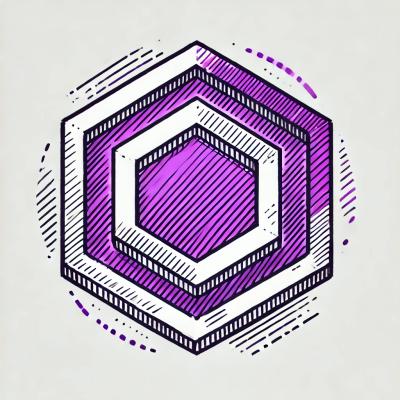
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
The sqlite3 npm package is a library that provides a straightforward interface for interacting with SQLite databases in Node.js applications. It allows you to create, read, update, and delete records in SQLite databases, execute SQL queries, and manage database connections.
Create a Database
This code demonstrates how to create an in-memory SQLite database, create a table, and insert some records into it.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
for (let i = 0; i < 10; i++) {
stmt.run('Ipsum ' + i);
}
stmt.finalize();
});
db.close();
Query a Database
This code shows how to create a table, insert records, and query the database to retrieve and print the records.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
for (let i = 0; i < 10; i++) {
stmt.run('Ipsum ' + i);
}
stmt.finalize();
db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {
console.log(row.id + ': ' + row.info);
});
});
db.close();
Update Records
This code demonstrates how to update records in an SQLite database.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
for (let i = 0; i < 10; i++) {
stmt.run('Ipsum ' + i);
}
stmt.finalize();
db.run('UPDATE lorem SET info = ? WHERE rowid = ?', ['Updated Ipsum', 1]);
db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {
console.log(row.id + ': ' + row.info);
});
});
db.close();
Delete Records
This code shows how to delete records from an SQLite database.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
for (let i = 0; i < 10; i++) {
stmt.run('Ipsum ' + i);
}
stmt.finalize();
db.run('DELETE FROM lorem WHERE rowid = ?', 1);
db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {
console.log(row.id + ': ' + row.info);
});
});
db.close();
better-sqlite3 is a faster and simpler alternative to sqlite3. It provides a more synchronous API, which can be easier to work with in some cases. Unlike sqlite3, better-sqlite3 does not use callbacks and instead returns results directly.
Sequelize is a promise-based Node.js ORM for various SQL databases, including SQLite. It provides a higher-level abstraction over SQL queries and supports features like model definition, associations, and migrations. It is more feature-rich compared to sqlite3 but also more complex.
Knex.js is a SQL query builder for Node.js that supports multiple databases, including SQLite. It provides a flexible and powerful API for building and executing SQL queries. Knex.js can be used with or without an ORM and offers more flexibility compared to sqlite3.
node-sqlite3 - Asynchronous, non-blocking SQLite3 bindings for node.js 0.2-0.4 (versions 2.0.x) and 0.6.x (versions 2.1.x).
Install with npm install sqlite3
.
var sqlite3 = require('sqlite3').verbose();
var db = new sqlite3.Database(':memory:');
db.serialize(function() {
db.run("CREATE TABLE lorem (info TEXT)");
var stmt = db.prepare("INSERT INTO lorem VALUES (?)");
for (var i = 0; i < 10; i++) {
stmt.run("Ipsum " + i);
}
stmt.finalize();
db.each("SELECT rowid AS id, info FROM lorem", function(err, row) {
console.log(row.id + ": " + row.info);
});
});
db.close();
See the API documentation in the wiki.
Make sure you have the sources for sqlite3
installed. Mac OS X ships with these by default. If you don't have them installed, install the -dev
package with your package manager, e.g. apt-get install libsqlite3-dev
for Debian/Ubuntu. Make sure that you have at least libsqlite3
>= 3.6.
To obtain and build the bindings:
git clone git://github.com/developmentseed/node-sqlite3.git
cd node-sqlite3
./configure
make
You can also use npm
to download and install them:
npm install sqlite3
expresso is required to run unit tests.
npm install expresso
make test
Thanks to Orlando Vazquez, Eric Fredricksen and Ryan Dahl for their SQLite bindings for node, and to mraleph on Freenode's #v8 for answering questions.
Development of this module is sponsored by Development Seed.
node-sqlite3
is BSD licensed.
FAQs
Asynchronous, non-blocking SQLite3 bindings
The npm package sqlite3 receives a total of 662,057 weekly downloads. As such, sqlite3 popularity was classified as popular.
We found that sqlite3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.