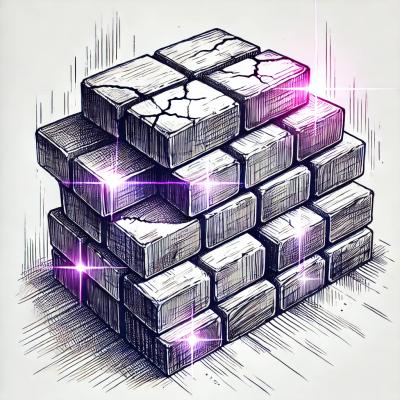
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
TypeScript toolset to simplify building peer-to-peer applications using IPFS, Ethereum, and OrbitDb
A TypeScript toolset to simplify building peer-to-peer smart contract applications using Ethereum, IPFS, and OrbitDB. All the tools you'll need to get started quickly. Smart contracts, storage, MVC, and unit tests with a proven MVC pattern inspired by enterprise Java/.NET libraries like Spring.
SpaceMVC provides a pre-configured development environment with reasonable defaults. Start building real apps immediately.
The fastest way to create a new project is to clone the SpaceMVC Starter project. Creates a super basic CRUD app to save and load lists of students for a class roster.
//TODO: this will become a truffle box or similar.
import "core-js/stable"
import "regenerator-runtime/runtime"
import SpaceMVC from "space-mvc";
// Set up Inversify container with dependencies.
//Example bindings. See iversify docs for full details. You'll bind your controllers, services, and DAOs here.
import { FranchiseService } from "./service/franchise-service" //just an example of a service
import { LeagueController } from "./controller/league-controller" //just ann example of a controller.
const container = new Container()
container.bind(FranchiseService).toSelf().inSingletonScope()
container.bind(HomeController).toSelf().inSingletonScope()
/**
* Create Framework 7 init params.
*
* See Framework7 documentation for available options: https://framework7.io/docs/app.html
*
* Please note that the routes field is optional.
* SpaceMVC maps routes with controller annotations.
* Passing a value will disable that mechanism.
*
*/
let f7Config = {
root: '#app', // App root element
id: 'example', // App bundle ID
name: 'SpaceMVC Example', // App name
theme: 'auto', // Automatic theme detection
}
/**
* Configure the Orbit DB store definitions that we'll open.
* For each StoreDefinition it will open an OrbitDB store.
*
* ownerAddress is the first user that will have permission to write
* to each store when it's opened. Later you can use the OrbitDB API to
* add more user permissions to it.
*/
let storeDefinitions:StoreDefinition[] = [{
name: "player",
type: "mfsstore",
load: 100,
options: {
schema: {
id: { unique: true },
firstName: { unique: false },
lastName: { unique: false }
}
}
}]
/*
* Using a truffle contract as an example.
Contracts are available by injecting the ContractService into your services or DAOs.
Call getContract(name) to get the initialized ethers contract.
*/
let truffleJson = require('../truffle/build/contracts/YourContract.json')
let contract:Contract = await ContractService.createContractFromTruffle(truffleJson)
/**
* By default the app will connect the "homestead" ethers provider.
* You can override this by passing a value for defaultProvider
*/
let spaceMvcInit:SpaceMvcInit = {
container: container,
f7Config: f7Config,
storeDefinitions: storeDefinitions,
contracts: [contract],
defaultProvider: new ethers.providers.JsonRpcProvider("http://localhost:8545") //an example to override and connect to ganache
}
await SpaceMVC.init(spaceMvcInit)
Use Inversify to configure and inject dependencies into our services, DAOs, controllers, and more. You can also extend this behavior and bind your own objects to the container. You can also inject the services that SpaceMVC exports into your own classes.
SpaceMVC implements the Model-View-Controller pattern.
URL routes are mapped to controller objects with the @routeMap() annotation.
Services are where your business logic goes. A service is a POJO annotated with the @service() annotation.
Data Access Objects (DAOs) store and retreive data from OrbitDB and IPFS. A DAO is a POJO with a @dao() annotation.
SpaceMVC uses ethers.js under the hood for contract communication but we also use Truffle to develop Solidity unit test suites.
We have a basic interface that's agnostic to implementation but an easy way to import Truffle JSON files.
interface Contract {
name:string,
networks:Network[],
abi:any
}
interface Network {
networkId:string, //network ID is exposed by provider.send("net_version")
address:string //the address where the contract is deployed.
}
Example: This will grab all the networking information out of the Truffle JSON and into the right format.
import { ContractService } from "space-mvc"
let truffleJson = require('../truffle/build/contracts/YourContract.json')
let contract:Contract = await ContractService.createContractFromTruffle(truffleJson)
A store definition contains info to open an OrbitDB store when the application starts.
mfsstore is a key-value store for browser (or node) use and lazily loads records and allows multiple indexed columns
interface StoreDefinition {
name: string
type: string
load?: number
options: {
schema?: any
}
}
Example
let globalStoreDefinitions:StoreDefinition[] = [{
name: "player",
type: "mfsstore",
load: 100,
options: {
schema: {
id: { unique: true },
firstName: { unique: false },
lastName: { unique: false }
}
}
}]
A Metastore is an object that loads and connects to a group of OrbitDB stores. This allows you to define all of the stores that your application needs and open them all at once.
A Metastore named "WALLET_METASTORE" is created by default. It's only writeable by the user's wallet address. The app will open and load all of the stores and OrbitDB will sync all of the stores to all of the user's devices.
Creating a custom Metastore
// Open the schema associated with the wallet and cache it.
interface Metastore {
name:string
writeAccess?: string[]
storeDefinitions?:StoreDefinition[]
addresses?: any
stores?: any
}
let metastore = {
//Fill out name, writeAccess, and storeDefinitions from interface above.
}
await metastoreService.loadStoreAddresses(metastore)
await metastoreService.loadStores(metastore)
await metastoreService.cacheMetastore(metastore)
//To load a store later
await metastoreService.getStore("YOUR METASTORE NAME", "THE ORBITDB STORE NAME IN STORE DEFINITION")
//Load a store from the default wallet metastore
let store = metastoreService.getWalletStore("student")
Production:
npm run build
Development:
npm run build:dev
Tests:
npm run test
FAQs
TypeScript toolset to simplify building peer-to-peer applications using IPFS and Ethereum
The npm package space-mvc receives a total of 0 weekly downloads. As such, space-mvc popularity was classified as not popular.
We found that space-mvc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.