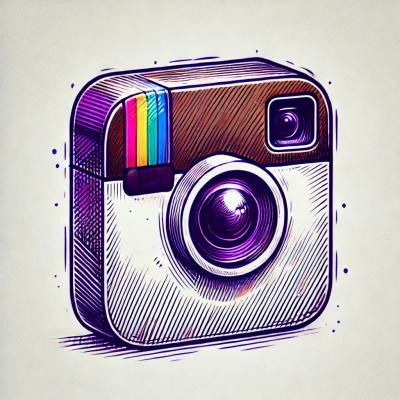
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
simplebar-react
Advanced tools
The simplebar-react package is a React wrapper for SimpleBar, a custom scrollbar library that aims to provide a simple and lightweight way to create custom scrollbars that look consistent across different browsers and platforms.
Custom Scrollbars
This feature allows you to create custom scrollbars for your content. The code sample demonstrates how to wrap your content with the SimpleBar component to apply custom scrollbars.
import React from 'react';
import SimpleBar from 'simplebar-react';
import 'simplebar-react/dist/simplebar.min.css';
const CustomScrollbar = () => (
<SimpleBar style={{ maxHeight: 300 }}>
<p>Your content here</p>
<p>Your content here</p>
<p>Your content here</p>
</SimpleBar>
);
export default CustomScrollbar;
Auto-hide Scrollbars
This feature allows the scrollbars to automatically hide when not in use. The code sample shows how to enable the auto-hide functionality by setting the autoHide prop to true.
import React from 'react';
import SimpleBar from 'simplebar-react';
import 'simplebar-react/dist/simplebar.min.css';
const AutoHideScrollbar = () => (
<SimpleBar autoHide={true} style={{ maxHeight: 300 }}>
<p>Your content here</p>
<p>Your content here</p>
<p>Your content here</p>
</SimpleBar>
);
export default AutoHideScrollbar;
Custom Scrollbar Styles
This feature allows you to apply custom styles to the scrollbars. The code sample demonstrates how to include a custom CSS file to style the scrollbars.
import React from 'react';
import SimpleBar from 'simplebar-react';
import 'simplebar-react/dist/simplebar.min.css';
import './customStyles.css';
const CustomStyledScrollbar = () => (
<SimpleBar style={{ maxHeight: 300 }}>
<p>Your content here</p>
<p>Your content here</p>
<p>Your content here</p>
</SimpleBar>
);
export default CustomStyledScrollbar;
react-custom-scrollbars is a highly customizable scrollbar component for React. It provides more flexibility in terms of styling and functionality compared to simplebar-react, but it may require more configuration.
react-scrollbars-custom is another React component for custom scrollbars. It offers a wide range of customization options and supports both vertical and horizontal scrolling. It is more feature-rich but also more complex to set up compared to simplebar-react.
rc-scrollbars is a lightweight and customizable scrollbar component for React. It is similar to simplebar-react in terms of simplicity and ease of use, but it may not offer as many customization options.
- Via npm
npm install simplebar-react --save
- Via Yarn
yarn add simplebar-react
Check out the Demo project or our live Codesandbox example.
If you are using a module loader (like Webpack) you first need to load SimpleBar:
import SimpleBar from 'simplebar-react';
import 'simplebar-react/dist/simplebar.min.css';
const App = () => (
<SimpleBar style={{ maxHeight: 300 }}>// your content</SimpleBar>
);
Don't forget to import both css and js in your project!
SimpleBar is not intended to be used on the body
element! I don't recommend wrapping your entire web page inside a custom scroll as it will often badly affect the user experience (slower scroll performance compared to the native body scroll, no native scroll behaviours like click on track, etc.). Do it at your own risk! SimpleBar is meant to improve the experience of internal web page scrolling; such as a chat box or a small scrolling area. Please read the caveats section first to be aware of the limitations!
If you are experiencing issues when setting up SimpleBar, it is most likely because your styles are clashing with SimpleBar ones. Make sure the element you are setting SimpleBar on does not override any SimpleBar css properties! We recommend to not style that element at all and use an inner element instead.
Thanks to BrowserStack for sponsoring open source projects and letting us test SimpleBar for free.
Find the list of available options on the core documentation.
<SimpleBar forceVisible="y" autoHide={false}>
// your content
</SimpleBar>
You can pass props to the underlying scrollable div
element. This is useful for example to get a ref
of it, if you want to access the scroll
event or apply imperative directive (like scrolling SimpleBar to the bottom, etc.).
const scrollableNodeRef = React.createRef();
<SimpleBar scrollableNodeProps={{ ref: scrollableNodeRef }}>
// your content
</SimpleBar>;
You can pass a ref to the SimpleBar element:
const ref = useRef();
useEffect(() => {
ref.current.recalculate();
console.log(ref.current.el); // <- the root element you applied SimpleBar on
})
<SimpleBar ref={ref}>
// your content
</SimpleBar>
For advanced usage, in some cases (like using react-window) you can forward the scrollable and content elements using a render prop pattern:
<SimpleBar>
{({ scrollableNodeRef, contentNodeRef }) => {
// Now you have access to scrollable and content nodes
return <div></div>;
}}
</SimpleBar>
If you define your own elements you should do:
<SimpleBar>
{({ scrollableNodeProps, contentNodeProps }) => {
return (
<div {...scrollableNodeProps}>
outer/scrollable element
<div {...contentNodeProps} />inner element</div>
</div>
);
}}
</SimpleBar>
FAQs
React component for SimpleBar
The npm package simplebar-react receives a total of 536,793 weekly downloads. As such, simplebar-react popularity was classified as popular.
We found that simplebar-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.