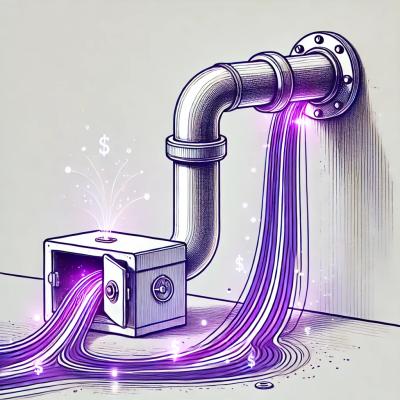
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A utility to manage one socket per user with Redis persistence for scalable WebSocket applications.
SockManage is a utility library for managing WebSocket connections, ensuring each user has a single active socket connection at any given time. It uses Redis for persistence, making it suitable for scalable and distributed WebSocket applications.
To install with npm:
npm install sockmanage
or with Yarn:
yarn add sockmanage
import { createClient } from "redis";
import { Server as SocketIOServer } from "socket.io";
import { SockManage } from "sockmanage";
// Initialize Redis and Socket.IO
const redisClient = createClient();
const io = new SocketIOServer(server); // assume 'server' is an HTTP server
// Initialize SockManage
const socketManager = new SockManage({ redis: redisClient });
// Set up the Socket.IO server and specify the namespace (optional)
socketManager.setup({ io, namespace: "/your-namespace" });
// Assuming top-level await is supported, otherwise wrap the following line in an async function
await socketManager.initialize();
setup
Sets up Socket.IO server and optional namespace.
socketManager.setup({ io, namespace: "/your-namespace" });
Parameters:
io
: Instance of SocketIOServer
.namespace
(optional): Namespace for Socket.IO events.initialize
Initializes user sockets from Redis.
await socketManager.initialize();
getSockets
Retrieves all user sockets from local map.
const userSockets = socketManager.getSockets();
Returns: Map<string, string>
: A map of user IDs to socket IDs, or an empty map.
getSocket
Retrieves the socket ID for a specific user.
const socketId = socketManager.getSocket("userId");
Parameters:
userId
: ID of the user.Returns: string | null
: Socket ID of the user or null
if not found.
register
Registers a socket for a user and ensures only one active socket per user.
Note: The data
parameter must be a JSON string containing the userId
.
await socketManager.register(socket, JSON.stringify({ userId: "user1" }));
Parameters:
socket
: The socket instance.data
: A JSON string containing the userId
.deRegister
De-registers a socket for a user.
socketManager.deRegister(socket);
Parameters:
socket
: The socket instance to deregister.inform
Emits an event to a specific socket.
socketManager.inform({
socketId: "socket1",
_event: "message",
data: { message: "Hello, User!" },
});
Parameters:
socketId
: ID of the socket to emit the event to._event
: Event name.data
: Data to send with the event.import { createClient } from "redis";
import { Server as SocketIOServer } from "socket.io";
import { SockManage } from "sockmanage";
const redisClient = createClient();
const io = new SocketIOServer(server);
const socketManager = new SockManage({ redis: redisClient });
socketManager.setup({ io });
// Assuming top-level await is supported, otherwise wrap the following line in an async function
await socketManager.initialize();
io.on("connection", (socket) => {
// You be getting the userId from anywhere, it doesn't matter where you get it from
// as long as you pass it to the register method.
const userId = socket.handshake.query.userId;
socketManager.register(socket, JSON.stringify({ userId }));
socket.on("disconnect", () => {
socketManager.deRegister(socket);
});
// this following block is completely optional, you shall proceed with using your own event sending logic
socket.on("message", (data) => {
socketManager.inform({
socketId: socket.id,
_event: "message",
data: { message: "Hello, User!" },
});
});
});
To run the tests, use the following command:
yarn test
Detailed changes for each version are documented in the History.md file.
MIT License. See LICENSE for details.
FAQs
A utility to manage one socket per user with Redis persistence for scalable WebSocket applications.
The npm package sockmanage receives a total of 4 weekly downloads. As such, sockmanage popularity was classified as not popular.
We found that sockmanage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.