What is size-limit?
Size Limit is a performance monitoring tool for JavaScript projects. It helps you keep your project within a specific size limit by analyzing the size of your JavaScript bundles and providing warnings when the size exceeds the specified limit.
What are size-limit's main functionalities?
Bundle Size Analysis
This feature allows you to specify the path to your JavaScript bundle and set a size limit. Size Limit will analyze the bundle and ensure it does not exceed the specified limit.
module.exports = [
{
path: 'dist/bundle.js',
limit: '500 KB'
}
];
Custom Configurations
You can customize the analysis by enabling gzip compression or disabling the running of the bundle. This provides more flexibility in how you measure the size of your bundles.
module.exports = [
{
path: 'dist/bundle.js',
limit: '500 KB',
gzip: true,
running: false
}
];
Multiple Bundles
Size Limit supports analyzing multiple bundles in a single configuration. This is useful for projects with multiple entry points or output files.
module.exports = [
{
path: 'dist/bundle1.js',
limit: '300 KB'
},
{
path: 'dist/bundle2.js',
limit: '200 KB'
}
];
Other packages similar to size-limit
webpack-bundle-analyzer
Webpack Bundle Analyzer is a tool that visualizes the size of webpack output files with an interactive zoomable treemap. It provides a detailed breakdown of the bundle contents, making it easier to identify large modules and optimize the bundle size. Unlike Size Limit, it focuses more on visualization and detailed analysis rather than enforcing size limits.
bundlesize
Bundlesize is a tool that lets you set size limits for your JavaScript bundles and reports the size of the bundles in your CI/CD pipeline. It is similar to Size Limit in that it enforces size limits, but it integrates more tightly with CI/CD workflows and provides a simpler configuration.
source-map-explorer
Source Map Explorer analyzes JavaScript bundles using source maps to determine which files contribute to the bundle size. It provides a detailed breakdown of the bundle contents, similar to Webpack Bundle Analyzer, but it relies on source maps for its analysis. This makes it useful for understanding the impact of individual source files on the final bundle size.
Size Limit CLI Tool

Size Limit is a performance budget tool for JavaScript. It checks every commit
on CI, calculates the real cost of your JS for end-users and throws an error
if the cost exceeds the limit.
- ES modules and tree-shaking support.
- Add Size Limit to Travis CI, Circle CI, GitHub Actions
or another CI system to know if a pull request adds a massive dependency.
- Modular to fit different use cases: big JS applications
that use their own bundler or small npm libraries with many files.
- Can calculate the time it would take a browser
to download and execute your JS. Time is a much more accurate
and understandable metric compared to the size in bytes.
- Calculations include all dependencies and polyfills
used in your JS.
With GitHub action Size Limit will post bundle size changes as a comment
in pull request discussion.
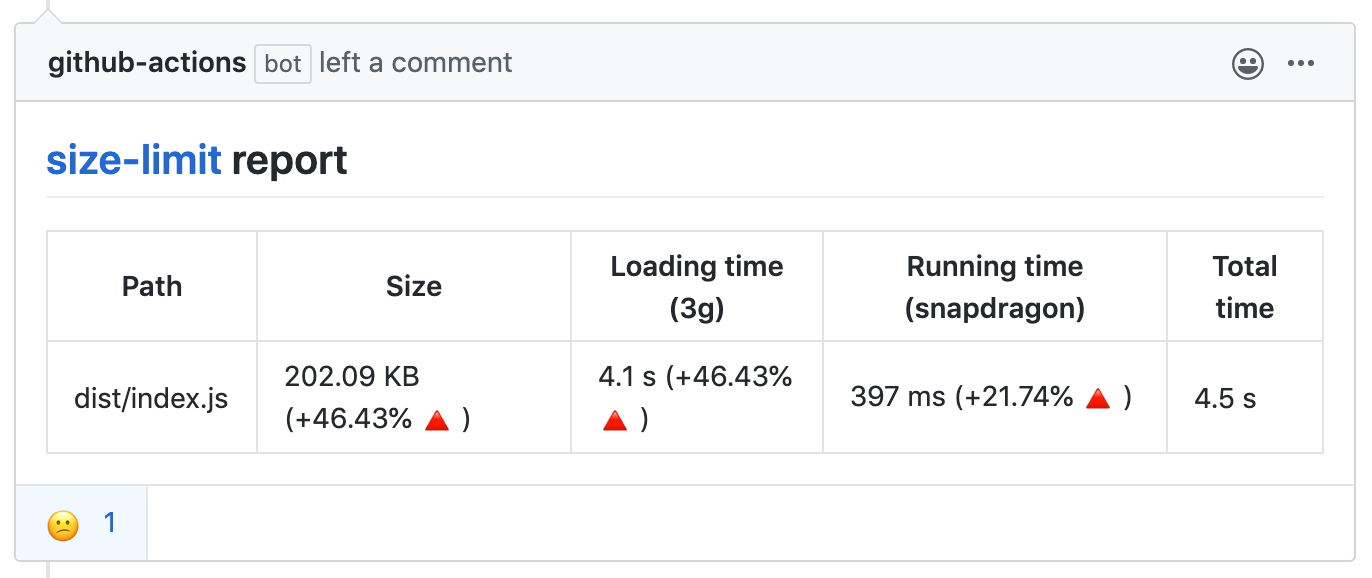
See full docs on GitHub.