${regression.b}`);
Hypothesis Testing
This feature provides functions for performing hypothesis testing, such as t-tests. The code sample shows how to perform a two-sample t-test on two datasets.
const ss = require('simple-statistics');
const tTest = ss.tTestTwoSample([1, 2, 3, 4, 5], [6, 7, 8, 9, 10]);
console.log(`T-Test Result: ${tTest}`);
jstat is a JavaScript library for statistical operations. It offers a wide range of statistical functions similar to simple-statistics, including descriptive statistics, probability distributions, and hypothesis testing. However, jstat has a more extensive API and supports matrix operations, making it suitable for more complex statistical analyses.
mathjs is a comprehensive mathematics library for JavaScript and Node.js. It includes a variety of mathematical functions, including statistical operations. While it is not solely focused on statistics like simple-statistics, it provides a broader range of mathematical tools, making it a versatile choice for projects that require both statistical and general mathematical computations.
ssjs (Statistical Software in JavaScript) is another library that provides statistical functions for JavaScript. It offers similar functionalities to simple-statistics, such as descriptive statistics and probability distributions. However, ssjs is less popular and has a smaller community compared to simple-statistics, which may affect the availability of support and updates.
A JavaScript implementation of descriptive, regression, and inference statistics.
Implemented in literate JavaScript with no dependencies, designed to work in all modern browsers (including IE) as well as in node.js.
Basic contracts of functions:
null
.Optionally mix in the following functions into the Array
prototype. Otherwise
you can use them off of the simple-statistics object itself.
If given a particular array instance as an argument, this adds the functions
only to that array rather than the global Array.prototype
. Without an argument,
it runs on the global Array.prototype
.
Mean of a single-dimensional Array of numbers. Also available as .average(x)
Sum of a single-dimensional Array of numbers.
Variance of a single-dimensional Array of numbers.
Standard Deviation of a single-dimensional Array of numbers.
The Median Absolute Deviation (MAD) is a robust measure of statistical dispersion. It is more resilient to outliers than the standard deviation. Accepts a single-dimensional array of numbers and returns a dispersion value.
Also aliased to .mad(x)
for brevity.
Median of a single-dimensional array of numbers.
Geometric mean of a single-dimensional array of positive numbers.
Harmonic mean of a single-dimensional array of positive numbers.
Finds the minimum of a single-dimensional array of numbers. This runs in linear O(n)
time.
Finds the maximum of a single-dimensional array of numbers. This runs in linear O(n)
time.
Does a student's t-test of a dataset sample
, represented by a single-dimensional array of numbers. x
is the known value, and the result is a measure of statistical significance.
The two-sample t-test is used to compare samples from two populations or groups, confirming or denying the suspicion (null hypothesis) that the populations are the same. It returns a t-value that you can then look up to give certain judgements of confidence based on a t distribution table.
This implementation expects the samples sample_x
and sample_y
to be given
as one-dimensional arrays of more than one number each.
Produces sample variance of a single-dimensional array of numbers.
Produces sample covariance of two single-dimensional arrays of numbers.
Produces sample correlation of two single-dimensional arrays of numbers.
Does a quantile of a dataset sample
,
at p. For those familiary with the k/q
syntax, p == k/q
. sample
must
be a single-dimensional array of numbers. p must be a number greater than or equal to
than zero and less or equal to than one, or an array of numbers following that rule.
If an array is given, an array of results will be returned instead of a single
number.
Does a quantile of a dataset sample
,
at p. sample
must be a one-dimensional sorted array of numbers, and
p
must be a single number from zero to one.
Calculates the Interquartile range of a sample - the difference between the upper and lower quartiles. Useful as a measure of dispersion.
Also available as .interquartile_range(x)
Calculates the skewness of a sample, a measure of the extent to which a probability distribution of a real-valued random variable "leans" to one side of the mean. The skewness value can be positive or negative, or even undefined.
This implementation uses the Fisher-Pearson standardized moment coefficient, which means that it behaves the same as Excel, Minitab, SAS, and SPSS.
Skewness is only valid for samples of over three values.
Find the Jenks Natural Breaks for
a single-dimensional array of numbers as input and a desired number_of_classes
.
The result is a single-dimensional with class breaks, including the minimum
and maximum of the input array.
Find the r-squared value of a particular dataset, expressed as a two-dimensional Array
of numbers, against a Function
.
var r_squared = ss.r_squared([[1, 1]], function(x) { return x * 2; });
Look up the given z
value in a standard normal table
to calculate the probability of a random variable appearing with a given value.
The standard score is the number of standard deviations an observation or datum is above or below the mean.
A standard normal table from which to pull values of Φ (phi).
Create a new linear regression solver.
Set the data of a linear regression. The input is a two-dimensional array of numbers, which are treated as coordinates, like [[x, y], [x1, y1]]
.
Get the linear regression line: this returns a function that you can
give x
values and it will return y
values. Internally, this uses the m()
and b()
values and the classic y = mx + b
equation.
var linear_regression_line = ss.linear_regression()
.data([[0, 1], [2, 2], [3, 3]]).line();
linear_regression_line(5);
Just get the slope of the fitted regression line, the m
component of the full
line equation. Returns a number.
Just get the y-intercept of the fitted regression line, the b
component
of the line equation. Returns a number.
Create a naïve bayesian classifier.
Train the classifier to classify a certain item, given as an object with keys, to be in a certain category, given as a string.
Get the classifications of a certain item, given as an object of
category -> score
mappings.
var bayes = ss.bayesian();
bayes.train({ species: 'Cat' }, 'animal');
bayes.score({ species: 'Cat' });
// { animal: 1 }
To use it in browsers, grab simple_statistics.js. To use it in node, install it with npm or add it to your package.json.
npm install simple-statistics
To use it with component,
component install tmcw/simple-statistics
To use it with bower,
bower install simple-statistics
// Require simple statistics
var ss = require('simple-statistics');
// The input is a simple array
var list = [1, 2, 3];
// Many different descriptive statistics are supported
var sum = ss.sum(list),
mean = ss.mean(list),
min = ss.min(list),
geometric_mean = ss.geometric_mean(list),
max = ss.max(list),
quantile = ss.quantile(0.25);
// For a linear regression, it's a two-dimensional array
var data = [ [1, 2], [2, 3] ];
// simple-statistics can produce a linear regression and return
// a friendly javascript function for the line.
var line = ss.linear_regression()
.data(data)
.line();
// get a point along the line function
line(0);
var line = ss.linear_regression()
// Get the r-squared value of the line estimation
ss.r_squared(data, line);
var bayes = ss.bayesian();
bayes.train({ species: 'Cat' }, 'animal');
bayes.score({ species: 'Cat' });
// { animal: 1 }
This is optional and not used by default. You can opt-in to mixins
with ss.mixin()
.
This mixes simple-statistics
methods into the Array prototype - note that
extending native objects is a
tricky move.
This will only work if defineProperty
is available, which means modern browsers
and nodejs - on IE8 and below, calling ss.mixin()
will throw an exception.
// mixin to Array class
ss.mixin();
// The input is a simple array
var list = [1, 2, 3];
// The same descriptive techniques as above, but in a simpler style
var sum = list.sum(),
mean = list.mean(),
min = list.min(),
max = list.max(),
quantile = list.quantile(0.25);
0.8.1
mode
that favored the last new numberFAQs
Simple Statistics
The npm package simple-statistics receives a total of 105,784 weekly downloads. As such, simple-statistics popularity was classified as popular.
We found that simple-statistics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.