spritesheet-templates 
Convert spritesheet data into CSS or CSS pre-processor data
spritesheet-templates
, formerly json2css
, was built as part of spritesmith
, a tool that converts images into spritesheets and CSS variables.
Getting Started
Install the module with: npm install spritesheet-templates
var templater = require('spritesheet-templates');
templater({
sprites: [{
name: 'github', x: 0, y: 0, width: 10, height: 20
}, {
name: 'twitter', x: 10, y: 20, width: 20, height: 30
}, {
name: 'rss', x: 30, y: 50, width: 50, height: 50
}],
spritesheet: {
width: 80, height: 100, image: 'url/path/to/spritesheet.png'
}
}, {format: 'stylus'});
Documentation
spritesheet-templates
is exports the funciton templater
as its module.exports
.
templater(data, options)
Converter for spritesheet/sprite info into spritesheet
- data
Object
- Container for data for template
- items
Object[]
- Deprecated alternative key to define data.sprites
- sprites
Object[]
- Array of objects with coordinate data about each sprite on the spritesheet
-
Object
- Container for sprite coordinate data
- For reference,
*
symbolizes any index (e.g. data.sprites[0]
) - name
String
- Name to use for the image - x
Number
- Horizontal coordinate of top-left corner of image - y
Number
- Vertical coordinate of top-left corner of image - width
Number
- Horizontal length of image in pixels - height
Number
- Vertical length of image in pixels
- spritesheet
Object
- Information about spritesheet
- width
Number
- Horizontal length of image in pixels - height
Number
- Vertical length of image in pixels - image
String
- URL to use for spritesheet
- This will typically be used in
background-image
- For example,
background-image: url({{spritesheet.image}});
- spritesheet_info
Object
- Optional container for metadata about spritesheet
and its representation
- name
String
- Prefix to use for all spritesheet variables
- For example,
icons
will generate $icons-width
/$icons-image
/etc in a Stylus template - By default, this is
spritesheet
(e.g. $spritesheet-width
, $spritesheet-image
)
- options
Object
- Optional settings
- spritesheetName
String
- Deprecated alternative for spritesheet_info.name
- format
String
- Format to generate output in
- We accept any format inside of the Templates section
- By default, we will use the
css
format
- formatOpts
Mixed
- Options to pass through to the formatter
Returns:
- retVal
String
- Result from specified formatter
Templates
Below are our template options for options.format
.
Handlebars-based templates support inheritance via handlebars-layouts
(e.g. {{#extend "css"}}
). Inherited templates must copy/paste JSON front matter. An example can be found in the Examples section.
css
Ouput CSS variables as CSS rules.
Options:
- cssSelector
Function
- Override mapping for CSS selector
cssSelector
should have signature function (sprite) { return 'selector'; }
- By default this will return
'.icon-' + sprite.name
- It will receive
sprite
with all parameters designed for template
Handlebars blocks:
css
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before CSS rules{{#content "sprites"}}
- CSS rules
Example:
.icon-sprite1 {
background-image: url(nested/dir/spritesheet.png);
background-position: 0px 0px;
width: 10px;
height: 20px;
}
.icon-sprite2 {
json
Output CSS variables in JSON format.
Example:
{
"sprite1": {
"x": 0,
"y": 0,
"width": 10,
"height": 20,
"total_width": 80,
"total_height": 100,
"image": "nested/dir/spritesheet.png",
"offset_x": 0,
"offset_y": 0,
"px": {
"x": "0px",
"y": "0px",
"offset_x": "0px",
"offset_y": "0px",
"height": "20px",
"width": "10px",
"total_height": "100px",
"total_width": "80px"
},
"escaped_image": "nested/dir/spritesheet.png"
},
"sprite2": {
json_array
Output CSS variables as an array of objects.
Example:
[
{
"name": "sprite1",
"x": 0,
"y": 0,
"width": 10,
"height": 20,
"total_width": 80,
"total_height": 100,
"image": "nested/dir/spritesheet.png",
"offset_x": 0,
"offset_y": 0,
"px": {
"x": "0px",
"y": "0px",
"offset_x": "0px",
"offset_y": "0px",
"height": "20px",
"width": "10px",
"total_height": "100px",
"total_width": "80px"
},
"escaped_image": "nested/dir/spritesheet.png"
},
{
"name": "sprite2",
less
Output CSS variables as LESS variables.
