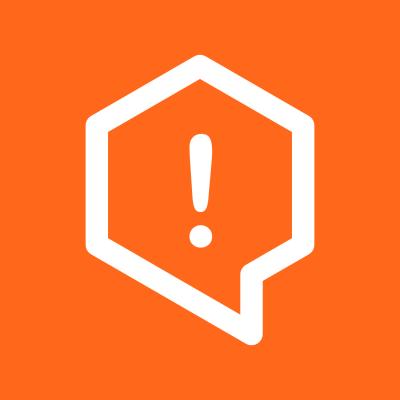
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
strict-event-emitter
Advanced tools
Type-safe implementation of EventEmitter for browser and Node.js
The 'strict-event-emitter' npm package is a TypeScript-first event emitter library that provides strong typing for event names and payloads. It ensures type safety and helps avoid common mistakes when working with events in TypeScript projects.
Basic Event Emission
This feature allows you to emit and listen to events with strong typing. The event name and payload are type-checked, ensuring that only valid events and payloads are used.
const { StrictEventEmitter } = require('strict-event-emitter');
interface Events {
greet: string;
}
const emitter = new StrictEventEmitter<Events>();
emitter.on('greet', (message) => {
console.log(message);
});
emitter.emit('greet', 'Hello, world!');
Typed Event Listeners
This feature allows you to define multiple events with different payload types. The event listeners are type-checked to ensure they handle the correct payload structure.
const { StrictEventEmitter } = require('strict-event-emitter');
interface Events {
greet: string;
farewell: { message: string; code: number };
}
const emitter = new StrictEventEmitter<Events>();
emitter.on('farewell', (data) => {
console.log(data.message, data.code);
});
emitter.emit('farewell', { message: 'Goodbye!', code: 200 });
Removing Event Listeners
This feature allows you to remove specific event listeners, ensuring that they no longer respond to emitted events. This is useful for cleaning up resources and avoiding memory leaks.
const { StrictEventEmitter } = require('strict-event-emitter');
interface Events {
greet: string;
}
const emitter = new StrictEventEmitter<Events>();
const greetListener = (message: string) => {
console.log(message);
};
emitter.on('greet', greetListener);
emitter.off('greet', greetListener);
emitter.emit('greet', 'Hello, world!'); // No output, listener removed
EventEmitter3 is a high-performance event emitter for Node.js and the browser. It is similar to strict-event-emitter but does not provide TypeScript-first strong typing for event names and payloads. It is more focused on performance and simplicity.
Mitt is a tiny (~200 bytes) functional event emitter. It is similar to strict-event-emitter in that it provides a simple API for emitting and listening to events, but it lacks the strong typing and TypeScript-first approach of strict-event-emitter.
Node Event Emitter is a lightweight event emitter library for Node.js. It provides basic event emission and listener functionality similar to strict-event-emitter but does not offer the same level of type safety and TypeScript integration.
A type-safe implementation of EventEmitter
for browser and Node.js.
Despite event emitters potentially accepting any runtime value, defining a strict event contract is crucial when developing complex event-driven architectures. Unfortunately, the native type definitions for Node's EventEmitter
annotate event names as string
, which forbids any stricter type validation.
// index.js
const emitter = new EventEmitter()
// Let's say our application expects a "ping"
// event with the number payload.
emitter.on('ping', (n: number) => {})
// We can, however, emit a different event by mistake.
emitter.emit('pong', 1)
// Or even the correct event with the wrong data.
emitter.emit('ping', 'wait, not a number')
The purpose of this library is to provide an EventEmitter
instance that can accept a generic describing the expected events contract.
import { Emitter } from 'strict-event-emitter'
// Define a strict events contract where keys
// represent event names and values represent
// the list of arguments expected in ".emit()".
type Events = {
ping: [number]
}
const emitter = new Emitter<Events>()
emitter.addListener('ping', (n) => {
// "n" argument type is inferred as "number'.
})
emitter.emit('ping', 10) // OK
emitter.emit('unknown', 10) // TypeError (invalid event name)
emitter.emit('ping', 'wait, not a number') // TypeError (invalid data)
This library is also a custom EventEmitter
implementation, which makes it compatible with other environments, like browsers or React Native.
npm install strict-event-emitter
import { Emitter } from 'strict-event-emitter'
// 1. Define an interface that describes your events.
// Set event names as the keys, and their expected payloads as values.
interface Events {
connect: [id: string]
disconnect: [id: string]
}
// 2. Create a strict emitter and pass the previously defined "Events"
// as its first generic argument.
const emitter = new Emitter<Events>()
// 3. Use the "emitter" the same way you'd use the regular "EventEmitter" instance.
emitter.addListener('connect', (id) => {})
emitter.emit('connect', 'abc-123')
MIT
FAQs
Type-safe implementation of EventEmitter for browser and Node.js
The npm package strict-event-emitter receives a total of 4,841,527 weekly downloads. As such, strict-event-emitter popularity was classified as popular.
We found that strict-event-emitter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.