Comparing version 0.10.2 to 0.10.3-alpha.0
@@ -1,3 +0,3 @@ | ||
import { Sofa } from './sofa'; | ||
import { ContextValue } from './types'; | ||
import type { Sofa } from './sofa'; | ||
import type { ContextValue } from './types'; | ||
export declare type ErrorHandler = (errors: ReadonlyArray<any>) => RouterError; | ||
@@ -4,0 +4,0 @@ declare type RouterRequest = { |
@@ -381,2 +381,3 @@ 'use strict'; | ||
function createQueryRoute({ sofa, router, fieldName, }) { | ||
var _a, _b, _c, _d; | ||
logger.debug(`[Router] Creating ${fieldName} query`); | ||
@@ -402,18 +403,19 @@ const queryType = sofa.schema.getQueryType(); | ||
const hasIdArgument = field.args.some((arg) => arg.name === 'id'); | ||
const path = getPath(fieldName, isSingle && hasIdArgument); | ||
const method = produceMethod({ | ||
typeName: queryType.name, | ||
fieldName, | ||
methodMap: sofa.method, | ||
defaultValue: 'GET', | ||
}); | ||
router[method.toLocaleLowerCase()](path, useHandler({ info, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} query available at ${method} ${path}`); | ||
const graphqlPath = `${queryType.name}.${fieldName}`; | ||
const routeConfig = (_a = sofa.routes) === null || _a === void 0 ? void 0 : _a[graphqlPath]; | ||
const route = { | ||
method: (_b = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.method) !== null && _b !== void 0 ? _b : 'GET', | ||
path: (_c = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.path) !== null && _c !== void 0 ? _c : getPath(fieldName, isSingle && hasIdArgument), | ||
responseStatus: (_d = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.responseStatus) !== null && _d !== void 0 ? _d : 200, | ||
}; | ||
router[route.method.toLocaleLowerCase()](route.path, useHandler({ info, route, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} query available at ${route.method} ${route.path}`); | ||
return { | ||
document: operation, | ||
path, | ||
method: method.toUpperCase(), | ||
path: route.path, | ||
method: route.method.toUpperCase(), | ||
}; | ||
} | ||
function createMutationRoute({ sofa, router, fieldName, }) { | ||
var _a, _b, _c, _d; | ||
logger.debug(`[Router] Creating ${fieldName} mutation`); | ||
@@ -434,10 +436,11 @@ const mutationType = sofa.schema.getMutationType(); | ||
const info = getOperationInfo(operation); | ||
const path = getPath(fieldName); | ||
const method = produceMethod({ | ||
typeName: mutationType.name, | ||
fieldName, | ||
methodMap: sofa.method, | ||
defaultValue: 'POST', | ||
}); | ||
router[method.toLowerCase()](path, useHandler({ info, fieldName, sofa, operation })); | ||
const graphqlPath = `${mutationType.name}.${fieldName}`; | ||
const routeConfig = (_a = sofa.routes) === null || _a === void 0 ? void 0 : _a[graphqlPath]; | ||
const route = { | ||
method: (_b = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.method) !== null && _b !== void 0 ? _b : 'POST', | ||
path: (_c = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.path) !== null && _c !== void 0 ? _c : getPath(fieldName), | ||
responseStatus: (_d = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.responseStatus) !== null && _d !== void 0 ? _d : 200, | ||
}; | ||
const { method, path } = route; | ||
router[method.toLowerCase()](path, useHandler({ info, route, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} mutation available at ${method} ${path}`); | ||
@@ -447,3 +450,3 @@ return { | ||
path, | ||