Options:
- functions
Boolean
- Flag to include mixins or not
- By default this is
true
(mixins will be included)
- variableNameTransforms
String[]
- Array of underscore.string
methods to run on variable names
Handlebars blocks:
less
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before LESS variable declarations{{#content "sprites"}}
- LESS variable declarations for sprites{{#content "spritesheet"}}
- LESS variable declarations for spritesheet{{#content "sprite-functions-comment"}}
- Comment before LESS functions for sprite variables{{#content "sprite-functions"}}
- LESS functions for sprite variables{{#content "spritesheet-functions-comment"}}
- Comment before LESS functions for spritesheet variables{{#content "spritesheet-functions"}}
- LESS functions for spritesheet variables
Example:
@sprite1-name: 'sprite1';
@sprite1-x: 0px;
@sprite1-y: 0px;
@sprite1-offset-x: 0px;
@sprite1-offset-y: 0px;
@sprite1-width: 10px;
@sprite1-height: 20px;
@sprite1-total-width: 80px;
@sprite1-total-height: 100px;
@sprite1-image: 'nested/dir/spritesheet.png';
@sprite1: 0px 0px 0px 0px 10px 20px 80px 100px 'nested/dir/spritesheet.png' 'sprite1';
@sprite2-name: 'sprite2';
sass
Output CSS variables as SASS variables.
Options:
- functions
Boolean
- Flag to include mixins or not
- By default this is
true
(mixins will be included)
- variableNameTransforms
String[]
- Array of underscore.string
methods to run on variable names
Handlebars blocks:
sass
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before SASS variable declarations{{#content "sprites"}}
- SASS variable declarations for sprites{{#content "spritesheet"}}
- SASS variable declarations for spritesheet{{#content "sprite-functions-comment"}}
- Comment before SASS functions for sprite variables{{#content "sprite-functions"}}
- SASS functions for sprite variables{{#content "spritesheet-functions-comment"}}
- Comment before SASS functions for spritesheet variables{{#content "spritesheet-functions"}}
- SASS functions for spritesheet variables
Example:
$sprite1-name: 'sprite1'
$sprite1-x: 0px
$sprite1-y: 0px
$sprite1-offset-x: 0px
$sprite1-offset-y: 0px
$sprite1-width: 10px
$sprite1-height: 20px
$sprite1-total-width: 80px
$sprite1-total-height: 100px
$sprite1-image: 'nested/dir/spritesheet.png'
$sprite1: 0px 0px 0px 0px 10px 20px 80px 100px 'nested/dir/spritesheet.png' 'sprite1'
$sprite2-name: 'sprite2'
// ...
scss
Output CSS variables as SCSS variables.
Options:
- functions
Boolean
- Flag to include mixins or not
- By default this is
true
(mixins will be included)
- variableNameTransforms
String[]
- Array of underscore.string
methods to run on variable names
Handlebars blocks:
scss
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before SCSS variable declarations{{#content "sprites"}}
- SCSS variable declarations for sprites{{#content "spritesheet"}}
- SCSS variable declarations for spritesheet{{#content "sprite-functions-comment"}}
- Comment before SCSS functions for sprite variables{{#content "sprite-functions"}}
- SCSS functions for sprite variables{{#content "spritesheet-functions-comment"}}
- Comment before SCSS functions for spritesheet variables{{#content "spritesheet-functions"}}
- SCSS functions for spritesheet variables
Example:
$sprite1-name: 'sprite1';
$sprite1-x: 0px;
$sprite1-y: 0px;
$sprite1-offset-x: 0px;
$sprite1-offset-y: 0px;
$sprite1-width: 10px;
$sprite1-height: 20px;
$sprite1-total-width: 80px;
$sprite1-total-height: 100px;
$sprite1-image: 'nested/dir/spritesheet.png';
$sprite1: 0px 0px 0px 0px 10px 20px 80px 100px 'nested/dir/spritesheet.png' 'sprite1';
$sprite2-name: 'sprite2';
scss_maps
Output CSS variables as SCSS maps variables.
