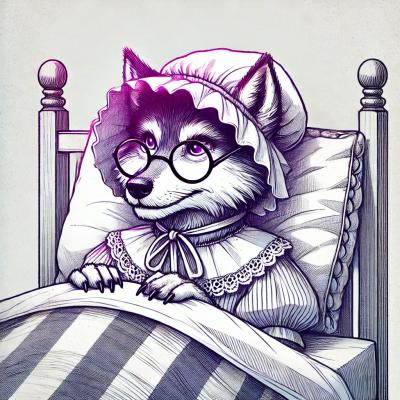
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
speed-measure-webpack-plugin
Advanced tools
Measure + analyse the speed of your webpack loaders and plugins
The speed-measure-webpack-plugin (SMP) is a tool designed to measure the build speed of your webpack configuration. It helps developers identify bottlenecks in their build process by providing detailed timing information for each plugin and loader used in the webpack configuration.
Measure Build Speed
This feature allows you to wrap your existing webpack configuration with SMP to measure the build speed. The `wrap` method is used to wrap the configuration, and SMP will then provide detailed timing information for each part of the build process.
const SpeedMeasurePlugin = require('speed-measure-webpack-plugin');
const smp = new SpeedMeasurePlugin();
module.exports = smp.wrap({
// Your webpack configuration
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: 'babel-loader'
}
]
},
plugins: [
// Your plugins
]
});
Detailed Timing Information
This feature allows you to customize the output format and target for the timing information. In this example, the `outputFormat` is set to 'human' for a more readable format, and the `outputTarget` is set to `console.log` to print the timing information to the console.
const SpeedMeasurePlugin = require('speed-measure-webpack-plugin');
const smp = new SpeedMeasurePlugin({
outputFormat: 'human',
outputTarget: console.log
});
module.exports = smp.wrap({
// Your webpack configuration
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: 'babel-loader'
}
]
},
plugins: [
// Your plugins
]
});
The webpack-bundle-analyzer plugin provides a visual representation of the size of webpack output files. It helps developers understand the size of their bundles and identify large dependencies. Unlike SMP, which focuses on build speed, webpack-bundle-analyzer focuses on bundle size and composition.
The webpack-dashboard plugin provides a terminal-based dashboard for webpack builds. It displays build progress, errors, and warnings in a more user-friendly way. While SMP focuses on measuring build speed, webpack-dashboard aims to improve the overall developer experience by providing real-time feedback during the build process.
The first step to optimising your webpack build speed, is to know where to focus your attention.
This plugin measures your webpack build speed, giving an output like this:
npm install --save speed-measure-webpack-plugin
or
yarn add speed-measure-webpack-plugin
Change your webpack config from
const webpackConfig = {
plugins: [
new MyPlugin(),
new MyOtherPlugin()
]
}
to
const SpeedMeasurePlugin = require("speed-measure-webpack-plugin");
const webpackConfig = {
plugins: SpeedMeasurePlugin.wrapPlugins({
MyPlugin: new MyPlugin(),
MyOtherPlugin: new MyOtherPlugin()
})
}
If you're using webpack-merge
, then you can do:
const smp = new SpeedMeasurePlugin();
const baseConfig = {
plugins: smp.wrapPlugins({
MyPlugin: new MyPlugin()
}).concat(smp)
// ^ note the `.concat(smp)`
};
const envSpecificConfig = {
plugins: smp.wrapPlugins({
MyOtherPlugin: new MyOtherPlugin()
})
// ^ note no `.concat(smp)`
}
const finalWebpackConfig = webpackMerge([
baseConfig,
envSpecificConfig
]);
Options are passed in to the constructor
const smp = new SpeedMeasurePlugin(options);
or as the second argument to the static wrapPlugins
SpeedMeasurePlugin.wrapPlugins(pluginMap, options);
options.outputFormat
Type: String
Default: "human"
Determines in what format this plugin prints its measurements
"json"
- produces a JSON blob"human"
- produces a human readable outputoptions.outputTarget
Type: String
Default: undefined
Specifies the path to a file to output to. If undefined, then output will print to console.log
options.disable
Type: Boolean
Default: false
If truthy, this plugin does nothing at all. It is recommended to set this with something similar to { disable: !process.env.MEASURE }
to allow opt-in measurements with a MEASURE=true npm run build
FAQs
Measure + analyse the speed of your webpack loaders and plugins
We found that speed-measure-webpack-plugin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.