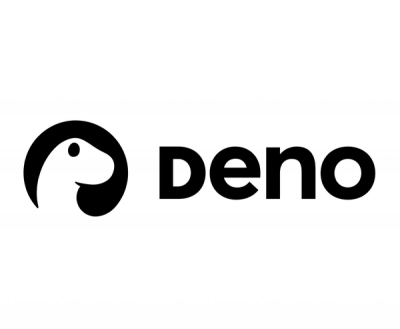
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
能動的音楽鑑賞サービス Songle (ソングル)の機能や解析結果をあなたのアプリケーションから利用することができるアプリケーション開発者向け API です。 Songle API の提供する機能を組み合わせることで、ウェブ上の好きな音楽の再生に合わせて動作するアプリケーションを容易に開発することができます。大規模音楽連動制御プラットフォーム Songle Sync (ソングルシンク)の機能を使う場合も、こちらをご利用ください。
詳細な使い方は api.songle.jp よりご確認ください。
Songle API モジュールは、次の環境で動作することが確認されています。
Songle API モジュールは script
タグもしくは npm
などのパッケージマネージャからインストールすることができます。
<script src="https://api.songle.jp/v2/api.js"></script>
$ npm install songle-api
ウェブブラウザから Songle API モジュールを利用した場合、その利用準備の完了を通知する onSongleAPIReady
が Songle API モジュールによって自動的に呼び出されます。
self.onSongleAPIReady =
function(Songle) {
alert("Hello Songle API !!");
}
次のサンプルプログラムは、音楽の拍子と連動するプログラムです。サンプルからも分かるように、音楽に連動したイベント駆動のプログラムを容易に記述することができます。
self.onSongleAPIReady =
function(Songle) {
var player =
new Songle.Player({
mediaElement: "#songle"
});
player.addPlugin(new Songle.Plugin.Beat());
player.useMedia("https://www.youtube.com/watch?v=zweVJrnE1uY");
player.on("beatPlay",
function(ev) {
switch(ev.data.beat.position) {
case 1:
console.log("1st beat !!");
break;
case 2:
console.log("2nd beat !!");
break;
case 3:
console.log("3rd beat !!")
break;
case 4:
console.log("4th beat !!");
break;
}
});
}
ウェブ上の音楽の再生に合わせて多種多様な機器を同時制御することで、一体感のある演出ができる大規模音楽連動制御プラットフォームです。誰でも自分のスマートフォンやパソコン等の機器を自由自在に組み合わせて、好きな楽曲の再生に合わせて光ったり動いたりする演出を作ることができます。
この機能を使うためには Songle API のウェブサイトからユーザ登録を行い、トークンを取得する必要があります。取得方法の詳細はチュートリアルのステップ3を参照してください。
チュートリアルサイトでは、より実践的な使い方を実際に体験しながら学ぶことができます。チュートリアルの内容は、アプリケーション開発者をメインターゲットとしていますが、途中までは、プログラミングの知識がない方でもより高度な大規模音楽連動制御を楽しみながら体験できます。
次のサンプルプログラムは、サンプルプログラム1を大規模音楽連動制御に対応させたプログラムです。取得したトークンを記述して複数のブラウザからマスターとスレーブを実行してみて、イメージを掴んでみましょう。
self.onSongleAPIReady =
function(Songle) {
var player =
new Songle.SyncPlayer({
accessToken: "YOUR-ACCESS-TOKEN-HERE", // Please edit your access token
secretToken: "YOUR-SECRET-TOKEN-HERE", // Please edit your secret token
mediaElement: "#songle"
});
player.addPlugin(new Songle.Plugin.Beat());
player.useMedia("https://www.youtube.com/watch?v=zweVJrnE1uY");
player.on("beatPlay",
function(ev) {
switch(ev.data.beat.position) {
case 1:
console.log("1st beat !!");
break;
case 2:
console.log("2nd beat !!");
break;
case 3:
console.log("3rd beat !!")
break;
case 4:
console.log("4th beat !!");
break;
}
});
}
self.onSongleAPIReady =
function(Songle) {
var player =
new Songle.SyncPlayer({
accessToken: "YOUR-ACCESS-TOKEN-HERE", // Please edit your access token
mediaElement: "#songle"
});
player.addPlugin(new Songle.Plugin.Beat());
player.useMedia("https://www.youtube.com/watch?v=zweVJrnE1uY");
player.on("beatPlay",
function(ev) {
switch(ev.data.beat.position) {
case 1:
console.log("1st beat !!");
break;
case 2:
console.log("2nd beat !!");
break;
case 3:
console.log("3rd beat !!")
break;
case 4:
console.log("4th beat !!");
break;
}
});
}
次のサンプルプログラムは Node.js 上で動作するスレーブのサンプルです。
var Songle = require("songle-api");
var player =
new Songle.SyncPlayer({
accessToken: "YOUR-ACCESS-TOKEN-HERE", // please edit your access token
});
player.addPlugin(new Songle.Plugin.Beat());
player.on("beatPlay",
function(ev) {
switch(ev.data.beat.position) {
case 1:
console.log("1st beat !!");
break;
case 2:
console.log("2nd beat !!");
break;
case 3:
console.log("3rd beat !!")
break;
case 4:
console.log("4th beat !!");
break;
}
});
Songle API を利用するためには、利用規約に同意する必要があります。
Copyright (c) 2017-2019 AIST Songle Project
FAQs
Songle API client library
We found that songle-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.