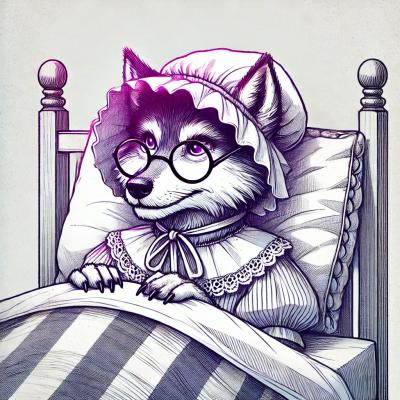
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
stack-chain
Advanced tools
The stack-chain npm package allows developers to customize and extend the stack traces in Node.js. It provides a way to manipulate the call stack, add custom frames, and format stack traces to make debugging easier.
Customizing Stack Traces
This feature allows you to customize the stack trace by modifying the frames. In this example, the file name in each frame is replaced with 'custom-file.js'.
const stackChain = require('stack-chain');
stackChain.extend.attach(function (error, frames) {
frames.forEach(frame => {
frame.getFileName = function () {
return 'custom-file.js';
};
});
return frames;
});
// Trigger an error to see the custom stack trace
try {
throw new Error('Test error');
} catch (error) {
console.log(error.stack);
}
Adding Custom Frames
This feature allows you to add custom frames to the stack trace. In this example, a custom frame with specific file name, line number, column number, and function name is added to the stack trace.
const stackChain = require('stack-chain');
stackChain.extend.attach(function (error, frames) {
frames.push({
getFileName: () => 'custom-frame.js',
getLineNumber: () => 42,
getColumnNumber: () => 21,
getFunctionName: () => 'customFunction'
});
return frames;
});
// Trigger an error to see the custom frame in the stack trace
try {
throw new Error('Test error');
} catch (error) {
console.log(error.stack);
}
Formatting Stack Traces
This feature allows you to format the stack trace according to your needs. In this example, the stack trace is formatted to show the function name, file name, line number, and column number in a custom format.
const stackChain = require('stack-chain');
stackChain.format.attach(function (error, frames) {
return frames.map(frame => {
return `${frame.getFunctionName()} at ${frame.getFileName()}:${frame.getLineNumber()}:${frame.getColumnNumber()}`;
}).join('\n');
});
// Trigger an error to see the formatted stack trace
try {
throw new Error('Test error');
} catch (error) {
console.log(error.stack);
}
The error-stack-parser package is used to parse and extract information from error stack traces. It provides a way to convert stack traces into a more readable and structured format. Unlike stack-chain, it does not allow customization or extension of the stack trace but focuses on parsing and extracting information.
The stacktrace-js package provides a way to capture and parse stack traces in JavaScript. It can be used in both Node.js and browser environments. While it offers similar functionality in terms of parsing stack traces, it does not provide the same level of customization and extension capabilities as stack-chain.
The trace package provides a way to trace function calls and log stack traces in Node.js. It offers features like logging function calls, arguments, and return values. While it provides some stack trace capabilities, its primary focus is on tracing function calls rather than customizing stack traces like stack-chain.
API for combining call site modifyers
npm install stack-chain
var chain = require('stack-chain');
When the Error.stack
getter is executed, the stack-chain
will perform the
following:
modifiers
attached by chain.extend
.modifiers
attached by chain.filter
.formater
set by chain.format.replace
.Will modify the callSite array. Note you shouldn't format the stack trace.
The modifier
is a function there takes two arguments error
and frames
.
error
is the Error
object.frames
is an array of callSite
objects, see
v8 documentation
for details.When the modifier
is done, it should return
a modified frames
array.
chain.filter.attach(function (error, frames) {
// Filter out traces related to this file
var rewrite = frames.filter(function (callSite) {
return callSite.getFileName() !== module.filename;
});
return rewrite;
});
Removes a modifier
function from the list of modifiers
.
var modifier = function () {};
// Attach modifier function
chain.extend.attach(modifier);
// Deattach modifier function
chain.extend.deattach(modifier);
Replaces the default v8 formater
. The new formater
takes a two arguments
error
and frames
.
error
is the Error
object.callSites
is an array of callSite
objects, see
v8 documentation
for details.When the formater
is done, it should return
a string
. The string
will
what Error.stack
returns.
chain.format.replace(function (error, frames) {
var lines = [];
lines.push(error.toString());
for (var i = 0; i < frames.length; i++) {
lines.push(" at " + frames[i].toString());
}
return lines.join("\n");
});
Will restore the default v8 formater
. Note that dude to the nature of v8
Error
objects, if one of the getters Error.stack
or Error.callSite
has
already executed, the value of Error.stack
won't change.
This will return the unmodified callSite
array from the current tick. This
is a performance shortcut, as it does not require generating the .stack
string. This behaviour is different from the Error().callSite
properties.
While this is mostly generating callSite
in hot code, it can be useful to
do some modification on the array. The options
object, supports the following:
options = {
// (default false) run the extenders on the callSite array.
extend: true,
// (default false) run the filters on the callSite array.
filter: true,
// (default 0) before running extend or filter methods, slice of some of the
// end. This can be useful for hiding the place from where you called this
// function.
slice: 2
}
This limites the size of the callSites
array. The default value is 10, and
can be set to any positive number including Infinity
. See
v8 documentation
for details.
Returns the original callSite
array.
Returns the mutated callSite
array, that is after extend
and filter
is applied. The array will not excite the Error.stackTraceLimit
.
The software is license under "MIT"
Copyright (c) 2012 Andreas Madsen
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
API for combining call site modifiers
The npm package stack-chain receives a total of 425,811 weekly downloads. As such, stack-chain popularity was classified as popular.
We found that stack-chain demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.