What is source-map-url?
The source-map-url npm package is a utility for working with source map URLs. It allows you to extract, insert, or remove source map URLs within a file, typically JavaScript or CSS files. This can be useful for developers who need to manage source map links in their generated code.
What are source-map-url's main functionalities?
Extracting source map URL
This feature allows you to extract the source map URL from a string of code. The `getFrom` method returns the URL if it exists.
const sourceMapUrl = require('source-map-url');
const code = '/*# sourceMappingURL=/path/to/file.js.map */';
const extractedUrl = sourceMapUrl.getFrom(code);
Inserting source map URL
This feature enables you to insert a source map URL into a string of code. The `insertInto` method adds the specified URL at the end of the code.
const sourceMapUrl = require('source-map-url');
const code = 'function example() {}';
const newCode = sourceMapUrl.insertInto(code, '/path/to/file.js.map');
Removing source map URL
This feature is used to remove an existing source map URL from a string of code. The `removeFrom` method strips out the source map URL.
const sourceMapUrl = require('source-map-url');
const code = 'function example() {}\n/*# sourceMappingURL=/path/to/file.js.map */';
const newCode = sourceMapUrl.removeFrom(code);
Other packages similar to source-map-url
source-map
The 'source-map' package provides utilities for generating and consuming source maps. It is more feature-rich than 'source-map-url', offering functionality for creating, parsing, and manipulating source maps in addition to handling URLs.
convert-source-map
The 'convert-source-map' package is similar to 'source-map-url' but with additional capabilities for converting source maps between different formats, such as from inline to external or vice versa.
Overview 
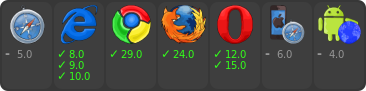
Tools for working with sourceMappingURL comments.
var sourceMappingURL = require("source-map-url")
var code = [
"!function(){...}();",
"/*# sourceMappingURL=foo.js.map */"
].join("\n")
sourceMappingURL.get(code)
code = sourceMappingURL.set(code, "/other/file.js.map")
code = sourceMappingURL.insertBefore(code, "\n// License: MIT")
code = sourceMappingURL.remove(code)
sourceMappingURL.get(code)
Installation
npm install source-map-url
bower install source-map-url
component install lydell/source-map-url
Works with CommonJS, AMD and browser globals, through UMD.
Usage
sourceMappingURL.get(code)
Returns the url of the sourceMappingURL comment in code
. Returns null
if
there is no such comment. Note that the url can be the empty string and that
both the empty string and null
are falsy. Consider using if (url === null) {}
rather than if (url) {}
if you need to tell those two cases apart.
Updates the sourceMappingURL comment in code
to use url
. Creates such a
comment if there is none. Returns the updated code
.
The commentSyntax
argument is optional. It is an array. The first element of
it defines how a comment starts, while the second element defines how it ends.
The default value is ["/*", " */"]
. /**/
comments were chosen as default in
favor of //
comments, because ideally they work with both JavaScript and CSS.
This way, you don’t have to think about what type of code you’re working with.
However, Chrome sadly does not support /**/
comments in JavaScript. So
currently, you need to use .set(code, url, ["//"])
for JavaScript that needs
source map support in Chrome. A bug has been filed about this.
sourceMappingURL.remove(code)
Removes the sourceMappingURL comment in code
. Does nothing if there is no
such comment. Returns the updated code
.
sourceMappingURL.insertBefore(code, string)
Inserts string
before the sourceMappingURL comment in code
. Appends
string
to code
if there is no such comment.
Lets you append something to a file without worrying about breaking the
sourceMappingURL comment (which needs to be at the end of the file).
sourceMappingURL.regex
The regex that is used to match sourceMappingURL comments. It matches both //
and /**/
comments, thus supporting both JavaScript and CSS.
Lets you create a new SourceMappingURL instance, using commentSyntax
as
default comment syntax for the set
method.
For example, if you mainly work with JavaScript code that needs to have source
map support in Chrome, you could use it like this:
var SourceMappingURL = require("source-map-url").SourceMappingURL
var sourceMappingURL = new SourceMappingURL(["//"])
License
The X11 (“MIT”) License.