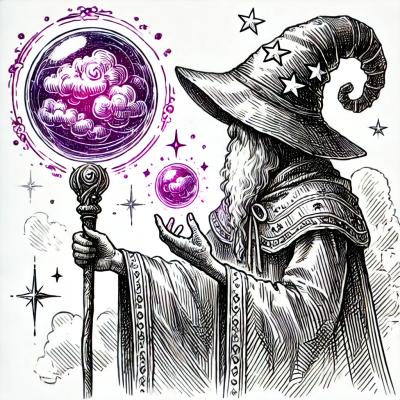
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Sinon is a testing utility that provides functions for spies, stubs, and mocks, which are essential for behavior-driven development and testing in JavaScript. It works with any unit testing framework and is widely used for its versatile API and comprehensive feature set.
Spies
Spies are functions that record their usage, such as how many times they were called, with what arguments, and what was returned. They can be used to wrap existing functions to add this tracking ability.
const sinon = require('sinon');
const myFunction = sinon.spy();
myFunction('Hello', 'World');
console.log(myFunction.calledOnce); // true
Stubs
Stubs are like spies, but they can replace the target function's behavior, either by returning a specific value or by throwing an exception. They are useful for controlling a function's behavior in a test.
const sinon = require('sinon');
const myObj = { myMethod: () => 'original' };
const stub = sinon.stub(myObj, 'myMethod').returns('stubbed');
console.log(myObj.myMethod()); // 'stubbed'
Mocks
Mocks are fake methods (like stubs) with pre-programmed behavior and expectations. They are used to assert that certain methods are called in certain ways.
const sinon = require('sinon');
const myObj = { myMethod: () => 'original' };
const mock = sinon.mock(myObj);
mock.expects('myMethod').once().returns('mocked');
console.log(myObj.myMethod()); // 'mocked'
mock.verify();
Jest is a complete testing framework that comes with spies, mocks, and stubs built-in. It is often compared to Sinon for its mocking capabilities, but Jest provides a more integrated experience with a test runner and assertion library included.
Testdouble.js (npm package 'testdouble') is a minimalistic testing library that provides a similar API to Sinon for creating test doubles like stubs, mocks, and spies. It focuses on providing a more user-friendly experience with better error messages and simpler APIs.
Chai-spies is a plugin for the Chai assertion library that adds spy capabilities. While it doesn't offer as comprehensive a set of features as Sinon, it integrates well with Chai for developers who prefer that assertion style.
Standalone and test framework agnostic JavaScript test spies, stubs and mocks (pronounced "sigh-non", named after Sinon, the warrior).
Do you wish that Sinon had better documentation?
So do we!
With your support, we can improve the documentation for everyone.
Thank you!
For details on compatibility and browser support, please see COMPATIBILITY.md
via npm
$ npm install sinon
or via sinon's browser builds available for download on the homepage. There are also npm based CDNs one can use.
See the sinon project homepage for documentation on usage.
If you have questions that are not covered by the documentation, you can check out the sinon
tag on Stack Overflow or drop by #sinon.js on irc.freenode.net:6667.
You can also search through the Sinon.JS mailing list archives.
See CONTRIBUTING.md for details on how you can contribute to Sinon.JS
Support us with a monthly donation and help us continue our activities. [Become a backer]
Become a sponsor and get your logo on our README on GitHub with a link to your site. [Become a sponsor]
Sinon.js was released under BSD-3
9.0.0
FAQs
JavaScript test spies, stubs and mocks.
The npm package sinon receives a total of 4,892,357 weekly downloads. As such, sinon popularity was classified as popular.
We found that sinon demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.