Options:
- functions
Boolean
- Flag to include mixins or not
- By default this is
true
(mixins will be included)
- variableNameTransforms
String[]
- Array of underscore.string
methods to run on variable names
Handlebars blocks:
scss_maps
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before SCSS variable declarations{{#content "sprites"}}
- SCSS variable declarations for sprites{{#content "spritesheet"}}
- SCSS variable declaration for spritesheet{{#content "sprite-functions-comment"}}
- Comment before SCSS functions for sprite variables{{#content "sprite-functions"}}
- SCSS functions for sprite variables{{#content "spritesheet-functions-comment"}}
- Comment before SCSS functions for spritesheet variables{{#content "spritesheet-functions"}}
- SCSS functions for spritesheet variables
Example:
$sprite1: (
name: 'sprite1',
x: 0px,
y: 0px,
offset_x: 0px,
offset_y: 0px,
width: 10px,
height: 20px,
total_width: 80px,
total_height: 100px,
image: 'nested/dir/spritesheet.png'
);
$sprite2: (
// ...
stylus
Output CSS variables as Stylus variables.
Options:
- functions
Boolean
- Flag to include mixins or not
- By default this is
true
(mixins will be included)
- variableNameTransforms
String[]
- Array of underscore.string
methods to run on variable names
Handlebars blocks:
stylus
is a Handlebars based template. We allow for overriding the following sections:
{{#content "sprites-comment"}}
- Comment before Stylus variable declarations{{#content "sprites"}}
- Stylus variable declarations for sprites{{#content "spritesheet"}}
- Stylus variable declarations for spritesheet{{#content "sprite-functions-comment"}}
- Comment before Stylus functions for sprite variables{{#content "sprite-functions"}}
- Stylus functions for sprite variables{{#content "spritesheet-functions-comment"}}
- Comment before Stylus functions for spritesheet variables{{#content "spritesheet-functions"}}
- Stylus functions for spritesheet variables
Example:
$sprite1_name = 'sprite1';
$sprite1_x = 0px;
$sprite1_y = 0px;
$sprite1_offset_x = 0px;
$sprite1_offset_y = 0px;
$sprite1_width = 10px;
$sprite1_height = 20px;
$sprite1_total_width = 80px;
$sprite1_total_height = 100px;
$sprite1_image = 'nested/dir/spritesheet.png';
$sprite1 = 0px 0px 0px 0px 10px 20px 80px 100px 'nested/dir/spritesheet.png';
$sprite2_name = 'sprite2';
Custom
Custom templates can be added dynamically via templater.addTemplate
and templater.addHandlebarsTemplate
.
Template data
The parameters passed into your template are known as data
. These are a cloned copy of the data
originally passed in. We add some normalized properties for your convenience.
- data
Object
- Data available to template
- items
Object[]
- Deprecated alias for data.sprites
- sprites
Object[]
- Array of objects with coordinate data about each sprite on the spritesheet
-
Object
- Container for sprite coordinate data
- For reference,
*
symbolizes any index (e.g. data.sprites[0]
) - name
String
- Name to use for the image - x
Number
- Horizontal coordinate of top-left corner of image - y
Number
- Vertical coordinate of top-left corner of image - width
Number
- Horizontal length of image in pixels - height
Number
- Vertical length of image in pixels - total_width
Number
- Width of entire spritesheet - total_height
Number
- Height of entire spritesheet - image
String
- URL path to spritesheet - escaped_image
String
- URL encoded image
- offset_x
Number
- Negative value of x
. Useful to background-position
- offset_y
Number
- Negative value of y
. Useful to background-position
- px
Object
- Container for numeric values including px
- x
String
- x
suffixed with px
- y
String
- y
suffixed with px
- width
String
- width
suffixed with px
- height
String
- height
suffixed with px
- total_width
String
- total_width
suffixed with px
- total_height
String
- total_height
suffixed with px
- offset_x
String
- offset_x
suffixed with px
- offset_y
String
- offset_y
suffixed with px
- spritesheet
Object
- Information about spritesheet
- name
String
- Deprecated alias for spritesheet_info.name
- width
Number
- Horizontal length of image in pixels - height
Number
- Vertical length of image in pixels - image
String
- URL to use for spritesheet
- This will typically be used in
background-image
- For example,
background-image: url({{spritesheet.image}});
- escaped_image
String
- URL encoded image
- px
Object
container for numeric values including px
- width
String
- width
suffixed with px
- height
String
- height
suffixed with px
- spritesheet_name
String
- Deprecated alias for spritesheet_info.name
- spritesheet_info
Object
- Container for information about spritesheet
and its representation
- name
String
- Name for spritesheet
- options
Mixed
- Options to passed through via options.formatOpts
Handlebars template data
We provide an extra set of data for handlebars
templates for variable/string names.
