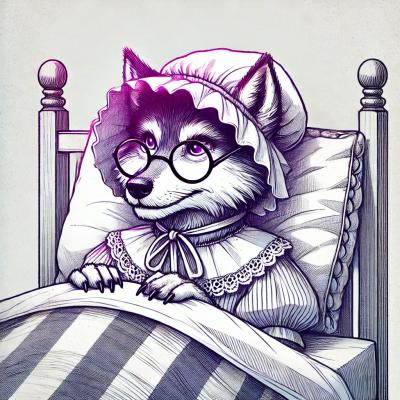
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The 'split' npm package is a stream utility that splits a stream of data into a stream of lines or any other delimited chunks. It's useful for processing streams of text data, such as logs or other outputs that are line-delimited.
Splitting stream by newlines
This code sample demonstrates how to use the 'split' package to read data from the standard input and split it into lines. Each line is then logged to the console.
"use strict";
const split = require('split');
process.stdin.pipe(split()).on('data', function (line) {
console.log('Line: ' + line);
});
Custom delimiter
This code sample shows how to use a custom delimiter (';') to split the stream into chunks. Each chunk is logged to the console.
"use strict";
const split = require('split');
process.stdin.pipe(split(';')).on('data', function (chunk) {
console.log('Chunk: ' + chunk);
});
Mapping lines
This code sample uses the 'split' package to map each line of the input to uppercase before emitting it. The mapped lines are logged to the console.
"use strict";
const split = require('split');
function map(line) {
return line.toUpperCase();
}
process.stdin.pipe(split(map)).on('data', function (line) {
console.log('Mapped Line: ' + line);
});
The 'byline' package is similar to 'split' in that it also provides a way to read a stream line by line. However, 'byline' is specifically focused on lines and does not support custom delimiters.
The 'binary-split' package is another alternative to 'split' that is optimized for splitting binary streams. It is similar in functionality but may perform better with binary data.
While 'through2' is not a direct alternative to 'split', it can be used in combination with other stream transformers to achieve similar line splitting functionality. It provides a simple wrapper around Node.js streams Transform to make creating custom transform streams easier.
Break up a stream and reassemble it so that each line is a chunk. matcher may be a String
, or a RegExp
Example, read every line in a file ...
fs.createReadStream(file)
.pipe(split())
.on('data', function (line) {
//each chunk now is a separate line!
})
split
takes the same arguments as string.split
except it defaults to '/\r?\n/' instead of ',', and the optional limit
parameter is ignored.
String#split
split
takes an optional options object on its third argument.
split(matcher, mapper, options)
Valid options:
matcher
,
if a single line exceeds this, the split stream will emit an error. split(JSON.parse, null, { maxLength: 2})
matcher
will be emitted. To prevent this set options.trailing
to false
. split(JSON.parse, null, { trailing: false })
As with String#split
, if you split by a regular expression with a matching group,
the matches will be retained in the collection.
stdin
.pipe(split(/(\r?\n)/))
... //lines + separators.
split
accepts a function which transforms each line.
fs.createReadStream(file)
.pipe(split(JSON.parse))
.on('data', function (obj) {
//each chunk now is a a js object
})
.on('error', function (err) {
//syntax errors will land here
//note, this ends the stream.
})
MIT
FAQs
split a Text Stream into a Line Stream
The npm package split receives a total of 5,123,921 weekly downloads. As such, split popularity was classified as popular.
We found that split demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.