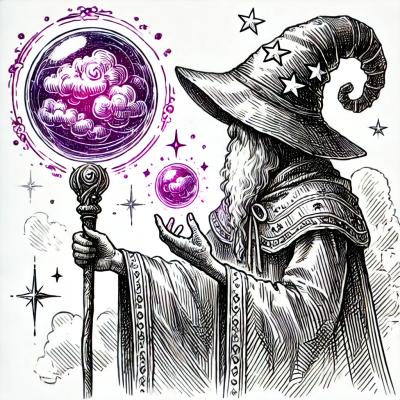
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Javascript implementation of the SSB binary field encodings spec.
The spec only has one type of nil, but JavaScript has two: null
and
undefined
. ssb-bfe will treat these two values in a way that mirrors what
JSON.stringify does:
null
field becomes an object with the
nil marker
JSON.stringify({a: null}) === '{"a": null}'
null
element becomes an array with the
nil marker
JSON.stringify([null]) === '[null]'
undefined
field will omit that field
JSON.stringify({a: undefined}) === '{}'
undefined
element becomes an array with
the nil marker
JSON.stringify([undefined]) === '[null]'
Takes any JavaScript primitive and returns its encoded counterpart. Is applied recursively in case the input is an object or an array. All inputs are converted to TFD Buffers, except for objects, arrays, and numbers, which remain the same.
Takes an encoded value (such as the output from encode
) and returns the
decoded counterparts as JavaScript primitives.
Returns the bfe.json
object that can be used to look up information
based on Type and Field. Example:
const { bfeTypes } = require('ssb-bfe')
const classic_key_size = bfeTypes[0][0].data_length
Returns the bfe.json
object converted to a map where the keys are
the type and format names. Example:
const { bfeNamedTypes } = require('ssb-bfe')
const FEED = bfeNamedTypes['feed']
const CLASSIC_FEED_TF = Buffer.from([FEED.code, FEED.formats['ssb/classic'].code])
FAQs
Binary Field Encodings (BFE) for Secure Scuttlebutt (SSB)
The npm package ssb-bfe receives a total of 92 weekly downloads. As such, ssb-bfe popularity was classified as not popular.
We found that ssb-bfe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 19 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.