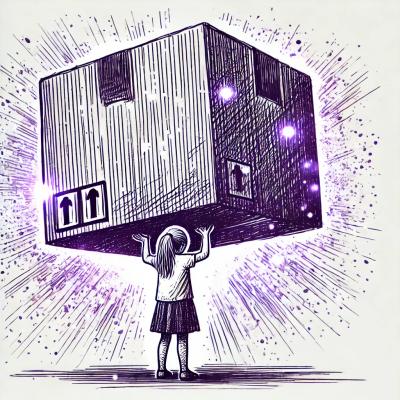
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
squeezer-chainkit
Advanced tools
Agnostic blockchain integration
The main usage of the ChainKit is to unify top blockchains interfaces into a single normalized API interface , therefore you can build blockchain apps easily without digging into blockchain infrastructure
Check the Squeezer ChainKit Gateway API Documentation:
npm install squeezer-chainkit --save
You will need a Squeezer access key in order to use the chainkit. You can get one here
const ChainKit = require('squeezer-chainkit');
const chainKit = new ChainKit(options);
options
requiredoptions.accessKey
- required Squeezer access keyoptions.environment
- required use test
for sandbox or live
for productionThe ITN system will notify your server when you receive a transaction and when a transaction status changes. This is a quick and useful way to integrate blockchain transactions processing.
Please check the Squeezer Blockchain Gateway API Documentation
-> Configure ITN callback url
chainKit.configureItnUrl(options, callback)
options
- required.options.url
- required. ITN callback url (http://example.org/itn/callback
).callback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.configureItnUrl({
url: 'http://example.org/itn/callback'
}, (err, response) => {
console.log(response)
});
Example response from server
{
"walletId": "8193d025-6430-496e-abf3-88f06b51889c",
"address": "0xbd61ef790C3eaf4D0c4D4bE3558F8a501863525f",
"token": "41dbecfb0454183a4c7a9be8b874e1785b5..."
}
ITN JSON object:
{
"from": "0xc03f7B9bddF8aeeBCbA2f818E5f873f71b85EB5c",
"to": "0x903f7B9bddF8aeeBCbA2f818E5f873f71b85EB5c",
"amount": "0.99999999",
"type": "in",
"currency": "ETH",
"accessKeyHash": "accessKeyHash",
"hash": "0xcf387e8d1a95bd3a5b54269aa0a228...",
"block": "891093",
"status": 1,
"itnStatus": 1,
"createdAt": "2018-05-13 18:09:18",
"updateAt": "2018-05-13 18:09:18"
}
NOTE: As a security measure please make sure that you validate accessKeyHash
is the same at with your default access key
Validate accessKeyHash
example:
if (accessKeyHash === crypto.createHmac('SHA256', accessKey).update(accessKey).digest('hex')) {
console.log('valid access key hash')
} else {
console.log('invalid access key hash')
}
Get current available blockchain wallet types
chainKit.walletTypes(callback)
callback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.walletTypes((err, response) => {
console.log(response)
});
Example response from server
{
"message":"success",
"data":[
{
"type":"ethereum",
"info":"Ethereum wallet."
},
{
"type":"stellar",
"info":"Stellar wallet."
}
]
}
Create a new blockchain wallet.
chainKit.createWallet(options, callback)
options
- required.options.type
- required. Wallet type (ETH
).options.secret
- required. Secret (secret123
).options.options
- required. Options object.callback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.createWallet({
type: 'ethereum',
options: {
asset: 'ETH',
assetType: 'native'
}
}, (err, response) => {
console.log(response)
});
Example response from server
{
"walletId": "8193d025-6430-496e-abf3-88f06b51889c",
"address": "0xbd61ef790C3eaf4D0c4D4bE3558F8a501863525f",
"token": "41dbecfb0454183a4c7a9be8b874e1785b5..."
}
NOTE: Squeezer will not store any sensitive data similar to token
. For later usage please store the wallet details on a secure & safe environment.
Initiate a new blockchain transaction.
chainKit.sendTransaction(options, callback)
options
- required.options.amount
- required. Amount to send, 8 decimal max. (0.01
).options.walltId
- required. wallet id. (ETH
).options.to
- required. Receiver's addressoptions.token
- required. Wallet tokenoptions.secret
- required. Secret (secret123
).callback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.sendTransaction({
amount: 0.01,
walletId: '1dd2e289-8f80-4b4a-8592-xxxxxxxxxxx',
to: '0x207E1a4F3Ab910D2164bC3646CFD0aF697f86713',
token: "41dbecfb04541........"
}, (err, response) => {
console.log(response)
});
Example response from server
{
"hash" : "0x4b9c1358fcbeb5434457355e3e8e44e10ebc6bec02d40c7a28046b1cfef99476"
}
Get transactions for a specific wallet
chainKit.getTransactions(options, callback)
options
- required.options.walletId
- required. Wallet IDcallback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.getTransactions({
walletId: "0dbeb851-b9e7-42e4-a448-71f8520f1ea3",
}, (err, response) => {
console.log(response)
});
Example response from server
{
"message": "success",
"data": [
{
"from": "0xc03f7B9bddF8aeeBCbA2f818E5f873f71b85EB5c",
"to": "0x903f7B9bddF8aeeBCbA2f818E5f873f71b85EB5c",
"amount": 0.99999999,
"type": "in",
"hash": "0xcf387e8d1a95bd3a5b54269aa0a228f159d3cd33fa9e946617c532c5cb8c77bb",
"block": 891093,
"status": 0,
"itnStatus": 0,
"createdAt": "2018-05-13 18:09:18",
"updateAt": "2018-05-13 18:09:18"
}
]
}
Get balance for a specific wallet
chainKit.getBalance(options, callback)
options
- required.options.walletId
- required. Wallet IDcallback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.getBalance({
walletId: "0dbeb851-b9e7-42e4-a448-71f8520f1ea3",
}, (err, response) => {
console.log(response)
});
Example response from server
{
"message":"success",
"data": {
"balance":0
}
}
Access a smart contract
chainKit.smartContract(options, callback)
options
- required.options.address
- required. Smart contract address.options.type
- required. Smart contract type. (ETH
)options.abi
- required. Abi code.options.methods
- required. Smart contract methods.options.token
- required. Wallet tokencallback
- required callback function, accepts 2 values (error
,result
)Example request
chainKit.smartContract({
type: 'ETH',
abi : '',
address: '0x207E1a4F3Ab910D2164bC3646CFD0aF697f86713',
token: '348nagfgf45tgtg....',
methods: [{
listVotes: ['arg1', 'arg2'],
votesType: ['positive']
}]
}, (err, response) => {
console.log(response)
});
FAQs
Squeezer agnostic blockchain integration
We found that squeezer-chainkit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.