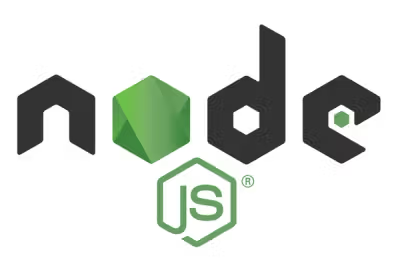
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
solid-google-maps
Advanced tools
SolidJS components and hooks for the Google Maps Javascript API
Solid Google Maps provides a loader and simple reactive bindings for the Google Maps API
Demo and examples: https://solid-google-maps.vercel.app/
pnpm install solid-google-maps
import { APIProvider, Map } from 'solid-google-maps'
const BasicExample = () => {
return (
<APIProvider apiKey={API_KEY}>
<Map
style={{ height: '500px', width: '100%' }}
defaultZoom={3}
defaultCenter={{ lat: 22.54992, lng: 0 }}
gestureHandling={'greedy'}
disableDefaultUI={true}
/>
</APIProvider>
)
}
import { APIProvider, Map } from 'solid-google-maps'
const MarkerExample = () => {
return (
<APIProvider apiKey={API_KEY}>
<Map
style={{ height: '500px', width: '100%' }}
defaultZoom={3}
defaultCenter={{ lat: 22.54992, lng: 0 }}
gestureHandling={'greedy'}
disableDefaultUI={true}
>
<AdvancedMarker position={{ lat: 22.54992, lng: 0 }}>
<CustomIcon />
</AdvancedMarker>
</Map>
</APIProvider>
)
}
import { APIProvider, Map, InfoWindow } from 'solid-google-maps'
const MarkerExample = () => {
const [infoWindowOpen, setInfoWindowOpen] = createSignal(false)
return (
<APIProvider apiKey={API_KEY}>
<Map
style={{ height: '500px', width: '100%' }}
defaultZoom={3}
defaultCenter={{ lat: 22.54992, lng: 0 }}
gestureHandling={'greedy'}
disableDefaultUI={true}
>
<AdvancedMarker position={{ lat: 22.54992, lng: 0 }} onClick={() => setInfoWindowOpen(!infoWindowOpen())}>
<CustomIcon />
<InfoWindow
open={infoWindowOpen()}
onOpenChange={setInfoWindowOpen}
maxWidth={200}
headerContent={<span>Header</span>}
>
InfoWindow Content
</InfoWindow>
</AdvancedMarker>
</Map>
</APIProvider>
)
}
import { APIProvider, Map, InfoWindow } from 'solid-google-maps'
const AnchorExample = () => {
const [anchor, setAnchor] = createSignal<google.maps.marker.AdvancedMarker | null>(null)
return (
<APIProvider apiKey={API_KEY}>
<Map
style={{ height: '500px', width: '100%' }}
defaultZoom={3}
defaultCenter={{ lat: 22.54992, lng: 0 }}
gestureHandling={'greedy'}
disableDefaultUI={true}
>
<AdvancedMarker position={{ lat: 21, lng: 0 }} onClick={(event) => setAnchor(event.marker)}>
<CustomIcon />
</AdvancedMarker>
<AdvancedMarker position={{ lat: 22, lng: 0 }} onClick={(event) => setAnchor(event.marker)}>
<CustomIcon />
</AdvancedMarker>
<Show when={anchor()}>
<InfoWindow anchor={anchor()} maxWidth={200} headerContent={<span>Header</span>}>
InfoWindow Content
</InfoWindow>
</Show>
</Map>
</APIProvider>
)
}
All Components have a ref
property that provides direct access to the Google Maps API. Additionally, the library provides a useMap()
method that can be used to get a reference to the API. If you have multiple maps you can provide them an id
prop and then use the ID in useMap(id)
to specify the map you want to access. useMap()
must be used inside the APIProvider
component.
First, install dependencies and Playwright browsers:
pnpm install
pnpm playwright install
Then ensure you've built the library:
pnpm build
Then run the tests using your local build against real browser engines:
pnpm test
FAQs
SolidJS components and hooks for the Google Maps Javascript API
The npm package solid-google-maps receives a total of 7 weekly downloads. As such, solid-google-maps popularity was classified as not popular.
We found that solid-google-maps demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.