- data.sprites[*].strings
Object
- Container for sprite-relevant variable/string names
- Each of these strings will be transformed via
variableNameTransforms
- name
String
- Transformed name of sprite (e.g. icon-home
) - name_name
String
- Transformed combination of sprite name and -name
string (e.g. icon-home-name
) - name_x
String
- Transformed combination of sprite name and -x
string (e.g. icon-home-x
) - name_y
String
- Transformed combination of sprite name and -y
string (e.g. icon-home-y
) - name_offset_x
String
- Transformed combination of sprite name and -offset-x
string (e.g. icon-home-offset-x
) - name_offset_y
String
- Transformed combination of sprite name and -offset-y
string (e.g. icon-home-offset-y
) - name_width
String
- Transformed combination of sprite name and -width
string (e.g. icon-home-width
) - name_height
String
- Transformed combination of sprite name and -height
string (e.g. icon-home-height
) - name_total_width
String
- Transformed combination of sprite name and -total-width
string (e.g. icon-home-total-width
) - name_total_height
String
- Transformed combination of sprite name and -total-height
string (e.g. icon-home-total-height
) - name_image
String
- Transformed combination of sprite name and -image
string (e.g. icon-home-image
) - name_sprites
String
- Transformed combination of sprite name and -sprites
string (e.g. icon-home-sprites
) - bare_name
String
- Transformed word for name
- bare_x
String
- Transformed word for x
- bare_y
String
- Transformed word for y
- bare_offset_x
String
- Transformed word for offset-x
- bare_offset_y
String
- Transformed word for offset-y
- bare_width
String
- Transformed word for width
- bare_height
String
- Transformed word for height
- bare_total_width
String
- Transformed word for total-width
- bare_total_height
String
- Transformed word for total-height
- bare_image
String
- Transformed word for image
- bare_sprites
String
- Transformed word for sprites
- data.spritesheet.strings
Object
- Deprecated container for spritesheet-relevant variable/string names
- Contents will match the same as
data.spritesheet_info.strings
- data.spritesheet_info.strings
Object
- Container for spritesheet-relevant variable/string names
- Each of these strings will be transformed via
variableNameTransforms
- name
String
- Transformed name of spritesheet (e.g. icon-home
) - name_name
String
- Transformed combination of spritesheet name and -name
string (e.g. icon-home-name
) - name_x
String
- Transformed combination of spritesheet name and -x
string (e.g. icon-home-x
) - name_y
String
- Transformed combination of spritesheet name and -y
string (e.g. icon-home-y
) - name_offset_x
String
- Transformed combination of spritesheet name and -offset-x
string (e.g. icon-home-offset-x
) - name_offset_y
String
- Transformed combination of spritesheet name and -offset-y
string (e.g. icon-home-offset-y
) - name_width
String
- Transformed combination of spritesheet name and -width
string (e.g. icon-home-width
) - name_height
String
- Transformed combination of spritesheet name and -height
string (e.g. icon-home-height
) - name_total_width
String
- Transformed combination of spritesheet name and -total-width
string (e.g. icon-home-total-width
) - name_total_height
String
- Transformed combination of spritesheet name and -total-height
string (e.g. icon-home-total-height
) - name_image
String
- Transformed combination of spritesheet name and -image
string (e.g. icon-home-image
) - name_sprites
String
- Transformed combination of spritesheet name and -sprites
string (e.g. icon-home-sprites
) - bare_name
String
- Transformed word for name
- bare_x
String
- Transformed word for x
- bare_y
String
- Transformed word for y
- bare_offset_x
String
- Transformed word for offset-x
- bare_offset_y
String
- Transformed word for offset-y
- bare_width
String
