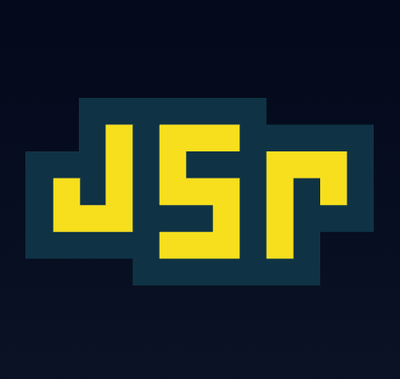
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
slate-react
Advanced tools
Tools for building completely customizable richtext editors with React.
The slate-react package is a highly customizable framework for building rich text editors in React applications. It provides a set of React components and hooks that allow developers to create complex text editing experiences with ease.
Basic Editor Setup
This code sets up a basic Slate editor with a single paragraph of text. The `Slate` component provides the context for the editor, and the `Editable` component renders the editable area.
import React, { useMemo } from 'react';
import { Slate, Editable, withReact } from 'slate-react';
import { createEditor } from 'slate';
const BasicEditor = () => {
const editor = useMemo(() => withReact(createEditor()), []);
const initialValue = [{ type: 'paragraph', children: [{ text: 'A line of text in a paragraph.' }] }];
return (
<Slate editor={editor} value={initialValue} onChange={value => {}}>
<Editable />
</Slate>
);
};
export default BasicEditor;
Custom Elements
This code demonstrates how to create custom elements in the Slate editor. In this example, a custom `quote` element is defined and rendered as a blockquote.
import React, { useMemo } from 'react';
import { Slate, Editable, withReact } from 'slate-react';
import { createEditor } from 'slate';
const CustomElement = ({ attributes, children, element }) => {
switch (element.type) {
case 'quote':
return <blockquote {...attributes}>{children}</blockquote>;
default:
return <p {...attributes}>{children}</p>;
}
};
const CustomEditor = () => {
const editor = useMemo(() => withReact(createEditor()), []);
const initialValue = [{ type: 'quote', children: [{ text: 'A line of text in a quote.' }] }];
return (
<Slate editor={editor} value={initialValue} onChange={value => {}}>
<Editable renderElement={props => <CustomElement {...props} />} />
</Slate>
);
};
export default CustomEditor;
Custom Leaf Nodes
This code shows how to create custom leaf nodes in the Slate editor. In this example, a custom `bold` leaf is defined and rendered as a strong element.
import React, { useMemo } from 'react';
import { Slate, Editable, withReact } from 'slate-react';
import { createEditor } from 'slate';
const CustomLeaf = ({ attributes, children, leaf }) => {
if (leaf.bold) {
children = <strong>{children}</strong>;
}
return <span {...attributes}>{children}</span>;
};
const CustomLeafEditor = () => {
const editor = useMemo(() => withReact(createEditor()), []);
const initialValue = [{ type: 'paragraph', children: [{ text: 'A bold line of text.', bold: true }] }];
return (
<Slate editor={editor} value={initialValue} onChange={value => {}}>
<Editable renderLeaf={props => <CustomLeaf {...props} />} />
</Slate>
);
};
export default CustomLeafEditor;
Draft.js is a framework for building rich text editors in React, developed by Facebook. It provides a set of immutable models and React components for creating complex text editing experiences. Compared to slate-react, Draft.js has a more opinionated architecture and may be less flexible for certain customizations.
Quill is a powerful, rich text editor built for the modern web. It offers a wide range of features out of the box, including formatting, themes, and modules for extending functionality. Quill is not React-specific, but it can be integrated with React applications using libraries like react-quill. Compared to slate-react, Quill provides more built-in features but may be less customizable.
ProseMirror is a toolkit for building rich text editors with a focus on extensibility and customizability. It provides a set of core modules that can be combined and extended to create complex text editing experiences. ProseMirror is not React-specific, but it can be integrated with React applications. Compared to slate-react, ProseMirror offers a more modular approach but may require more effort to set up and customize.
This package contains the React-specific logic for Slate. It's separated further into a series of directories:
Feel free to poke around in each of them to learn more!
FAQs
Tools for building completely customizable richtext editors with React.
The npm package slate-react receives a total of 684,077 weekly downloads. As such, slate-react popularity was classified as popular.
We found that slate-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.