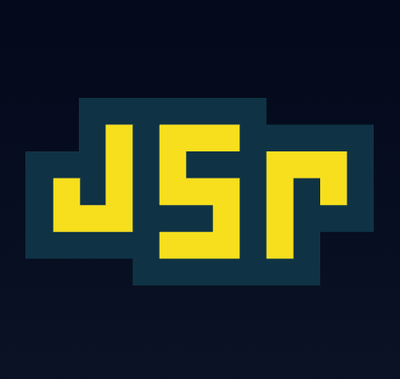
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Sinon is a testing utility that provides functions for spies, stubs, and mocks, which are essential for behavior-driven development and testing in JavaScript. It works with any unit testing framework and is widely used for its versatile API and comprehensive feature set.
Spies
Spies are functions that record their usage, such as how many times they were called, with what arguments, and what was returned. They can be used to wrap existing functions to add this tracking ability.
const sinon = require('sinon');
const myFunction = sinon.spy();
myFunction('Hello', 'World');
console.log(myFunction.calledOnce); // true
Stubs
Stubs are like spies, but they can replace the target function's behavior, either by returning a specific value or by throwing an exception. They are useful for controlling a function's behavior in a test.
const sinon = require('sinon');
const myObj = { myMethod: () => 'original' };
const stub = sinon.stub(myObj, 'myMethod').returns('stubbed');
console.log(myObj.myMethod()); // 'stubbed'
Mocks
Mocks are fake methods (like stubs) with pre-programmed behavior and expectations. They are used to assert that certain methods are called in certain ways.
const sinon = require('sinon');
const myObj = { myMethod: () => 'original' };
const mock = sinon.mock(myObj);
mock.expects('myMethod').once().returns('mocked');
console.log(myObj.myMethod()); // 'mocked'
mock.verify();
Jest is a complete testing framework that comes with spies, mocks, and stubs built-in. It is often compared to Sinon for its mocking capabilities, but Jest provides a more integrated experience with a test runner and assertion library included.
Testdouble.js (npm package 'testdouble') is a minimalistic testing library that provides a similar API to Sinon for creating test doubles like stubs, mocks, and spies. It focuses on providing a more user-friendly experience with better error messages and simpler APIs.
Chai-spies is a plugin for the Chai assertion library that adds spy capabilities. While it doesn't offer as comprehensive a set of features as Sinon, it integrates well with Chai for developers who prefer that assertion style.
Standalone and test framework agnostic JavaScript test spies, stubs and mocks.
via npm (node package manager)
$ npm install sinon
or install via git by cloning the repository and including sinon.js in your project, as you would any other third party library.
Don't forget to include the parts of Sinon.JS that you want to use as well (i.e. spy.js).
See the sinon project homepage
Pick an issue to fix, or pitch new features. To avoid wasting your time, please ask for feedback on feature suggestions either with an issue or on the mailing list.
The Sinon.JS developer environment requires Node/NPM. Please make sure you have Node installed, and install Sinon's dependencies:
$ npm install
$ npm test
Open test/sinon.html
in a browser. To test against a built distribution, first
make sure you have a build (requires Ruby and Juicer):
$ ./build
Then open test/sinon-dist.html
in a browser.
If the build script is unable to find Juicer, try
$ ruby -rubygems build
Some tests needs working XHR to pass. To run the tests over an HTTP server, run
$ node_modules/http-server/bin/http-server
Then open localhost:8080/test/sinon.html in a browser.
The Rhino tests are currently out of commission (pending update after switch to Buster.JS for tests).
1.7.1
FAQs
JavaScript test spies, stubs and mocks.
The npm package sinon receives a total of 5,018,878 weekly downloads. As such, sinon popularity was classified as popular.
We found that sinon demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.