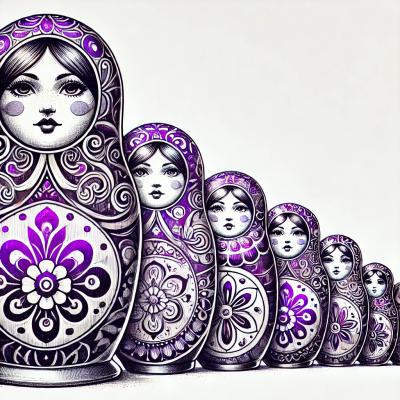
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The sntp npm package is a simple Network Time Protocol (NTP) client for Node.js. It allows you to synchronize your system time with NTP servers, ensuring accurate timekeeping for your applications.
Time Synchronization
This feature allows you to request the current time from an NTP server. The code sample demonstrates how to use the sntp package to get the current time from 'pool.ntp.org' and log it to the console.
const Sntp = require('sntp');
// Request the current time from an NTP server
Sntp.time({ host: 'pool.ntp.org', port: 123 }, (err, time) => {
if (err) {
console.error('Failed to get time:', err);
return;
}
console.log('Current time:', new Date(time.now));
});
Custom NTP Server
This feature allows you to specify a custom NTP server to synchronize time with. The code sample shows how to get the current time from 'time.google.com' and log it to the console.
const Sntp = require('sntp');
// Request the current time from a custom NTP server
Sntp.time({ host: 'time.google.com', port: 123 }, (err, time) => {
if (err) {
console.error('Failed to get time:', err);
return;
}
console.log('Current time from custom server:', new Date(time.now));
});
Time Offset
This feature allows you to calculate the time offset between your system time and the NTP server time. The code sample demonstrates how to get the time offset from 'pool.ntp.org' and log it to the console.
const Sntp = require('sntp');
// Request the current time and calculate the offset
Sntp.time({ host: 'pool.ntp.org', port: 123 }, (err, time) => {
if (err) {
console.error('Failed to get time:', err);
return;
}
console.log('Time offset:', time.t);
});
The ntp-client package is another NTP client for Node.js. It provides similar functionality to sntp, allowing you to synchronize your system time with NTP servers. However, ntp-client offers a simpler API and is easier to use for basic time synchronization tasks.
The ntp-time package is a Node.js module for retrieving the current time from NTP servers. It offers similar functionality to sntp, with a focus on providing accurate timekeeping for applications. It is a good choice if you need a reliable NTP client with minimal configuration.
An SNTP v4 client (RFC4330) for node. Simpy connects to the NTP or SNTP server requested and returns the server time
along with the roundtrip duration and clock offset. To adjust the local time to the NTP time, add the returned t
offset
to the local time.
Current version: 0.1.1
var Sntp = require('sntp');
// All options are optional
var options = {
host: 'nist1-sj.ustiming.org', // Defaults to pool.ntp.org
port: 123, // Defaults to 123 (NTP)
resolveReference: true, // Default to false (not resolving)
timeout: 1000 // Defaults to zero (no timeout)
};
// Request server time
Sntp.time(options, function (err, time) {
if (err) {
console.log('Failed: ' + err.message);
process.exit(1);
}
console.log('Local clock is off by: ' + time.t + ' milliseconds');
process.exit(0);
});
If an application needs to maintain continuous time synchronization, the module provides a stateful method for querying the current offset only when the last one is too old (defaults to daily).
// Request offset once
Sntp.offset(function (err, offset) {
console.log(offset); // New (served fresh)
// Request offset again
Sntp.offset(function (err, offset) {
console.log(offset); // Identical (served from cache)
});
});
To set a background offset refresh, start the interval and use the provided now() method. If for any reason the client fails to obtain an up-to-date offset, the current system clock is used.
var before = Sntp.now(); // System time without offset
Sntp.start(function () {
var now = Sntp.now(); // With offset
Sntp.stop();
});
FAQs
SNTP Client
The npm package sntp receives a total of 1,646,988 weekly downloads. As such, sntp popularity was classified as popular.
We found that sntp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.