method: method.toUpperCase(), | ||
method, | ||
}; | ||
@@ -487,3 +490,3 @@ } | ||
type: 'result', | ||
status: 200, | ||
status: config.route.responseStatus, | ||
body: result.data && result.data[fieldName], | ||
@@ -508,9 +511,2 @@ }; | ||
} | ||
function produceMethod({ typeName, fieldName, methodMap, defaultValue, }) { | ||
const path = `${typeName}.${fieldName}`; | ||
if (methodMap && methodMap[path]) { | ||
return methodMap[path]; | ||
} | ||
return defaultValue; | ||
} | ||
@@ -517,0 +513,0 @@ function createSofa(config) { |
@@ -375,2 +375,3 @@ import { __awaiter, __asyncValues, __rest } from 'tslib'; | ||
function createQueryRoute({ sofa, router, fieldName, }) { | ||
var _a, _b, _c, _d; | ||
logger.debug(`[Router] Creating ${fieldName} query`); | ||
@@ -396,18 +397,19 @@ const queryType = sofa.schema.getQueryType(); | ||
const hasIdArgument = field.args.some((arg) => arg.name === 'id'); | ||
const path = getPath(fieldName, isSingle && hasIdArgument); | ||
const method = produceMethod({ | ||
typeName: queryType.name, | ||
fieldName, | ||
methodMap: sofa.method, | ||
defaultValue: 'GET', | ||
}); | ||
router[method.toLocaleLowerCase()](path, useHandler({ info, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} query available at ${method} ${path}`); | ||
const graphqlPath = `${queryType.name}.${fieldName}`; | ||
const routeConfig = (_a = sofa.routes) === null || _a === void 0 ? void 0 : _a[graphqlPath]; | ||
const route = { | ||
method: (_b = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.method) !== null && _b !== void 0 ? _b : 'GET', | ||
path: (_c = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.path) !== null && _c !== void 0 ? _c : getPath(fieldName, isSingle && hasIdArgument), | ||
responseStatus: (_d = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.responseStatus) !== null && _d !== void 0 ? _d : 200, | ||
}; | ||
router[route.method.toLocaleLowerCase()](route.path, useHandler({ info, route, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} query available at ${route.method} ${route.path}`); | ||
return { | ||
document: operation, | ||
path, | ||
method: method.toUpperCase(), | ||
path: route.path, | ||
method: route.method.toUpperCase(), | ||
}; | ||
} | ||
function createMutationRoute({ sofa, router, fieldName, }) { | ||
var _a, _b, _c, _d; | ||
logger.debug(`[Router] Creating ${fieldName} mutation`); | ||
@@ -428,10 +430,11 @@ const mutationType = sofa.schema.getMutationType(); | ||
const info = getOperationInfo(operation); | ||
const path = getPath(fieldName); | ||
const method = produceMethod({ | ||
typeName: mutationType.name, | ||
fieldName, | ||
methodMap: sofa.method, | ||
defaultValue: 'POST', | ||
}); | ||
router[method.toLowerCase()](path, useHandler({ info, fieldName, sofa, operation })); | ||
const graphqlPath = `${mutationType.name}.${fieldName}`; | ||
const routeConfig = (_a = sofa.routes) === null || _a === void 0 ? void 0 : _a[graphqlPath]; | ||
const route = { | ||
method: (_b = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.method) !== null && _b !== void 0 ? _b : 'POST', | ||
path: (_c = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.path) !== null && _c !== void 0 ? _c : getPath(fieldName), | ||
responseStatus: (_d = routeConfig === null || routeConfig === void 0 ? void 0 : routeConfig.responseStatus) !== null && _d !== void 0 ? _d : 200, | ||
}; | ||
const { method, path } = route; | ||