- Transformed word for width
- bare_height
String
- Transformed word for height
- bare_total_width
String
- Transformed word for total-width
- bare_total_height
String
- Transformed word for total-height
- bare_image
String
- Transformed word for image
- bare_sprites
String
- Transformed word for sprites
- data.strings
Object
- Container for generic strings
- Each of these strings will be transformed via
variableNameTransforms
- bare_name
String
- Transformed word for name
- bare_x
String
- Transformed word for x
- bare_y
String
- Transformed word for y
- bare_offset_x
String
- Transformed word for offset-x
- bare_offset_y
String
- Transformed word for offset-y
- bare_width
String
- Transformed word for width
- bare_height
String
- Transformed word for height
- bare_total_width
String
- Transformed word for total-width
- bare_total_height
String
- Transformed word for total-height
- bare_image
String
- Transformed word for image
- bare_sprites
String
- Transformed word for sprites
templater.addTemplate(name, fn)
Method to define a custom template under the format of name
.
- name
String
- Key to store template under for reference via options.format
- fn
Function
- Template function
- Should have signature of
function (data)
and return a String
output
templater.addHandlebarsTemplate(name, tmplStr)
Method to define a custom handlebars template under the format of name
.
As noted in the Templates section, these can inherit from existing templates via handlebars-layouts
conventions (e.g. {{#extend "scss"}}
). An example can be found in the Examples section.
- name
String
- Key to store template under for reference via options.format
- tmplStr
String
- Handlebars template to use for formatting
- This will receive
data
as its data
(e.g. {{sprites}}
is data.sprites
)
templater.addMustacheTemplate(name, tmplStr)
Deprecated alias for templater.addHandlebarsTemplate
Examples
Inheriting from a template
In this example, we will extend the SCSS template to output a minimal set of template data.
It should be noted that we must include the JSON front matter from the original template we are inheriting from to preserve default casing and options.
scss-minimal.handlebars:
{
// Default options
'functions': true,
'variableNameTransforms': ['dasherize']
}
{{#extend "scss"}}
{{#content "sprites"}}
{{#each sprites}}
${{strings.name}}: ({{px.x}}, {{px.y}}, {{px.offset_x}}, {{px.offset_y}}, {{px.width}}, {{px.height}}, {{px.total_width}}, {{px.total_height}}, '{{{escaped_image}}}', '{{name}}', );
{{/each}}
{{/content}}
{{#content "spritesheet"}}
${{spritesheet_info.strings.name_sprites}}: ({{#each sprites}}${{strings.name}}, {{/each}});
${{spritesheet_info.strings.name}}: ({{spritesheet.px.width}}, {{spritesheet.px.height}}, '{{{spritesheet.escaped_image}}}', ${{spritesheet_info.strings.name_sprites}}, );
{{/content}}
{{/extend}}
index.js:
var fs = require('fs');
var templater = require('spritesheet-templates');
var scssMinimalHandlebars = fs.readFileSync('scss-minimal.handlebars', 'utf8');
templater.addHandlebarsTemplate('scss-minimal', scssMinimalHandlebars);
templater({
sprites: [{
name: 'github', x: 0, y: 0, width: 10, height: 20
}, {
name: 'twitter', x: 10, y: 20, width: 20, height: 30
}, {
name: 'rss', x: 30, y: 50, width: 50, height: 50
}],
spritesheet: {
width: 80, height: 100, image: 'url/path/to/spritesheet.png'
}
}, {format: 'scss-minimal'});
Contributing
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint via npm run lint
and test via npm test
.
Donating
Support this project and others by twolfson via gratipay.
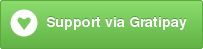
Unlicense
As of Sep 08 2013, Todd Wolfson has released this repository and its contents to the public domain.
It has been released under the UNLICENSE.
Prior to Sep 08 2013, this repository and its contents were licensed under the MIT license.