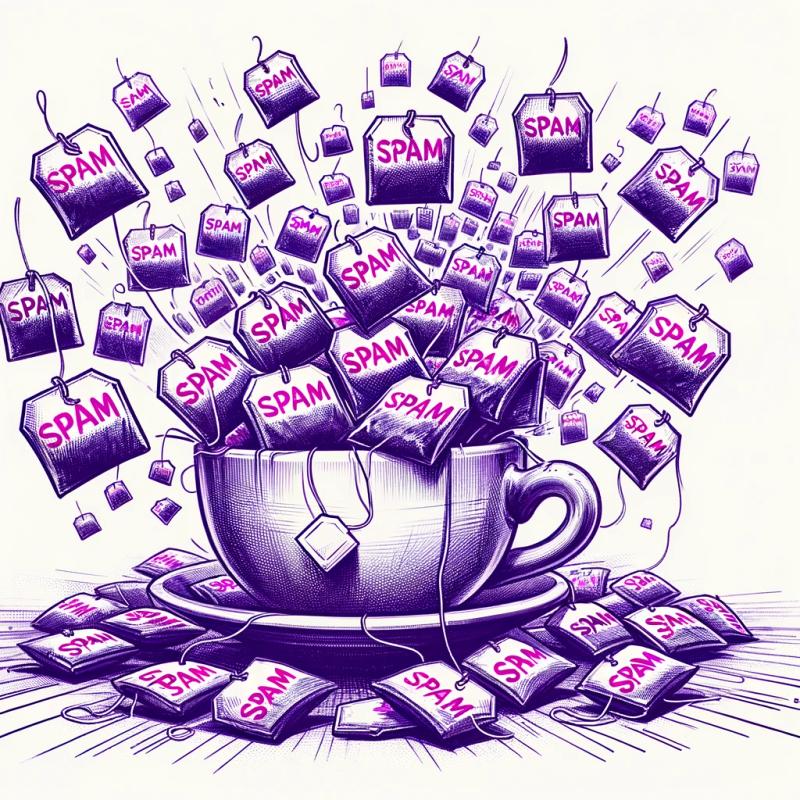
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
string-argv
Advanced tools
Package description
The string-argv npm package is used to parse string representations of command-line arguments into an array format, similar to how the arguments would be received in a Node.js script when running from the command line. It can handle quoted arguments, escaped characters, and supports both single and double quotes.
Parsing command-line argument strings
This feature allows you to convert a string that represents command-line arguments into an array of arguments, as they would appear in process.argv in a Node.js application.
const parseArgs = require('string-argv');
const args = parseArgs('node app.js --option=value "argument with spaces"');
console.log(args);
yargs-parser is a powerful argument parsing library that can parse command line arguments and generate an object. It supports various features like boolean flags, number parsing, arrays, and more. It is more feature-rich compared to string-argv, which focuses on converting a string to an argv array.
minimist is a minimalistic argument parsing library that also converts command line arguments to an object. It is simpler and has fewer features than yargs-parser but is similar to string-argv in its simplicity and direct approach to parsing arguments.
commander is a complete solution for node.js command-line interfaces, which is capable of parsing command line arguments and also includes a variety of other features like subcommands, custom help, auto-version, and more. It is more complex and feature-complete compared to string-argv, which is more focused on argument parsing.
Changelog
v0.2.0 (2019-04-14)
Parsing Behavior Changes
Readme
string-argv
parses a string into an argument array to mimic process.argv
.
This is useful when testing Command Line Utilities that you want to pass arguments to and is the opposite of what the other argv utilities do.
npm install string-argv --save
var stringArgv = require('string-argv');
var args = stringArgv(
'-testing test -valid=true --quotes "test quotes" "nested \'quotes\'" --key="some value" --title="Peter\'s Friends"',
'node',
'testing.js'
);
//legacy
var args2 = stringArgv.parseArgsStringToArgv(
'-testing test -valid=true --quotes "test quotes" "nested \'quotes\'" --key="some value" --title="Peter\'s Friends"',
'node',
'testing.js'
);
console.log(args);
/** output
[ 'node',
'testing.js',
'-testing',
'test',
'-valid=true',
'--quotes',
'test quotes',
'nested \'quotes\'',
'--key="some value"',
'--title="Peter\'s Friends"' ]
**/
required: arguments String: arguments that you would normally pass to the command line.
optional: environment String: Adds to the environment position in the argv array. If ommitted then there is no need to call argv.split(2) to remove the environment/file values. However if your cli.parse method expects a valid argv value then you should include this value.
optional: file String: file that called the arguments. If omitted then there is no need to call argv.split(2) to remove the environment/file values. However if your cli.parse method expects a valid argv value then you should include this value.
FAQs
string-argv parses a string into an argument array to mimic process.argv. This is useful when testing Command Line Utilities that you want to pass arguments to.
The npm package string-argv receives a total of 10,861,727 weekly downloads. As such, string-argv popularity was classified as popular.
We found that string-argv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.