router[method.toLowerCase()](path, useHandler({ info, route, fieldName, sofa, operation })); | ||
logger.debug(`[Router] ${fieldName} mutation available at ${method} ${path}`); | ||
@@ -441,3 +444,3 @@ return { | ||
path, | ||
method: method.toUpperCase(), | ||
method, | ||
}; | ||
@@ -481,3 +484,3 @@ } | ||
type: 'result', | ||
status: 200, | ||
status: config.route.responseStatus, | ||
body: result.data && result.data[fieldName], | ||
@@ -502,9 +505,2 @@ }; | ||
} | ||
function produceMethod({ typeName, fieldName, methodMap, defaultValue, }) { | ||
const path = `${typeName}.${fieldName}`; | ||
if (methodMap && methodMap[path]) { | ||
return methodMap[path]; | ||
} | ||
return defaultValue; | ||
} | ||
@@ -511,0 +507,0 @@ function createSofa(config) { |
{ | ||
"name": "sofa-api", | ||
"version": "0.10.2", | ||
"version": "0.10.3-alpha.0", | ||
"description": "Create REST APIs with GraphQL", | ||
@@ -43,2 +43,2 @@ "sideEffects": false, | ||
} | ||
} | ||
} |
@@ -32,3 +32,3 @@ [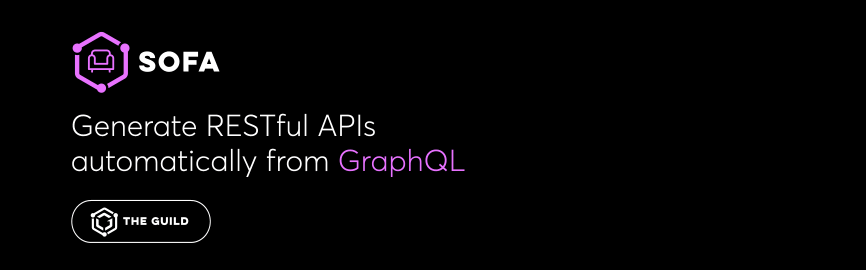](https://sofa-api.com) | ||
try { | ||
consr response = await invokeSofa({ | ||
const response = await invokeSofa({ | ||
method: req.method, | ||
@@ -38,3 +38,3 @@ url: req.url, | ||
contextValue: { | ||
req | ||
req, | ||
}, | ||
@@ -181,5 +181,5 @@ }); | ||
### Customize endpoint's HTTP Method | ||
### Customize endpoint's HTTP Method, path and response status code | ||
Sofa allows you to cutomize the http method. For example, in case you need `POST` instead of `GET` method in one of your query, you do the following: | ||
Sofa allows you to cutomize the http method, path and response status. For example, in case you need `POST` instead of `GET` method in one of your query, you do the following: | ||
@@ -191,4 +191,4 @@ ```typescript | ||
schema, | ||
method: { | ||
'Query.feed': 'POST', | ||
routes: { | ||
'Query.feed': { method: 'POST' }, | ||
}, | ||
@@ -203,2 +203,4 @@ }) | ||
You can also specify `path` with dynamic params support (for example `/feed/:offset/:limit`) and `responseStatus`. | ||
### Custom depth limit | ||
@@ -205,0 +207,0 @@ |
import { GraphQLSchema } from 'graphql'; | ||
import { Ignore, ExecuteFn, OnRoute, MethodMap } from './types'; | ||
import { Ignore, ExecuteFn, OnRoute, Method } from './types'; | ||
import { ErrorHandler } from './express'; | ||
interface RouteConfig { | ||
method?: Method; | ||
path?: string; | ||
responseStatus?: number; | ||
} | ||
export interface Route { | ||
method: Method; | ||
path: string; | ||
responseStatus: number; | ||
} | ||
export interface SofaConfig { | ||
@@ -17,6 +27,5 @@ basePath: string; | ||
/** | ||
* Overwrites the default HTTP method. | ||
* @example {"Query.field": "GET", "Mutation.field": "POST"} | ||
* Overwrites the default HTTP route. | ||
*/ | ||
method?: MethodMap; | ||
routes?: Record<string, RouteConfig>; | ||
} | ||
@@ -29,3 +38,3 @@ export interface Sofa { | ||
depthLimit: number; | ||
method?: MethodMap; | ||
routes?: Record<string, RouteConfig>; | ||
execute: ExecuteFn; | ||
@@ -36,1 +45,2 @@ onRoute?: OnRoute; | ||
export declare function createSofa(config: SofaConfig): Sofa; | ||
export {}; |
@@ -12,2 +12,1 @@ import { GraphQLArgs, ExecutionResult, DocumentNode } from 'graphql'; | ||
export declare type OnRoute = (info: RouteInfo) => void; | ||
export declare type MethodMap = Record<string, Method>; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
220597
2308
378